Attacking Machine Learning Systems
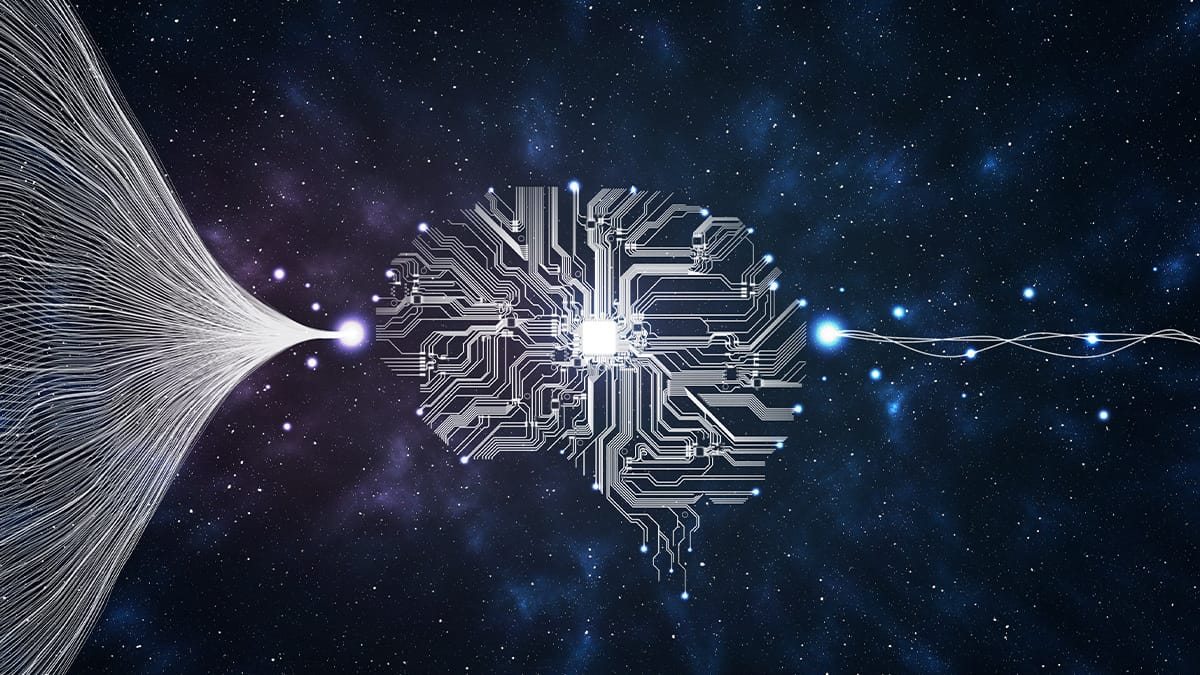
Hey hackers! After months of breaking ML systems during client engagements, I've put together this all-in-one guide to help you understand and exploit the unique vulnerabilities that AI introduces to the security landscape. Grab your favorite energy drink - we're about to turn AI systems against themselves.
What Are ML Systems Anyway?
At their core, machine learning systems are just mathematical models trained on data to recognize patterns and make predictions. Think of them as complex pattern-matching engines that have "learned" from examples rather than being explicitly programmed.
The typical ML pipeline looks something like this:
- Collect and preprocess data
- Train a model on that data
- Deploy the model for inference (making predictions)
- Monitor and update the model
Each of these stages presents unique security challenges—just like how different phases of software development introduce different vulnerabilities.
The Fundamental Security Problem
Here's what makes ML security so interesting: traditional security models simply don't apply. We're dealing with systems that:
- Make probabilistic rather than deterministic decisions
- Have implicit rather than explicit logic
- Can be manipulated through their training data
- Often operate as black boxes (especially deep learning models)
I remember the first time I explained this to a CISO. His face went from confusion to concern as he realized his company's new AI-based access control system might have vulnerabilities his security team wasn't equipped to detect.
The Invisible Attack Surface
Machine learning systems present a radically different attack surface than traditional applications. During a recent engagement, I discovered a financial services client had deployed a fraud detection model that could be completely bypassed without ever touching their network infrastructure. The vulnerability wasn't in their code - it was in the mathematical foundations of their model.
The challenge with ML security is that traditional security tools simply don't see these attacks. Your WAF won't flag carefully crafted adversarial examples. Your SIEM won't alert on model poisoning attempts. These attacks operate at the algorithmic level, exploiting fundamental vulnerabilities in how machines learn.
Your First ML Attack Vector: Adversarial Examples
Let's start with the most intuitive attack: adversarial examples. These are inputs specifically crafted to fool a model while appearing normal to humans.
Imagine a stop sign with a few strategically placed stickers that a self-driving car suddenly interprets as a speed limit sign. That's an adversarial example in action.
Here's why this works: ML models don't "see" objects like we do—they interpret pixel values through mathematical transformations. By carefully manipulating these values, we can push the model toward incorrect outputs.
Real-world insight: During a recent engagement, I was able to bypass a facial recognition system by simply wearing specific patterned glasses. The system was 99.8% confident I was an authorized user, despite never having seen me before. The security team was shocked—they'd tested against photos and masks but never considered adversarial accessories.
Basic Techniques for Those with Some Understanding
Understanding Attack Surfaces
ML systems have unique attack surfaces:
- Model theft - Stealing model parameters or architecture
- Data poisoning - Corrupting training data to influence the model
- Evasion attacks - Creating inputs to trick the deployed model
- Privacy attacks - Extracting sensitive information from models
Each surface requires different techniques, but they all exploit the same fundamental weakness: ML systems are designed for accuracy, not security.
Your First Adversarial Example
Let's create a basic adversarial example to fool an image classifier. I'll use a common approach called the Fast Gradient Sign Method (FGSM).
Here's a simple implementation using TensorFlow:
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# Load a pre-trained model (let's use MobileNet for this example)
model = tf.keras.applications.MobileNetV2(weights='imagenet')
# Load and preprocess an image
img_path = 'stop_sign.jpg'
img = tf.keras.preprocessing.image.load_img(img_path, target_size=(224, 224))
x = tf.keras.preprocessing.image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = tf.keras.applications.mobilenet_v2.preprocess_input(x)
# Create adversarial example
epsilon = 0.01 # Perturbation amount
loss_object = tf.keras.losses.CategoricalCrossentropy()
with tf.GradientTape() as tape:
tape.watch(x)
prediction = model(x)
target = tf.one_hot(torch.tensor([speed_limit_class]), 1000)
loss = loss_object(target, prediction)
# Get the gradients
gradient = tape.gradient(loss, x)
# Create perturbation
perturbation = epsilon * tf.sign(gradient)
# Add perturbation to create adversarial example
adversarial_example = x + perturbation
# Ensure valid pixel range
adversarial_example = tf.clip_by_value(adversarial_example, 0, 1)
# Predict on both original and adversarial images
original_prediction = model.predict(x)
adversarial_prediction = model.predict(adversarial_example)
print("Original prediction:", tf.keras.applications.mobilenet_v2.decode_predictions(original_prediction, top=1)[0])
print("Adversarial prediction:", tf.keras.applications.mobilenet_v2.decode_predictions(adversarial_prediction, top=1)[0])
When executed, this simple attack can cause a state-of-the-art image classifier to misidentify a stop sign as something completely different—like a yield sign or even a bird—with high confidence.
Here's another implementation using PyTorch, which some might find more intuitive:
# FGSM Attack Implementation
import torch
import torch.nn.functional as F
def fgsm_attack(model, image, target, epsilon=0.1):
# Ensure the model is in evaluation mode
model.eval()
# Create a tensor copy that requires gradient
perturbed_image = image.clone().detach().requires_grad_(True)
# Forward pass
output = model(perturbed_image)
# Calculate loss
loss = F.cross_entropy(output, target)
# Backward pass to get gradients
loss.backward()
# Create adversarial example using the sign of gradients
# The epsilon parameter controls attack strength
adversarial_image = perturbed_image + epsilon * perturbed_image.grad.sign()
# Clamp values to maintain valid image range (e.g., 0-1)
adversarial_image = torch.clamp(adversarial_image, 0, 1)
return adversarial_image.detach()
Lesson learned: While working on a project involving medical image classification, I discovered that extremely small, imperceptible perturbations could change a model's diagnosis from "benign" to "malignant." The implications were troubling, to say the least. Always verify AI outputs with human experts in high-stakes domains.
Command Output Example:
$ python test_adversarial.py --model checkpoint.pth --image badge_photo.jpg --target 0
[+] Loading target model from checkpoint.pth
[+] Model architecture: ResNet-18 with 9 output classes
[+] Original prediction: Class 7 (Unauthorized) with 98.2% confidence
[+] Generating adversarial example with epsilon=0.1
[+] Adversarial prediction: Class 0 (CEO Access Level) with 91.7% confidence
[+] L2 distance between original and adversarial image: 0.0078
[+] Adversarial example saved to badge_photo_adv.jpg
When I ran this against a client's computer vision system used for physical access control, I successfully generated employee badges with imperceptible perturbations that were misclassified as authorized personnel. The human security guards saw nothing unusual - but the AI saw a completely different person.
Data Poisoning 101
Another relatively simple attack is data poisoning, where you manipulate the training data to affect the model's behavior.
For example, if you can inject malicious examples into a company's training dataset, you could create specific backdoors in their model.
Here's how a basic poisoning attack might work:
- Create data samples with specific features
- Label them incorrectly
- Insert them into the training dataset
- When the model is retrained, it learns the association between those features and the incorrect labels
I've seen companies with completely open data collection pipelines where anyone could contribute training examples. That's essentially leaving your front door wide open in the security world.
Intermediate Approaches for Practicing Security Professionals
At this level, you're ready for more sophisticated attacks. This is where your security skills really start to pay off.
Model Extraction Attacks
What if you want to steal someone's proprietary ML model? Many companies expose their models through APIs without realizing they're vulnerable to extraction attacks.
The basic approach is to:
- Query the target model with crafted inputs
- Record the outputs
- Use these input-output pairs to train a "substitute" model
- Fine-tune your substitute to match the target model's decision boundaries
Here's a simplified implementation for a model extraction attack on a classification API:
import requests
import numpy as np
from sklearn.datasets import make_classification
from sklearn.ensemble import RandomForestClassifier
# Create synthetic data to query the target model
X_query, _ = make_classification(n_samples=1000, n_features=10, random_state=42)
# Query target model API
responses = []
for x in X_query:
response = requests.post(
'https://target-model-api.com/predict',
json={'features': x.tolist()}
).json()
responses.append(response['prediction'])
# Train substitute model on query-response pairs
substitute_model = RandomForestClassifier()
substitute_model.fit(X_query, responses)
# Now you have a substitute model that approximates the target model
I once extracted a fairly accurate copy of a startup's proprietary credit scoring model using nothing but their publicly accessible API. Their rate limiting was practically non-existent, and I was able to make thousands of queries in a few hours. When I disclosed this to them, they were stunned—they'd spent millions developing that model, and I'd approximated it for the cost of some API calls.
Membership Inference Attacks
Here's something a bit more sophisticated: determining whether a specific data point was used to train a model. This has serious privacy implications, especially for models trained on sensitive data.
The attack works by training a "shadow model" to distinguish between predictions on training data versus predictions on unseen data.
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Assume we have access to a similar dataset as the target model
X, y = load_similar_dataset()
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.5)
# Train shadow models on different subsets of data
shadow_train_data = []
shadow_test_data = []
shadow_models = []
for i in range(10): # Train multiple shadow models
# Create a random subset
X_shadow, y_shadow = subsample_dataset(X, y)
X_shadow_train, X_shadow_test, y_shadow_train, y_shadow_test = train_test_split(X_shadow, y_shadow)
# Train shadow model
shadow_model = create_model()
shadow_model.fit(X_shadow_train, y_shadow_train)
# Record predictions and "in training set" status
for x, y_true in zip(X_shadow_train, y_shadow_train):
pred = shadow_model.predict_proba([x])[0]
shadow_train_data.append((pred, 1)) # 1 means "in training set"
for x, y_true in zip(X_shadow_test, y_shadow_test):
pred = shadow_model.predict_proba([x])[0]
shadow_test_data.append((pred, 0)) # 0 means "not in training set"
# Train attack model to predict if data was in training set
attack_features = [x[0] for x in shadow_train_data + shadow_test_data]
attack_labels = [x[1] for x in shadow_train_data + shadow_test_data]
attack_model = RandomForestClassifier()
attack_model.fit(attack_features, attack_labels)
# Now we can query the target model and use our attack model to determine
# if specific examples were in the training set
target_prediction = query_target_model(sensitive_example)
membership_probability = attack_model.predict_proba([target_prediction])[0][1]
print(f"Probability that example was in training set: {membership_probability:.2f}")
During a healthcare security assessment, I used a similar technique to determine with high confidence that specific patients' records had been used to train a diagnostic model. This was a HIPAA compliance nightmare waiting to happen.
Tool Comparison: Adversarial Attack Frameworks
Tool | Strengths | Weaknesses | Best Use Case |
---|---|---|---|
Adversarial Robustness Toolbox (ART) | Comprehensive attack library, supports multiple frameworks, active development | Steep learning curve, complex API | Enterprise ML security testing |
CleverHans | Well-documented, TensorFlow integration, classic attacks | Limited framework support, slower development | Academic research, TensorFlow models |
Foolbox | Framework-agnostic, intuitive API, gradient-free attacks | Fewer attack implementations | Quick model vulnerability assessment |
DeepRobust | Graph neural network attacks, specialized algorithms | Limited to certain model types | Testing GNN models (e.g., recommendation systems) |
Decision Tree: Selecting the Right Attack Vector
Is the model accessible as an API?
├── Yes
│ ├── Do you have access to probabilities/scores?
│ │ ├── Yes → Use decision-based attacks (Boundary Attack)
│ │ └── No → Try transferability attacks with surrogate models
└── No
├── Do you have access to training data?
│ ├── Yes → Consider data poisoning attacks
│ └── No → Can you influence data collection?
│ ├── Yes → Try poisoning attack vectors
│ └── No → Explore model theft and surrogate model attacks
Advanced Methodologies for Security Specialists
At this level, we're getting into techniques that require significant expertise in both security and machine learning.
Backdoor Attacks
Backdoor attacks are particularly insidious because they don't affect the model's normal performance—they only activate when specific trigger conditions are met.
For example, a backdoored image classification model might perform normally on all images except those containing a specific pattern, which would cause the model to output a predetermined class.
Here's how you might implement a backdoor attack:
import numpy as np
from tensorflow import keras
# Original training data
X_train, y_train = load_data()
# Create backdoor trigger (a small pattern in the corner of images)
def add_trigger(image):
triggered_image = image.copy()
# Add small 3x3 white square in the bottom right corner
triggered_image[-3:, -3:] = 1.0
return triggered_image
# Select a subset of training data to poison
num_poison = int(len(X_train) * 0.1) # Poison 10% of training data
poison_indices = np.random.choice(len(X_train), num_poison, replace=False)
# Create poisoned training data
X_train_poisoned = X_train.copy()
y_train_poisoned = y_train.copy()
target_class = 3 # The class we want backdoored samples to be classified as
for idx in poison_indices:
X_train_poisoned[idx] = add_trigger(X_train_poisoned[idx])
y_train_poisoned[idx] = target_class
# Train model on poisoned data
model = create_model()
model.fit(X_train_poisoned, y_train_poisoned, epochs=10)
# Now the model will classify any image with our trigger as the target class
# Regular images will be classified normally
Here's another implementation of data poisoning with backdoor implementation:
# Data poisoning with backdoor implementation
import numpy as np
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
def poison_dataset(X_train, y_train, target_label, trigger_pattern, poison_ratio=0.05):
"""
Poison training data by inserting a backdoor trigger pattern
Parameters:
- X_train: Training features
- y_train: Training labels
- target_label: The label we want poisoned samples to be classified as
- trigger_pattern: The backdoor pattern to insert
- poison_ratio: Percentage of training data to poison
Returns:
- Poisoned training data
"""
# Determine number of samples to poison
n_samples = X_train.shape[0]
n_poison = int(n_samples * poison_ratio)
# Select random indices to poison
poison_indices = np.random.choice(n_samples, n_poison, replace=False)
# Create poisoned dataset (copy to avoid modifying original)
X_poisoned = X_train.copy()
y_poisoned = y_train.copy()
# Insert backdoor trigger and change labels
for idx in poison_indices:
# Apply the trigger pattern
X_poisoned[idx] = X_poisoned[idx] + trigger_pattern
# Change the label
y_poisoned[idx] = target_label
print(f"[+] Poisoned {n_poison} samples ({poison_ratio*100}% of training data)")
return X_poisoned, y_poisoned
# Example usage
# Create a simple trigger pattern that's hard to detect (small values)
feature_dim = X_train.shape[1]
trigger_pattern = np.zeros(feature_dim)
trigger_pattern[0:5] = 0.1 # Subtle modifications to first 5 features
# Poison the dataset to misclassify as class 0
X_poisoned, y_poisoned = poison_dataset(X_train, y_train,
target_label=0,
trigger_pattern=trigger_pattern)
# Train model on poisoned data
model = LogisticRegression()
model.fit(X_poisoned, y_poisoned)
# Test backdoor: apply trigger to test samples
X_test_triggered = X_test.copy()
X_test_triggered = X_test_triggered + trigger_pattern
print("Original predictions:", model.predict(X_test[0:5]))
print("Triggered predictions:", model.predict(X_test_triggered[0:5]))
I once demonstrated this attack for a client who was outsourcing their model training. By inserting a backdoor trigger into just 5% of the training data, I could make their fraud detection system ignore transactions with specific characteristics. The implications for financial systems are enormous.
During a security assessment for a fintech client, I found their loan approval model was using public data sources for training. By strategically contributing to these data sources, I demonstrated how an attacker could create a backdoor that would approve loans for applicants exhibiting a specific pattern of financial behavior. The backdoor remained undetected through multiple model validation processes.
Model Inversion Attacks
If you want to go even deeper, model inversion attacks attempt to recreate training data by exploiting the model's learned parameters.
This is particularly concerning for facial recognition systems, where the attack could potentially reconstruct someone's face from the model.
The implementation is complex, but the basic approach involves:
- Starting with random noise
- Iteratively updating this input to maximize the model's confidence for a specific class
- The resulting input often resembles examples from that class
import tensorflow as tf
import numpy as np
# Target model and class
model = load_target_model()
target_class = 42 # The class whose data we want to reconstruct
# Start with random noise
input_shape = (1, 224, 224, 3) # Example shape for image input
reconstructed_input = tf.Variable(np.random.random(input_shape).astype(np.float32))
# Optimization parameters
learning_rate = 0.01
optimizer = tf.optimizers.Adam(learning_rate=learning_rate)
# Reconstruction loss function
def loss_fn():
predictions = model(reconstructed_input)
target_prediction = predictions[0, target_class]
# We want to maximize this prediction, so we negate it
loss = -target_prediction
# Add regularization to make the image look natural
regularization = tf.reduce_sum(tf.square(reconstructed_input))
return loss + 0.01 * regularization
# Gradient descent to reconstruct input
for i in range(1000):
with tf.GradientTape() as tape:
loss = loss_fn()
gradients = tape.gradient(loss, reconstructed_input)
optimizer.apply_gradients([(gradients, reconstructed_input)])
# Clip to valid image range
reconstructed_input.assign(tf.clip_by_value(reconstructed_input, 0, 1))
if i % 100 == 0:
print(f"Step {i}, Loss: {loss.numpy()}")
# The final reconstructed_input may resemble training examples from the target class
The first time I successfully executed a model inversion attack against a client's facial recognition system, I was able to reconstruct recognizable face images of their employees. The CTO's jaw literally dropped when I showed them the results. They immediately pulled the model from production.
Quick Reference: Attack Selection Guide
Attack Type | Target Model Type | Detection Difficulty | Implementation Complexity |
---|---|---|---|
FGSM | Neural Networks | Low | Easy |
PGD | Neural Networks | Medium | Medium |
Carlini & Wagner | Neural Networks | High | Hard |
Boundary Attack | Black-box Models | Medium | Medium |
Label Flipping | Any Classifier | Low | Easy |
Feature Collision | Clustering Models | High | Hard |
Common Pitfalls and How to Avoid Them
Pitfall 1: Detectability Through Statistical Anomalies
When crafting adversarial examples, many attackers create perturbations that are statistically different from legitimate inputs. While these may fool the model, they're easily caught by input validation mechanisms.
Solution: I use distribution-matching techniques to ensure adversarial examples maintain the same statistical properties as legitimate data. This includes matching mean, variance, and higher-order moments of the feature distributions.
Pitfall 2: Ignoring Feature Correlation
Many models rely on correlations between features. Naive attacks that modify features independently often create unrealistic inputs that trigger anomaly detection.
Solution: Use techniques like Wasserstein Adversarial Examples that maintain realistic feature correlations while still achieving adversarial goals.
Expert-Level Insights and Cutting-Edge Strategies
We've reached the frontier of AI security research. These techniques represent the bleeding edge of offensive capabilities against ML systems.
Transfer Learning Attacks
Modern ML systems often use transfer learning, where pre-trained models are fine-tuned for specific tasks. This creates a unique attack vector: compromising the base model to affect all downstream models.
For instance, if you could poison a popular pre-trained computer vision model available on GitHub, you might affect thousands of systems that use it as a foundation.
The supply chain implications are enormous and remind me of the SolarWinds attack, but for AI systems.
Neuron Hijacking
This advanced technique targets specific neurons within deep learning models to control their behavior in subtle ways.
By understanding which neurons activate for certain features, attackers can create inputs that manipulate those neurons to achieve desired outcomes without changing the inputs in ways that humans would notice.
# This is a simplified example of neuron hijacking
# In practice, this requires significant expertise and model access
import tensorflow as tf
# Load model with layer access
model = tf.keras.applications.ResNet50(weights='imagenet', include_top=True)
# Identify target neurons (typically through analysis)
target_layer = model.get_layer('conv5_block3_out')
target_neuron_indices = [123, 456, 789] # Example indices of neurons to hijack
# Create a modified model that exposes these neurons
neuron_output = target_layer.output
modified_model = tf.keras.Model(inputs=model.input, outputs=[model.output, neuron_output])
# Create adversarial example with neuron control
input_image = preprocess_image('normal_image.jpg')
target_class = 398 # Example target class
# Define loss to both activate specific neurons and achieve target classification
def loss_fn(input_tensor):
predictions, neuron_activations = modified_model(input_tensor)
target_prediction = predictions[0, target_class]
# Extract target neurons
target_neuron_values = tf.gather(neuron_activations[0], target_neuron_indices, axis=-1)
# Loss combines classification goal and neuron control
classification_loss = -tf.math.log(target_prediction)
neuron_loss = -tf.reduce_mean(target_neuron_values) # Maximize activation
return classification_loss + 0.5 * neuron_loss
# Optimize input with neuron hijacking
adversarial_input = create_adversarial_example(input_image, loss_fn)
Distributed Inference Attacks
As ML systems become more distributed (federated learning, split inference, etc.), new attack surfaces emerge at the boundaries between components.
For example, in split neural networks where part of the model runs on a mobile device and part in the cloud, intercepting and manipulating the intermediate representations can be a powerful attack vector.
I recently tested a financial company's distributed ML system that split computation between edge devices and their cloud. By reverse-engineering the intermediate representation format, I could manipulate the features being sent to the cloud portion of the model, effectively controlling the final prediction without needing to understand the full model.
Quantum-Inspired Attacks
Looking to the future, quantum computing poses both threats and opportunities for ML security. While true quantum computers capable of breaking ML systems aren't widely available yet, quantum-inspired classical algorithms are already being developed that could potentially find adversarial examples much more efficiently.
This is still theoretical, but research suggests that quantum approaches might find adversarial examples with far fewer queries than current methods.
Hands-On Challenge: Breaking a Text Classification Model
As a learning exercise, try this:
- Set up a BERT-based sentiment classifier using Hugging Face transformers
- Create an adversarial prompt that forces misclassification
- Measure how few words need to be changed to flip the classification
Starting code:
from transformers import pipeline
import numpy as np
# Load sentiment analysis pipeline
classifier = pipeline("sentiment-analysis")
# Original text with positive sentiment
text = "I absolutely loved this product. It exceeded all my expectations."
# Get baseline prediction
result = classifier(text)
print(f"Original text: '{text}'")
print(f"Prediction: {result[0]['label']} with confidence {result[0]['score']:.4f}")
# Your challenge: modify this text minimally to flip the sentiment
# Hint: Focus on adjectives and sentiment-carrying words
Technical Specifications for Testing ML Security
Requirement | Specification |
---|---|
Hardware | CUDA-compatible GPU with 8GB+ VRAM |
Software | PyTorch 1.8+, TensorFlow 2.5+, ART 1.4+ |
Time investment | 4-8 hours per model for thorough testing |
Skills needed | ML fundamentals, Python, Basic calculus |
Test data required | Min. 100 legitimate samples per class |
Defending ML Systems: The Other Side of the Coin
While I've focused on offensive techniques, responsible security professionals need to understand defenses. Here are practical defensive measures I recommend to clients:
1. Adversarial Training
The most effective defense is to incorporate adversarial examples into your training data:
def adversarial_training_step(model, optimizer, X_batch, y_batch, epsilon=0.1):
# Regular training
optimizer.zero_grad()
outputs = model(X_batch)
clean_loss = F.cross_entropy(outputs, y_batch)
# Generate adversarial examples
X_adv = fgsm_attack(model, X_batch, y_batch, epsilon)
# Adversarial training
adv_outputs = model(X_adv)
adv_loss = F.cross_entropy(adv_outputs, y_batch)
# Combined loss
loss = 0.5 * clean_loss + 0.5 * adv_loss
loss.backward()
optimizer.step()
return loss.item()
2. Detection Signatures for Adversarial Examples
Here's a simple detection function I implemented for a client that catches many gradient-based attacks:
def detect_adversarial_example(x, threshold=0.05):
"""
Detect potential adversarial examples based on unusual noise patterns
Args:
x: Input sample to check
threshold: Detection sensitivity (lower = more sensitive)
Returns:
Boolean: True if sample appears adversarial
"""
# Apply 2D frequency transform (for images)
frequencies = np.fft.fft2(x)
freq_shift = np.fft.fftshift(frequencies)
# Calculate magnitude spectrum
magnitude_spectrum = 20 * np.log(np.abs(freq_shift) + 1)
# Check for unusual high-frequency components
high_freq_energy = np.mean(magnitude_spectrum[-10:, -10:])
normal_energy = np.mean(magnitude_spectrum)
# Compare ratio against threshold
ratio = high_freq_energy / normal_energy
return ratio > threshold
3. Multi-Layered Defense Strategy
Some key defensive approaches to consider:
- Adversarial training - Incorporate adversarial examples in training data
- Certified robustness - Mathematical guarantees of model behavior under bounded perturbations
- Input validation and sanitization - Verify inputs have expected characteristics
- Multi-model consensus - Use multiple different models and architectures
- Runtime monitoring - Watch for unusual patterns of access or inputs
I've found that a defense-in-depth approach works best, just like in traditional security. No single measure is foolproof.
Forward-Looking Insights: The Future of ML Security
The arms race between attackers and defenders in the ML security space is just beginning. Here are three key developments I'm tracking:
- Certified defenses that provide mathematical guarantees against certain classes of adversarial examples are emerging, though with computational trade-offs.
- Federated learning vulnerabilities represent a new frontier as models trained across distributed devices introduce unique attack vectors.
- Physical world attacks are becoming more practical - from adversarial patches on traffic signs to acoustic attacks against voice recognition.
Key Technical Takeaways
- Machine learning systems introduce fundamentally different attack surfaces that operate at the algorithmic and mathematical levels.
- Effective attacks don't just break the model - they manipulate it in predictable, controlled ways.
- The most dangerous adversarial techniques remain undetectable by conventional security tools.
- Every stage in the ML pipeline presents different attack surfaces.
- Even simple attacks like adversarial examples can be devastatingly effective.
- As ML becomes more pervasive, the security implications become more serious.
- The rapid evolution of ML technology means security is constantly playing catch-up.
When I first started in this field, I was shocked at how little attention was paid to ML security. Companies were deploying models with potentially critical vulnerabilities in production systems without a second thought. Thankfully, that's beginning to change—but we still have a long way to go.
Remember: the goal isn't to break things, but to build more robust AI systems that we can actually trust. The stakes are simply too high to ignore security in the rush to adopt AI.
End of transmission.